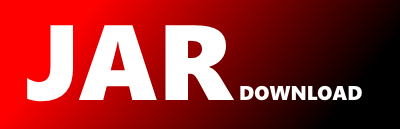
org.kawanfw.sql.json.no_obfuscation.CallableStatementHolderTransportJsonSimple Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.json.no_obfuscation;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.Vector;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.JSONValue;
import org.kawanfw.commons.util.FrameworkDebug;
import org.kawanfw.sql.jsontypes.StaParms;
public class CallableStatementHolderTransportJsonSimple {
/** Debug flag */
private static boolean DEBUG = FrameworkDebug
.isSet(CallableStatementHolderTransportJsonSimple.class);
/**
* Convert to Json a unique of StatementHolder
*
* @return the instance converted to Json
*/
public static String toJson(CallableStatementHolder statementHolder) {
int[] stP = statementHolder.getStP();
List list = new Vector();
for (int i = 0; i < stP.length; i++) {
list.add(stP[i]);
}
String stPstr = JSONValue.toJSONString(list);
String parmsTstr = JSONValue.toJSONString(statementHolder.getParmsT());
String parmsVstr = JSONValue.toJSONString(statementHolder.getParmsV());
String outPstr = JSONValue.toJSONString(statementHolder.getOutP());
// Put all in map
Map map = new HashMap();
map.put("sql", statementHolder.getSql());
map.put("stP", stPstr);
map.put("parmsT", parmsTstr);
map.put("parmsV", parmsVstr);
map.put("outP", outPstr);
map.put("paramatersEncrypted", statementHolder.isParamatersEncrypted());
map.put("htmlEncodingOn", statementHolder.isHtmlEncodingOn());
String theString = JSONValue.toJSONString(map);
// Clean all to release memory for GC
stP = null;
statementHolder = null;
map = null;
return theString;
}
/**
* Convert from a Json string a List of StatementHolder
*
* @return the StatementHolder list converted from Json
*/
@SuppressWarnings({ "rawtypes" })
public static CallableStatementHolder fromJson(String jsonString) {
// Revert it
Object obj = JSONValue.parse(jsonString);
JSONObject mapBack = (JSONObject) obj;
String sql = (String) mapBack.get("sql");
String stPstr = (String) mapBack.get("stP");
String parmsTstr = (String) mapBack.get("parmsT");
String parmsVstr = (String) mapBack.get("parmsV");
String outPstr = (String) mapBack.get("outP");
Boolean paramatersEncrypted = (Boolean) mapBack
.get("paramatersEncrypted");
Boolean htmlEncodingOn = (Boolean) mapBack.get("htmlEncodingOn");
mapBack = null;
Object objParmsT = JSONValue.parse(parmsTstr);
JSONObject mapBackParmsT = (JSONObject) objParmsT;
Object objParmsV = JSONValue.parse(parmsVstr);
JSONObject mapBackParmsV = (JSONObject) objParmsV;
Object JSONArray = JSONValue.parse(stPstr);
JSONArray arrayBackStP = (JSONArray) JSONArray;
Object outPstrT = JSONValue.parse(outPstr);
JSONArray arrayBackoutP = (JSONArray) outPstrT;
int[] stP = new int[StaParms.NUM_PARMS];
for (int i = 0; i < arrayBackStP.size(); i++) {
long myLong = (Long) arrayBackStP.get(i);
stP[i] = (int) myLong;
}
Map parmsT = new TreeMap();
Set set = mapBackParmsT.keySet();
for (Iterator iterator = set.iterator(); iterator.hasNext();) {
String key = (String) iterator.next();
long value = (Long) mapBackParmsT.get(key);
parmsT.put(Integer.parseInt(key), (int) value);
}
Map parmsV = new TreeMap();
set = mapBackParmsV.keySet();
for (Iterator iterator = set.iterator(); iterator.hasNext();) {
String key = (String) iterator.next();
String value = (String) mapBackParmsV.get(key);
parmsV.put(Integer.parseInt(key), value);
}
List outP = new ArrayList();
for (int i = 0; i < arrayBackoutP.size(); i++) {
long myLong = (Long) arrayBackoutP.get(i);
outP.add((int) myLong);
}
// Clean all to release memory for GC
obj = null;
objParmsT = null;
objParmsV = null;
arrayBackStP = null;
arrayBackoutP = null;
if (DEBUG) {
String stPaString = "[";
for (int i = 0; i < stP.length; i++) {
stPaString += stP[i] + " ";
}
stPaString += "]";
System.out.println();
System.out.println(sql);
System.out.println(stPaString);
System.out.println(parmsT);
System.out.println(parmsV);
System.out.println(outP);
System.out.println(paramatersEncrypted);
System.out.println(htmlEncodingOn);
System.out.println();
}
CallableStatementHolder callableStatementHolder = new CallableStatementHolder();
callableStatementHolder.setSql(sql);
callableStatementHolder.setStP(stP);
callableStatementHolder.setParmsT(parmsT);
callableStatementHolder.setParmsV(parmsV);
callableStatementHolder.setOutP(outP);
callableStatementHolder.setParamatersEncrypted(paramatersEncrypted);
callableStatementHolder.setHtmlEncodingOn(htmlEncodingOn);
return callableStatementHolder;
}
/**
* Debug
*
* @param s
*/
public static void debug(String s) {
if (DEBUG) {
System.out.println(s);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy