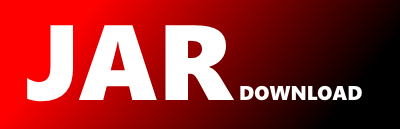
org.kawanfw.sql.tomcat.util.LinkedProperties Maven / Gradle / Ivy
/*
* This file is part of AceQL.
* AceQL: Remote JDBC access over HTTP.
* Copyright (C) 2015, KawanSoft SAS
* (http://www.kawansoft.com). All rights reserved.
*
* AceQL is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* AceQL is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*
* Any modifications to this file must keep this entire header
* intact.
*/
package org.kawanfw.sql.tomcat.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.Properties;
import java.util.Set;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
/**
*
* @author Nicolas de Pomereu
*
* Allows to read a properties with property names ordered on insertion
* order passed to constructor. (Just for reading, ordered store is not
* supported.)
*/
public class LinkedProperties extends Properties {
/**
* Generated serial ID
*/
private static final long serialVersionUID = 6588861237819163772L;
/** The ordered set of properties */
private Set orderedPropNames = new LinkedHashSet();
/**
* Constructor
*
* @param orderedPropNames
* the ordered properties names
*/
public LinkedProperties(Set orderedPropNames) {
this.orderedPropNames = orderedPropNames;
}
/**
* Returns a set of keys in this property list where the key and its
* corresponding value are strings, including distinct keys in the default
* property list if a key of the same name has not already been found from
* the main properties list. Properties whose key or value is not of type
* String are omitted.
*
* The returned set is not backed by the Properties object. Changes
* to this Properties are not reflected in the set, or vice versa.
*
* @return a set of keys in this property list where the key and its
* corresponding value are strings, including the keys in the
* default property list.
* @see java.util.Properties#defaults
* @since 1.6
*/
@Override
public Set stringPropertyNames() {
Set orderedPropNamesNew = new LinkedHashSet();
for (Iterator iterator = orderedPropNames.iterator(); iterator
.hasNext();) {
String propertyName = (String) iterator.next();
if (super.containsKey(propertyName)) {
orderedPropNamesNew.add(propertyName);
}
}
return orderedPropNamesNew;
}
/*
* (non-Javadoc)
*
* @see java.util.Properties#propertyNames()
*/
@Override
public Enumeration> propertyNames() {
Set orderedPropNamesNew = new LinkedHashSet();
for (Iterator iterator = orderedPropNames.iterator(); iterator
.hasNext();) {
String propertyName = (String) iterator.next();
if (super.containsKey(propertyName)) {
orderedPropNamesNew.add(propertyName);
}
}
return Collections.enumeration(orderedPropNamesNew);
}
/*
* (non-Javadoc)
*
* @see java.util.Hashtable#keys()
*/
@Override
public synchronized Enumeration