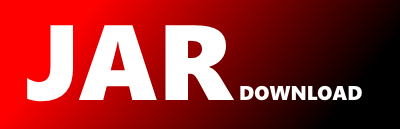
org.kawanfw.sql.jdbc.http.JdbcHttpFileTransfer Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.jdbc.http;
import java.io.File;
import java.sql.SQLException;
import java.util.logging.Level;
import org.kawanfw.commons.util.ClientLogger;
import org.kawanfw.commons.util.FrameworkDebug;
import org.kawanfw.commons.util.KeepTempFilePolicyParms;
import org.kawanfw.file.api.client.RemoteSession;
import org.kawanfw.sql.jdbc.ConnectionHttp;
/**
*
* Class that execute all http file transfer to the server.
*
*/
public class JdbcHttpFileTransfer {
/** Set to true to display/log debug info */
private static boolean DEBUG = FrameworkDebug
.isSet(JdbcHttpFileTransfer.class);
/** The Remote Connection */
private ConnectionHttp connectionHttp = null;
/**
* Constructor
*
* @param connectionHttp
* the http connection
*/
public JdbcHttpFileTransfer(ConnectionHttp connectionHttp) {
this.connectionHttp = connectionHttp;
}
/**
* Upload all the blob parameters without progress indicators
*/
public void uploadFile(File localFile, String remoteFileName)
throws SQLException {
if (localFile == null) {
throw new SQLException("localFile order is null!");
}
if (remoteFileName == null) {
throw new SQLException("remoteFileName order is null!");
}
if (!localFile.exists()) {
throw new SQLException("localFile to upload does not exists: "
+ localFile);
}
// Do the upload
try {
// fileSession.getFileTransferWrapper().upload(localFile,
// remoteFileName);
// FilesTransferWithProgress filesTransferWithProgress = new
// FilesTransferWithProgress(
// connectionHttp.getFileSession(),
// connectionHttp.getProgress(), connectionHttp.getCancelled());
// filesTransferWithProgress.upload(localFile, remoteFileName,
// localFile.length());
RemoteSession remoteSession = connectionHttp.getRemoteSession();
remoteSession.upload(localFile, remoteFileName);
} catch (Exception e) {
JdbcHttpTransferUtil.wrapExceptionAsSQLException(e);
} finally {
debug("local file: " + localFile);
debug("KeepTempFilePolicyParms.KEEP_TEMP_FILE: "
+ KeepTempFilePolicyParms.KEEP_TEMP_FILE);
if (!DEBUG && !KeepTempFilePolicyParms.KEEP_TEMP_FILE) {
localFile.delete();
}
}
}
/**
* Debug tool
*
* @param s
*/
// @SuppressWarnings("unused")
private void debug(String s) {
if (DEBUG) {
ClientLogger.getLogger().log(Level.WARNING, s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy