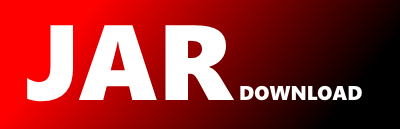
com.acgist.snail.net.UdpMessageHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snail Show documentation
Show all versions of snail Show documentation
基于Java开发的下载工具,支持下载协议:BT(BitTorrent、磁力链接、种子文件)、HLS(M3U8)、FTP、HTTP。
package com.acgist.snail.net;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.SocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.DatagramChannel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.acgist.snail.context.exception.NetException;
/**
* UDP消息代理
*
* @author acgist
*/
public abstract class UdpMessageHandler extends MessageHandler {
private static final Logger LOGGER = LoggerFactory.getLogger(UdpMessageHandler.class);
/**
* 远程地址
*
* 远程地址管理
*
* 类型
* 描述
* 参考
*
*
* 客户端
* 初始化固定地址
* UdpClient子类
*
*
* 服务端(只要接收)
* 不用管理地址
* LSD/Stun/UPNP/Tracker
*
*
* 服务端(需要发送)
* 单独管理消息代理
* UTP
*
*
* 服务端(需要发送)
* 没有管理消息代理:直接使用消息地址
* DHT
*
*
*/
protected final InetSocketAddress socketAddress;
/**
* @param socketAddress 远程地址
*/
protected UdpMessageHandler(InetSocketAddress socketAddress) {
this.socketAddress = socketAddress;
}
@Override
public void handle(DatagramChannel channel) {
this.channel = channel;
}
@Override
public void send(ByteBuffer buffer, int timeout) throws NetException {
this.send(buffer, this.remoteSocketAddress());
}
@Override
public InetSocketAddress remoteSocketAddress() {
return this.socketAddress;
}
@Override
public void close() {
LOGGER.debug("UDP连接关闭:{}", this.socketAddress);
// 标记关闭:不能关闭通道(UDP通道单例复用)
this.close = true;
}
/**
* 发送消息
*
* @param buffer 消息
* @param socketAddress 地址
*
* @throws NetException 网络异常
*/
protected final void send(ByteBuffer buffer, SocketAddress socketAddress) throws NetException {
this.check(buffer);
try {
// UDP不用加锁
final int size = this.channel.send(buffer, socketAddress);
if(size <= 0) {
LOGGER.warn("UDP消息发送失败:{}-{}", socketAddress, size);
}
} catch (IOException e) {
throw new NetException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy