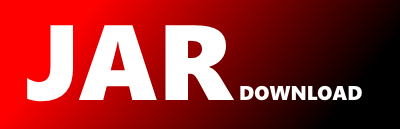
com.acgist.snail.net.application.ApplicationMessageHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snail Show documentation
Show all versions of snail Show documentation
基于Java开发的下载工具,支持下载协议:BT(BitTorrent、磁力链接、种子文件)、HLS(M3U8)、FTP、HTTP。
package com.acgist.snail.net.application;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.acgist.snail.config.SymbolConfig;
import com.acgist.snail.context.GuiContext;
import com.acgist.snail.context.GuiContext.Mode;
import com.acgist.snail.context.SystemContext;
import com.acgist.snail.context.TaskContext;
import com.acgist.snail.context.exception.DownloadException;
import com.acgist.snail.context.exception.NetException;
import com.acgist.snail.context.exception.PacketSizeException;
import com.acgist.snail.format.BEncodeDecoder;
import com.acgist.snail.format.BEncodeEncoder;
import com.acgist.snail.net.TcpMessageHandler;
import com.acgist.snail.net.codec.IMessageDecoder;
import com.acgist.snail.net.codec.IMessageEncoder;
import com.acgist.snail.net.codec.LineMessageCodec;
import com.acgist.snail.net.codec.StringMessageCodec;
import com.acgist.snail.pojo.ITaskSession;
import com.acgist.snail.pojo.message.ApplicationMessage;
import com.acgist.snail.pojo.message.GuiMessage;
import com.acgist.snail.utils.StringUtils;
/**
* 系统消息代理
*
* @author acgist
*/
public final class ApplicationMessageHandler extends TcpMessageHandler implements IMessageDecoder {
private static final Logger LOGGER = LoggerFactory.getLogger(ApplicationMessageHandler.class);
/**
* 消息编码器
*/
private final IMessageEncoder messageEncoder;
public ApplicationMessageHandler() {
final var lineMessageCodec = new LineMessageCodec(this, SymbolConfig.LINE_SEPARATOR_COMPAT);
final var stringMessageCodec = new StringMessageCodec(lineMessageCodec);
this.messageDecoder = stringMessageCodec;
this.messageEncoder = lineMessageCodec;
}
/**
* 发送系统消息
*
* @param message 系统消息
*/
public void send(ApplicationMessage message) {
try {
this.send(message.toString());
} catch (NetException e) {
LOGGER.error("发送系统消息异常", e);
}
}
@Override
public void send(String message, String charset) throws NetException {
super.send(this.messageEncoder.encode(message), charset);
}
@Override
public void onMessage(String message) {
if(StringUtils.isEmpty(message)) {
LOGGER.warn("系统消息错误:{}", message);
return;
}
message = message.trim();
final ApplicationMessage applicationMessage = ApplicationMessage.valueOf(message);
if(applicationMessage == null) {
LOGGER.warn("系统消息错误(格式):{}", message);
return;
}
this.execute(applicationMessage);
}
/**
* 处理系统消息
*
* @param message 系统消息
*/
private void execute(ApplicationMessage message) {
final ApplicationMessage.Type type = message.getType();
if(type == null) {
LOGGER.warn("系统消息错误(未知类型):{}", type);
return;
}
LOGGER.debug("处理系统消息:{}", message);
switch (type) {
case GUI -> this.onGui();
case TEXT -> this.onText(message);
case CLOSE -> this.onClose();
case NOTIFY -> this.onNotify();
case SHUTDOWN -> this.onShutdown();
case TASK_NEW -> this.onTaskNew(message);
case TASK_LIST -> this.onTaskList();
case TASK_START -> this.onTaskStart(message);
case TASK_PAUSE -> this.onTaskPause(message);
case TASK_DELETE -> this.onTaskDelete(message);
case SHOW -> this.onShow();
case HIDE -> this.onHide();
case ALERT -> this.onAlert(message);
case NOTICE -> this.onNotice(message);
case REFRESH_TASK_LIST -> this.onRefreshTaskList();
case REFRESH_TASK_STATUS -> this.onRefreshTaskStatus();
case RESPONSE -> this.onResponse(message);
default -> LOGGER.warn("系统消息错误(类型未适配):{}", type);
}
}
/**
* 扩展GUI注册
* 将当前连接的消息代理注册为GUI消息代理
*
* @see Mode#EXTEND
*/
private void onGui() {
final boolean success = GuiContext.getInstance().extendGuiMessageHandler(this);
if(success) {
this.send(ApplicationMessage.Type.RESPONSE.build(ApplicationMessage.SUCCESS));
} else {
this.send(ApplicationMessage.Type.RESPONSE.build(ApplicationMessage.FAIL));
}
}
/**
* 文本消息
*
* @param message 系统消息
*/
private void onText(ApplicationMessage message) {
this.send(ApplicationMessage.Type.RESPONSE.build(message.getBody()));
}
/**
* 关闭连接
*/
private void onClose() {
this.close();
}
/**
* 唤醒窗口
*/
private void onNotify() {
GuiContext.getInstance().show();
}
/**
* 关闭程序
*/
private void onShutdown() {
SystemContext.shutdown();
}
/**
* 新建任务
*
* - body:Map(B编码)
* - url:下载链接
* - files:选择下载文件列表(B编码)
*
*
* @param message 系统消息
*/
private void onTaskNew(ApplicationMessage message) {
final String body = message.getBody();
try {
final var decoder = BEncodeDecoder.newInstance(body).next();
if(decoder.isEmpty()) {
this.send(ApplicationMessage.Type.RESPONSE.build(ApplicationMessage.FAIL));
return;
}
final String url = decoder.getString("url");
final String files = decoder.getString("files");
synchronized (this) {
// 设置选择文件
GuiContext.getInstance().files(files);
// 开始下载任务
TaskContext.getInstance().download(url);
}
this.send(ApplicationMessage.Type.RESPONSE.build(ApplicationMessage.SUCCESS));
} catch (NetException | DownloadException e) {
this.send(ApplicationMessage.Type.RESPONSE.build(e.getMessage()));
}
}
/**
* 任务列表
* 返回任务列表(B编码)
*/
private void onTaskList() {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy