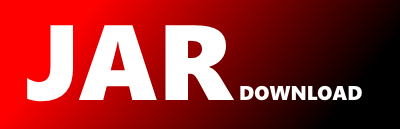
org.kawanfw.sql.json.no_obfuscation.ResultSetMetaDataHolder Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.json.no_obfuscation;
import java.io.Serializable;
import java.util.List;
import java.util.Vector;
import org.kawanfw.commons.util.HtmlConverter;
/**
* @author Nicolas de Pomereu A holder for a ResultSetMetaData that we want to
* transport.
*
*/
public class ResultSetMetaDataHolder implements Serializable {
/**
* Cleaner
*/
private static final long serialVersionUID = 1798593349080529806L;
private int columnCount;
private List autoIncrement;
private List caseSensitive;
private List searchable;
private List currency;
private List nullable;
private List signed;
private List columnDisplaySize;
private List columnLabel;
private List columnName;
private List schemaName;
private List precision;
private List scale;
private List tableName;
private List catalogName;
private List columnType;
private List columnTypeName;
private List readOnly;
private List writable;
private List definitelyWritable;
private List columnClassName;
/**
* @return the autoIncrement
*/
public List getAutoIncrement() {
return this.autoIncrement;
}
/**
* @param autoIncrement
* the autoIncrement to set
*/
public void setAutoIncrement(List autoIncrement) {
this.autoIncrement = autoIncrement;
}
/**
* @return the caseSensitive
*/
public List getCaseSensitive() {
return this.caseSensitive;
}
/**
* @param caseSensitive
* the caseSensitive to set
*/
public void setCaseSensitive(List caseSensitive) {
this.caseSensitive = caseSensitive;
}
/**
* @return the searchable
*/
public List getSearchable() {
return this.searchable;
}
/**
* @param searchable
* the searchable to set
*/
public void setSearchable(List searchable) {
this.searchable = searchable;
}
/**
* @return the currency
*/
public List getCurrency() {
return this.currency;
}
/**
* @param currency
* the currency to set
*/
public void setCurrency(List currency) {
this.currency = currency;
}
/**
* @return the nullable
*/
public List getNullable() {
return this.nullable;
}
/**
* @param nullable
* the nullable to set
*/
public void setNullable(List nullable) {
this.nullable = nullable;
}
/**
* @return the signed
*/
public List getSigned() {
return this.signed;
}
/**
* @param signed
* the signed to set
*/
public void setSigned(List signed) {
this.signed = signed;
}
/**
* @return the columnDisplaySize
*/
public List getColumnDisplaySize() {
return this.columnDisplaySize;
}
/**
* @param columnDisplaySize
* the columnDisplaySize to set
*/
public void setColumnDisplaySize(List columnDisplaySize) {
this.columnDisplaySize = columnDisplaySize;
}
/**
* @return the columnLabel
*/
public List getColumnLabel() {
// return this.columnLabel;
List columnLabelReturn = new Vector();
for (String label : this.columnLabel) {
columnLabelReturn.add(HtmlConverter.fromHtml(label));
}
return columnLabelReturn;
}
/**
* @param columnLabel
* the columnLabel to set
*/
public void setColumnLabel(List columnLabel) {
this.columnLabel = new Vector();
for (String label : columnLabel) {
this.columnLabel.add(HtmlConverter.toHtml(label));
}
}
/**
* @return the columnName
*/
public List getColumnName() {;
List columnNameReturn = new Vector();
for (String name : this.columnName) {
columnNameReturn.add(HtmlConverter.fromHtml(name));
}
return columnNameReturn;
}
/**
* @param columnName
* the columnName to set
*/
public void setColumnName(List columnName) {
this.columnName = new Vector();
for (String name : columnName) {
this.columnName.add(HtmlConverter.toHtml(name));
}
}
/**
* @return the schemaName
*/
public List getSchemaName() {
return this.schemaName;
}
/**
* @param schemaName
* the schemaName to set
*/
public void setSchemaName(List schemaName) {
this.schemaName = schemaName;
}
/**
* @return the precision
*/
public List getPrecision() {
return this.precision;
}
/**
* @param precision
* the precision to set
*/
public void setPrecision(List precision) {
this.precision = precision;
}
/**
* @return the scale
*/
public List getScale() {
return this.scale;
}
/**
* @param scale
* the scale to set
*/
public void setScale(List scale) {
this.scale = scale;
}
/**
* @return the tableName
*/
public List getTableName() {
return this.tableName;
}
/**
* @param tableName
* the tableName to set
*/
public void setTableName(List tableName) {
this.tableName = tableName;
}
/**
* @return the catalogName
*/
public List getCatalogName() {
return this.catalogName;
}
/**
* @param catalogName
* the catalogName to set
*/
public void setCatalogName(List catalogName) {
this.catalogName = catalogName;
}
/**
* @return the columnType
*/
public List getColumnType() {
return this.columnType;
}
/**
* @param columnType
* the columnType to set
*/
public void setColumnType(List columnType) {
this.columnType = columnType;
}
/**
* @return the columnTypeName
*/
public List getColumnTypeName() {
return this.columnTypeName;
}
/**
* @param columnTypeName
* the columnTypeName to set
*/
public void setColumnTypeName(List columnTypeName) {
this.columnTypeName = columnTypeName;
}
/**
* @return the readOnly
*/
public List getReadOnly() {
return this.readOnly;
}
/**
* @param readOnly
* the readOnly to set
*/
public void setReadOnly(List readOnly) {
this.readOnly = readOnly;
}
/**
* @return the writable
*/
public List getWritable() {
return this.writable;
}
/**
* @param writable
* the writable to set
*/
public void setWritable(List writable) {
this.writable = writable;
}
/**
* @return the definitelyWritable
*/
public List getDefinitelyWritable() {
return this.definitelyWritable;
}
/**
* @param definitelyWritable
* the definitelyWritable to set
*/
public void setDefinitelyWritable(List definitelyWritable) {
this.definitelyWritable = definitelyWritable;
}
/**
* @return the columnClassName
*/
public List getColumnClassName() {
return this.columnClassName;
}
/**
* @param columnClassName
* the columnClassName to set
*/
public void setColumnClassName(List columnClassName) {
this.columnClassName = columnClassName;
}
/**
* @return the columnCount
*/
public int getColumnCount() {
return this.columnCount;
}
/**
* @param columnCount
* the columnCount to set
*/
public void setColumnCount(int columnCount) {
this.columnCount = columnCount;
}
/*
* (non-Javadoc)
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return "ResultSetMetaDataHolder [columnCount=" + this.columnCount
+ ", autoIncrement=" + this.autoIncrement + ", caseSensitive="
+ this.caseSensitive + ", searchable=" + this.searchable
+ ", currency=" + this.currency + ", nullable=" + this.nullable
+ ", signed=" + this.signed + ", columnDisplaySize="
+ this.columnDisplaySize + ", columnLabel=" + this.columnLabel
+ ", columnName=" + this.columnName + ", schemaName="
+ this.schemaName + ", precision=" + this.precision
+ ", scale=" + this.scale + ", tableName=" + this.tableName
+ ", catalogName=" + this.catalogName + ", columnType="
+ this.columnType + ", columnTypeName=" + this.columnTypeName
+ ", readOnly=" + this.readOnly + ", writable=" + this.writable
+ ", definitelyWritable=" + this.definitelyWritable
+ ", columnClassName=" + this.columnClassName + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy