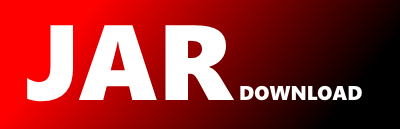
com.actelion.research.chem.SmilesParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
/*
* Copyright (c) 1997 - 2016
* Actelion Pharmaceuticals Ltd.
* Gewerbestrasse 16
* CH-4123 Allschwil, Switzerland
*
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of the the copyright holder nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR
* ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
*/
package com.actelion.research.chem;
import java.util.TreeMap;
public class SmilesParser {
private static final int MAX_BRACKET_LEVELS = 64;
private static final int MAX_RE_CONNECTIONS = 64;
private static final int MAX_AROMATIC_RING_SIZE = 15;
private StereoMolecule mMol;
private boolean[] mIsAromaticBond;
/**
* Parses the given smiles into the molecule, creates proper atom coordinates
* to reflect correct double bond geometries and translates tetrahedral and allene
* parities into up/down-bonds.
* @param mol
* @param smiles
* @throws Exception
*/
public void parse(StereoMolecule mol, String smiles) throws Exception {
parse(mol, smiles.getBytes(), true, true);
}
public void parse(StereoMolecule mol, byte[] smiles) throws Exception {
parse(mol, smiles, true, true);
}
public void parse(StereoMolecule mol, byte[] smiles, boolean createCoordinates, boolean readStereoFeatures) throws Exception {
mMol = mol;
mMol.deleteMolecule();
TreeMap parityMap = null;
int[] baseAtom = new int[MAX_BRACKET_LEVELS];
baseAtom[0] = -1;
int[] ringClosureAtom = new int[MAX_RE_CONNECTIONS];
int[] ringClosurePosition = new int[MAX_RE_CONNECTIONS];
boolean[] ringClosureInUse = new boolean[MAX_RE_CONNECTIONS];
for (int i=0; i();
// using position as hydrogenPosition is close enough
parityMap.put(atom, new THParity(atom, fromAtom, explicitHydrogens, position, isClockwise));
}
}
continue;
}
if (theChar == '.') {
bondType = Molecule.cBondTypeDeleted;
continue;
}
if (theChar == '=') {
bondType = Molecule.cBondTypeDouble;
continue;
}
if (theChar == '#') {
bondType = Molecule.cBondTypeTriple;
continue;
}
if (Character.isDigit(theChar)) {
int number = theChar - '0';
if (squareBracketOpen) {
while (position < smilesLength
&& Character.isDigit(smiles[position])) {
number = 10 * number + smiles[position] - '0';
position++;
}
atomMass = number;
}
else {
if (percentFound
&& position < smilesLength
&& Character.isDigit(smiles[position])) {
number = 10 * number + smiles[position] - '0';
position++;
}
percentFound = false;
if (number >= MAX_RE_CONNECTIONS)
throw new Exception("SmilesParser: ringClosureAtom number out of range");
if (ringClosureAtom[number] == -1) {
ringClosureAtom[number] = baseAtom[bracketLevel];
ringClosurePosition[number] = position-1;
}
else {
if (ringClosureAtom[number] == baseAtom[bracketLevel])
throw new Exception("SmilesParser: ring closure to same atom");
if (readStereoFeatures && parityMap != null) {
THParity parity = parityMap.get(ringClosureAtom[number]);
if (parity != null)
parity.addNeighbor(baseAtom[bracketLevel], ringClosurePosition[number], false);
parity = parityMap.get(baseAtom[bracketLevel]);
if (parity != null)
parity.addNeighbor(ringClosureAtom[number], position-1, false);
}
mMol.addBond(baseAtom[bracketLevel], ringClosureAtom[number], bondType);
ringClosureAtom[number] = -1; // for number re-usage
}
bondType = Molecule.cBondTypeSingle;
}
continue;
}
if (theChar == '+') {
if (!squareBracketOpen)
throw new Exception("SmilesParser: '+' found outside brackets");
int charge = 1;
while (smiles[position] == '+') {
charge++;
position++;
}
if (charge == 1 && Character.isDigit(smiles[position])) {
charge = smiles[position] - '0';
position++;
}
mMol.setAtomCharge(baseAtom[bracketLevel], charge);
continue;
}
if (theChar == '-') {
if (!squareBracketOpen)
continue; // single bond
int charge = -1;
while (smiles[position] == '-') {
charge--;
position++;
}
if (charge == -1 && Character.isDigit(smiles[position])) {
charge = '0' - smiles[position];
position++;
}
mMol.setAtomCharge(baseAtom[bracketLevel], charge);
continue;
}
if (theChar == '(') {
if (baseAtom[bracketLevel] == -1)
throw new Exception("Smiles with leading parenthesis are not supported");
baseAtom[bracketLevel+1] = baseAtom[bracketLevel];
bracketLevel++;
continue;
}
if (theChar == ')') {
bracketLevel--;
continue;
}
if (theChar == '[') {
if (squareBracketOpen)
throw new Exception("SmilesParser: nested square brackets found");
squareBracketOpen = true;
continue;
}
if (theChar == ']') {
if (!squareBracketOpen)
throw new Exception("SmilesParser: closing bracket without opening one");
squareBracketOpen = false;
continue;
}
if (theChar == '%') {
percentFound = true;
continue;
}
/* if (theChar == '.') {
if (bracketLevel != 0)
throw new Exception("SmilesParser: '.' found within brackets");
baseAtom[0] = -1;
// for (int i=0; i=4; qualifyingNo--) {
do {
qualifyingBondFound = false;
for (int bond=0; bond 3
&& mMol.getAtomPi(atom) > 0) {
for (int i=0; i 1)
&& mMol.isElectronegative(connAtom)) {
if (mMol.getBondType(connBond) == Molecule.cBondTypeTriple)
mMol.setBondType(connBond, Molecule.cBondTypeDouble);
else
mMol.setBondType(connBond, Molecule.cBondTypeSingle);
mMol.setAtomCharge(atom, mMol.getAtomCharge(atom) + 1);
mMol.setAtomCharge(connAtom, mMol.getAtomCharge(connAtom) - 1);
break;
}
}
}
}
}
private boolean resolveStereoBonds() {
mMol.ensureHelperArrays(Molecule.cHelperRings);
boolean paritiesFound = false;
int[] refAtom = new int[2];
int[] refBond = new int[2];
int[] otherAtom = new int[2];
for (int bond=0; bond mNeighborPosition[i]) {
minPosition = mNeighborPosition[i];
minIndex = i;
}
}
mFromAtom = mNeighborAtom[minIndex];
for (int i=minIndex+1; i 4 || totalNeighborCount < 3)
return Molecule.cAtomParityUnknown;
// We look from the hydrogen towards the central carbon if the fromAtom is a hydrogen or
// if there is no fromAtom but the central atom has an implicit hydrogen.
boolean fromAtomIsHydrogen = (mFromAtom == -1 && mImplicitHydrogen == 1)
|| (mFromAtom != -1 && mMol.isSimpleHydrogen(mFromAtom));
int hydrogenNeighborIndex = -1;
for (int i=0; i atom[i])
inversion = !inversion;
if (position[j] > position[i])
inversion = !inversion;
}
}
return inversion;
}
}
public static void main(String[] args) {
System.out.println("ID-code equivalence test:");
final String[][] data = { { "N[C@@]([H])(C)C(=O)O", "S-alanine", "gGX`BDdwMUM@@" },
{ "N[C@@H](C)C(=O)O", "S-alanine", "gGX`BDdwMUM@@" },
{ "N[C@H](C(=O)O)C", "S-alanine", "gGX`BDdwMUM@@" },
{ "[H][C@](N)(C)C(=O)O", "S-alanine", "gGX`BDdwMUM@@" },
{ "[C@H](N)(C)C(=O)O", "S-alanine", "gGX`BDdwMUM@@" },
{ "N[C@]([H])(C)C(=O)O", "R-alanine", "gGX`BDdwMUL`@" },
{ "N[C@H](C)C(=O)O", "R-alanine", "gGX`BDdwMUL`@" },
{ "N[C@@H](C(=O)O)C", "R-alanine", "gGX`BDdwMUL`@" },
{ "[H][C@@](N)(C)C(=O)O", "R-alanine", "gGX`BDdwMUL`@" },
{ "[C@@H](N)(C)C(=O)O", "R-alanine", "gGX`BDdwMUL`@" },
{ "C[C@H]1CCCCO1", "S-Methyl-pyran", "gOq@@eLm]UUH`@" },
{ "O1CCCC[C@@H]1C", "S-Methyl-pyran", "gOq@@eLm]UUH`@" },
{ "[C@H](F)(B)O", "S-Methyl-oxetan", "gCaDDICTBSURH@" },
{ "C1CO[C@H]1C", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "C1CO[C@@H](C)1", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "[C@H]1(C)CCO1", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "[H][C@]1(C)CCO1", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "[H][C@@]1(CCO1)C", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "[C@@]1([H])(C)CCO1", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "[C@]1(C)([H])CCO1", "S-Methyl-oxetan", "gKQ@@eLmUTb@" },
{ "C1[C@@H]2COC2=N1", "oxetan-azetin", "gGy@LDimDvfja`@" },
{ "CC(C)[C@@]12C[C@@H]1[C@@H](C)C(=O)C2", "alpha-thujone", "dmLH@@RYe~IfyjjjkDaIh@" },
{ "CN1CCC[C@H]1c2cccnc2", "Nicotine", "dcm@@@{IDeCEDUSh@UUECP@" },
{ "CC[C@H](O1)CC[C@@]12CCCO2", "2S,5R-Chalcogran", "dmLD@@qJZY|fFZjjjdbH`@" },
{ "CCCC", "butane", "gC`@Dij@@" },
{ "C1C.CC1", "butane", "gC`@Dij@@" },
{ "[CH3][CH2][CH2][CH3]", "butane", "gC`@Dij@@" },
{ "C-C-C-C", "butane", "gC`@Dij@@" },
{ "C12.C1.CC2", "butane", "gC`@Dij@@" },
{ "[Na+].[Cl-]", "NaCl", "eDARHm@zd@@" },
{ "[Na+]-[Cl-]", "NaCl", "error" },
{ "[Na+]1.[Cl-]1", "NaCl", "error" },
{ "c1ccccc1", "benzene", "gFp@DiTt@@@" },
{ "C1=C-C=C-C=C1", "benzene", "gFp@DiTt@@@" },
{ "C1:C:C:C:C:C:1", "benzene", "gFp@DiTt@@@" },
{ "c1ccncc1", "pyridine", "gFx@@eJf`@@@" },
{ "[nH]1cccc1", "pyrrole", "gKX@@eKcRp@" },
{ "N1C=C-C=C1", "pyrrole", "gKX@@eKcRp@" },
{ "[H]n1cccc1", "pyrrole", "gKX@@eKcRp@" },
{ "[H]n1cccc1", "pyrrole", "gKX@@eKcRp@" },
{ "c1cncc1", "pyrrole no [nH]", "error" },
{ "[13CH4]", "C13-methane", "fH@FJp@" },
{ "[35ClH]", "35-chlorane", "fHdP@qX`" },
{ "[35Cl-]", "35-chloride", "fHtPxAbq@" },
{ "[Na+].[O-]c1ccccc1", "Na-phenolate", "daxHaHCPBXyAYUn`@@@" },
{ "c1cc([O-].[Na+])ccc1", "Na-phenolate", "daxHaHCPBXyAYUn`@@@" },
{ "C[C@@](C)(O1)C[C@@H](O)[C@@]1(O2)[C@@H](C)[C@@H]3CC=C4[C@]3(C2)C(=O)C[C@H]5[C@H]4CC[C@@H](C6)[C@]5(C)Cc(n7)c6nc(C[C@@]89(C))c7C[C@@H]8CC[C@@H]%10[C@@H]9C[C@@H](O)[C@@]%11(C)C%10=C[C@H](O%12)[C@]%11(O)[C@H](C)[C@]%12(O%13)[C@H](O)C[C@@]%13(C)CO",
"Cephalostatin-1",
"gdKe@h@@K`H@XjKHuYlnoP\\bbdRbbVTLbTrJbRaQRRRbTJTRTrfrfTTOBPHtFODPhLNSMdIERYJmShLfs]aqy|uUMUUUUUUE@UUUUMUUUUUUTQUUTPR`nDdQQKB|RIFbiQeARuQt`rSSMNtGS\\ct@@" },
};
StereoMolecule mol = new StereoMolecule();
for (String[] test:data) {
try {
new SmilesParser().parse(mol, test[0]);
String idcode = new Canonizer(mol).getIDCode();
if (test[2].equals("error"))
System.out.println("Should create error! "+test[1]+" smiles:"+test[0]+" idcode:"+idcode);
else if (!test[2].equals(idcode))
System.out.println("ERROR! "+test[1]+" smiles:"+test[0]+" is:"+idcode+" must:"+test[2]);
}
catch (Exception e) {
if (!test[2].equals("error"))
System.out.println("ERROR! "+test[1]+" smiles:"+test[0]+" exception:"+e.getMessage());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy