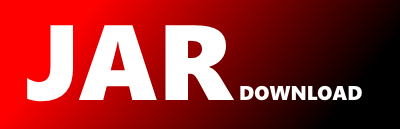
org.kawanfw.sql.jdbc.util.StatementHolderFileList Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.jdbc.util;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Writer;
import java.sql.SQLException;
import java.util.Date;
import java.util.List;
import java.util.Vector;
import org.apache.commons.io.IOUtils;
import org.kawanfw.commons.util.FrameworkDebug;
import org.kawanfw.commons.util.FrameworkFileUtil;
import org.kawanfw.commons.util.KeepTempFilePolicyParms;
import org.kawanfw.commons.util.Tag;
import org.kawanfw.sql.jdbc.ConnectionHttp;
import org.kawanfw.sql.json.StatementHolder;
import org.kawanfw.sql.json.StatementHolderTransport;
/**
* @author Nicolas de Pomereu
*
* File holder for StatementHolder added one per one at end of writer
*
*/
public class StatementHolderFileList {
/** Debug flag */
private static boolean DEBUG = FrameworkDebug
.isSet(StatementHolderFileList.class);
public static String CR_LF = System.getProperty("line.separator");
/** The local file name created */
private File localFile = null;
/** The remote file name to create */
private String remoteFileName = null;
/** The write to use */
private Writer writer = null;
/** The actual size of the file list (number of lines) **/
private int size = 0;
/** the maximum allowed statements for transport in memory */
private int maxStatementsForMemoryTransport = 0;
/** For debug usage */
private boolean isFirstAdd = true;
/**
* Keep in memory all statements holder up to
* maxStatementsForMemoryTransport
*/
private List statementHolderList = new Vector();
/** The connection in use */
private ConnectionHttp connectionHttp = null;
/**
* Constructor
*
* @param maxStatementsForMemoryTransport
* the maximum allowed statements for transport in memory
*/
public StatementHolderFileList(ConnectionHttp connectionHttp) {
if (connectionHttp == null) {
throw new IllegalArgumentException(Tag.PRODUCT_PRODUCT_FAIL
+ "connectionHttp can not be null!");
}
// Create the file names
String unique = FrameworkFileUtil.getUniqueId();
String dir = FrameworkFileUtil.getKawansoftTempDir();
remoteFileName = "stat-holder-list-" + unique + ".kawanfw";
localFile = new File(dir + File.separator + remoteFileName);
this.maxStatementsForMemoryTransport = connectionHttp
.getMaxStatementsForMemoryTransport();
this.connectionHttp = connectionHttp;
}
/**
* Json a StatementHolder and add it to the end of the file, and
*
* @param statementHolder
* the statement holder to add
*/
public void add(StatementHolder statementHolder) throws SQLException {
if (DEBUG && isFirstAdd) {
System.out.println(new Date()
+ " StatementHolderFileList: Fist add...");
// isFirstAdd = false;
}
try {
if (writer == null) {
writer = new BufferedWriter(new FileWriter(localFile));
}
} catch (IOException e) {
throw new SQLException(Tag.PRODUCT_PRODUCT_FAIL
+ "Impossible to create the writer for localFile: "
+ localFile, e);
}
// Encrypt here
StatementHolderListEncryptor.encrypt(statementHolder,
this.connectionHttp);
String jsonString = StatementHolderTransport.toJson(statementHolder);
if (DEBUG && isFirstAdd) {
System.out.println(new Date()
+ " StatementHolderFileList: jsonString: " + jsonString);
isFirstAdd = false;
}
try {
writer.write(jsonString + CR_LF);
} catch (IOException e) {
throw new SQLException(Tag.PRODUCT_PRODUCT_FAIL
+ "Impossible to write on localFile : " + localFile, e);
}
size++;
// Store in memory the first maxStatementsForMemoryTransport
// StatementHolder
if (size <= maxStatementsForMemoryTransport) {
statementHolderList.add(statementHolder);
}
}
/**
* @return the localFile
*/
public File getLocalFile() {
return this.localFile;
}
/**
* Delete the associated file
*
* @return true if the file ise deleted
*/
public boolean delete() {
this.close();
if (KeepTempFilePolicyParms.KEEP_TEMP_FILE || DEBUG) {
return false;
} else {
return this.localFile.delete();
}
}
/**
* @return the remoteFileName
*/
public String getRemoteFileName() {
return this.remoteFileName;
}
/** Returns the size of the file list */
public int size() {
return size;
}
public boolean isEmpty() {
return size() == 0;
}
/**
* @return the statementHolderList
*/
public List getStatementHolderList() {
return this.statementHolderList;
}
/**
* closes the underlying writer
*/
public void close() {
if (DEBUG) {
System.out.println(new Date() + " StatementHolderFileList: Close!");
}
IOUtils.closeQuietly(writer);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy