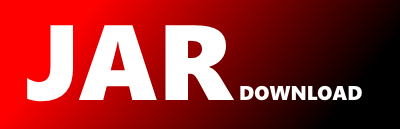
org.kawanfw.sql.transport.no_obfsucation.ArrayHttp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aceql-http Show documentation
Show all versions of aceql-http Show documentation
AceQL HTTP is a framework of REST like http APIs that allow to access to remote SQL databases over http from any device that supports http.
AceQL HTTP is provided with four client SDK:
- The AceQL C# Client SDK allows to wrap the HTTP APIs using Microsoft SQL Server like calls in their code, just like they would for a local database.
- The AceQL Java Client SDK allows to wrap the HTTP APIs using JDBC calls in their code, just like they would for a local database.
- The AceQL Python Client SDK allows SQL calls to be encoded with standard unmodified DB-API 2.0 syntax
/*
* Copyright (c)2022 KawanSoft S.A.S. All rights reserved.
*
* Use of this software is governed by the Business Source License included
* in the LICENSE.TXT file in the project's root directory.
*
* Change Date: 2026-11-01
*
* On the date above, in accordance with the Business Source License, use
* of this software will be governed by version 2.0 of the Apache License.
*/
package org.kawanfw.sql.transport.no_obfsucation;
import java.io.Serializable;
import java.sql.Array;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.Map;
import org.kawanfw.sql.util.Tag;
/**
* @author Nicolas de Pomereu
*
*/
class ArrayHttp implements Array, Serializable {
private static final String KAWANFW_NOT_SUPPORTED_METHOD = Tag.PRODUCT
+ "Method is not yet implemented.";
/**
* Generated serial version ID
*/
private static final long serialVersionUID = 7248103889999011521L;
// The ArrayId on host
private String arrayId = null;
private String baseTypeName = null;
private int baseType = -1;
private Object arrayElements;
public ArrayHttp(Array array) throws SQLException {
baseTypeName = array.getBaseTypeName();
baseType = array.getBaseType();
arrayElements = array.getArray();
}
public ArrayHttp(String arrayId, String baseTypeName, int baseType,
Object arrayElements) {
super();
this.arrayId = arrayId;
this.baseTypeName = baseTypeName;
this.baseType = baseType;
this.arrayElements = arrayElements;
}
/**
* @return the arrayId
*/
public String getArrayId() {
return arrayId;
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getBaseTypeName()
*/
@Override
public String getBaseTypeName() throws SQLException {
return baseTypeName;
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getBaseType()
*/
@Override
public int getBaseType() throws SQLException {
return baseType;
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getArray()
*/
@Override
public Object getArray() throws SQLException {
return arrayElements;
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getArray(java.util.Map)
*/
@Override
public Object getArray(Map> map) throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getArray(long, int)
*/
@Override
public Object getArray(long index, int count) throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getArray(long, int, java.util.Map)
*/
@Override
public Object getArray(long index, int count, Map> map)
throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getResultSet()
*/
@Override
public ResultSet getResultSet() throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getResultSet(java.util.Map)
*/
@Override
public ResultSet getResultSet(Map> map)
throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getResultSet(long, int)
*/
@Override
public ResultSet getResultSet(long index, int count) throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#getResultSet(long, int, java.util.Map)
*/
@Override
public ResultSet getResultSet(long index, int count,
Map> map) throws SQLException {
throw new SQLFeatureNotSupportedException(KAWANFW_NOT_SUPPORTED_METHOD);
}
/*
* (non-Javadoc)
*
* @see java.sql.Array#free()
*/
@Override
public void free() throws SQLException {
// Does nothing
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return "ArrayHttp [arrayId=" + arrayId + ", baseTypeName="
+ baseTypeName + ", baseType=" + baseType + ", arrayElements="
+ arrayElements + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy