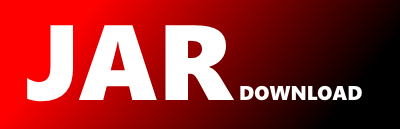
org.kawanfw.sql.jdbc.http.JdbcHttpTransferUtil Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.jdbc.http;
import java.io.IOException;
import java.net.ConnectException;
import java.net.MalformedURLException;
import java.net.SocketException;
import java.net.UnknownHostException;
import java.sql.SQLException;
import org.kawanfw.commons.api.client.InvalidLoginException;
import org.kawanfw.commons.api.client.RemoteException;
import org.kawanfw.sql.api.client.InvalidatedSessionException;
import org.kawanfw.sql.util.SqlReturnCode;
/**
* Utility methods for JdbcHttpTransfer
*
* @author Nicolas de Pomereu
*/
public class JdbcHttpTransferUtil {
/**
* Protected constructor, not callable
*/
protected JdbcHttpTransferUtil() {
}
/**
* Throws back the trapped Exception throw by HttpTransfer as SQLException
*
* @param e
* the Exception thrown by HttpTransfer call
*
* @throws SQLException
* the wrapped thrown Exception:
*/
public static void wrapExceptionAsSQLException(Exception e)
throws SQLException {
/*
* UnknownHostException, ConnectException, SocketException,
* RemoteException, SecurityException, IOException,
*/
if (e instanceof UnknownHostException) {
UnknownHostException wrappedException = (UnknownHostException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof ConnectException) {
ConnectException wrappedException = (ConnectException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof SocketException) {
SocketException wrappedException = (SocketException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof MalformedURLException) {
MalformedURLException wrappedException = (MalformedURLException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof InvalidLoginException) {
throw new SQLException(e);
}
// Must be done before IOException
else if (e instanceof RemoteException) {
RemoteException wrappedException = (RemoteException) e;
// Special case if message is SESSION_INVALIDATED
if (wrappedException.getMessage() != null
&& wrappedException.getMessage().trim()
.equals(SqlReturnCode.SESSION_INVALIDATED)) {
throw new SQLException(new InvalidatedSessionException());
} else {
throw new SQLException(wrappedException.getMessage(),
wrappedException);
}
}
else if (e instanceof SecurityException) {
SecurityException wrappedException = (SecurityException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
// SecurityException wrappedException = (SecurityException) e;
// throw wrappedException;
}
else if (e instanceof IOException) // IOException thrown by local code
{
IOException wrappedException = (IOException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else if (e instanceof InterruptedException) // InterruptedException
// thrown by local code
{
InterruptedException wrappedException = (InterruptedException) e;
throw new SQLException(wrappedException.getMessage(),
wrappedException);
} else {
// Other Exceptions...
IOException wrappedException = new IOException(e);
throw new SQLException(wrappedException.getMessage(),
wrappedException);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy