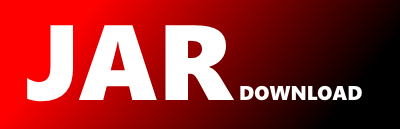
com.actelion.research.chem.phesa.MolecularVolume Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
package com.actelion.research.chem.phesa;
import com.actelion.research.chem.Coordinates;
import com.actelion.research.chem.StereoMolecule;
import com.actelion.research.chem.conf.Conformer;
import com.actelion.research.chem.phesa.pharmacophore.IPharmacophorePoint;
import com.actelion.research.chem.phesa.pharmacophore.IonizableGroupDetector;
import com.actelion.research.chem.phesa.pharmacophore.PPGaussian;
import com.actelion.research.chem.phesa.pharmacophore.PharmacophoreCalculator;
import java.util.ArrayList;
import java.util.List;
import com.actelion.research.util.EncoderFloatingPointNumbers;
/**
* @version: 1.0, February 2018
* Author: J. Wahl
* class to approximate the volume of a molecule as a sum of atom-centered Gaussians, as introduced by Grant and Pickup, J. Phys. Chem. 1995, 99, 3503-3510
* no higher order terms (atom-atom overlaps) are calculated to reduce computational costs
*/
public class MolecularVolume {
static public final double p = 2.82842712475; // height of Atomic Gaussian, 2*sqrt(2), commonly used in the literature: Haque and Pande, DOI 10.1002/jcc.11307
static public final double alpha_pref = 2.41798793102; // taken from DOI 10.1002/jcc.11307
private double volume;
private Coordinates com;
private ArrayList atomicGaussians;
private ArrayList ppGaussians;
private ArrayList volumeGaussians; //exclusion and inclusion spheres
private ArrayList hydrogens;
public MolecularVolume(List atomicGaussiansInp,List ppGaussiansInp,List volGaussians,
List hydrogenCoords) {
this.volume = 0.0;
this.atomicGaussians = new ArrayList();
for(AtomicGaussian ag : atomicGaussiansInp) {
atomicGaussians.add(new AtomicGaussian(ag));
}
this.ppGaussians = new ArrayList();
for(PPGaussian pg : ppGaussiansInp) {
ppGaussians.add(new PPGaussian(pg));
}
this.hydrogens = new ArrayList();
for(Coordinates hydrogen : hydrogenCoords) {
this.hydrogens.add(hydrogen);
}
this.volumeGaussians = new ArrayList();
for(VolumeGaussian eg : volGaussians) {
this.volumeGaussians.add(new VolumeGaussian(eg));
}
this.calcCOM();
}
public void updateCOM() {
this.calcCOM();
}
private void updateAtomIndeces(List extends Gaussian3D> gaussians,int[] map) {
for(Gaussian3D gaussian:gaussians)
gaussian.updateAtomIndeces(map);
}
public void updateAtomIndeces(int[] map) {
updateAtomIndeces(ppGaussians,map);
updateAtomIndeces(atomicGaussians,map);
updateAtomIndeces(volumeGaussians,map);
}
public MolecularVolume(StereoMolecule mol) {
this.volume = 0.0;
this.hydrogens = new ArrayList();
this.volumeGaussians = new ArrayList();
this.calc(mol);
this.calcPPVolume(mol);
this.calcCOM();
}
public MolecularVolume(MolecularVolume original, Conformer conf) {
this(original);
update(conf);
}
public MolecularVolume(MolecularVolume original) {
this.volume = new Double(original.volume);
this.atomicGaussians = new ArrayList();
this.ppGaussians = new ArrayList();
this.volumeGaussians = new ArrayList();
for(AtomicGaussian ag : original.getAtomicGaussians()) {
this.atomicGaussians.add(new AtomicGaussian(ag));
}
for(PPGaussian pg : original.getPPGaussians()) {
this.ppGaussians.add(new PPGaussian(pg));
}
this.hydrogens = new ArrayList();
for(Coordinates hydrogen : original.hydrogens) {
this.hydrogens.add(new Coordinates(hydrogen));
}
for(VolumeGaussian eg : original.volumeGaussians) {
this.volumeGaussians.add(new VolumeGaussian(eg));
}
this.com = new Coordinates(original.com);
}
/**
* calculates the molecular Volume for a StereoMolecule with 3D coordinates
* @param mol
*/
private void calc(StereoMolecule mol) {
this.atomicGaussians = new ArrayList();
int nrOfAtoms = mol.getAllAtoms();
for (int i=0;i();
List ppPoints = new ArrayList();
IonizableGroupDetector detector = new IonizableGroupDetector(mol);
ppPoints.addAll(detector.detect());
ppPoints.addAll(PharmacophoreCalculator.getPharmacophorePoints(mol));
for(IPharmacophorePoint ppPoint : ppPoints )
ppGaussians.add(new PPGaussian(6,ppPoint));
}
/**
* calculates volume weighted center of mass of the molecular Volume
*/
private void calcCOM(){
double volume = 0.0;
double comX = 0.0;
double comY = 0.0;
double comZ = 0.0;
for(AtomicGaussian atGauss : this.atomicGaussians){
volume += atGauss.getVolume();
comX += atGauss.getCenter().x*atGauss.getVolume();
comY += atGauss.getCenter().y*atGauss.getVolume();
comZ += atGauss.getCenter().z*atGauss.getVolume();
}
for(VolumeGaussian volGauss : this.volumeGaussians){
volume += volGauss.getRole()*volGauss.getVolume();
comX += volGauss.getRole()*volGauss.getCenter().x*volGauss.getVolume();
comY += volGauss.getRole()*volGauss.getCenter().y*volGauss.getVolume();
comZ += volGauss.getRole()*volGauss.getCenter().z*volGauss.getVolume();
}
comX = comX/volume;
comY = comY/volume;
comZ = comZ/volume;
this.volume = volume;
this.com = new Coordinates(comX,comY,comZ);
}
public Coordinates getCOM() {
this.calcCOM();
return this.com;
}
public ArrayList getAtomicGaussians() {
return this.atomicGaussians;
}
public ArrayList getPPGaussians() {
return this.ppGaussians;
}
public ArrayList getVolumeGaussians() {
return this.volumeGaussians;
}
public ArrayList getHydrogens() {
return this.hydrogens;
}
private void updateHydrogens(StereoMolecule mol) {
int h = 0;
for(int i = mol.getAtoms();i gaussians, StereoMolecule mol) {
for(Gaussian3D gaussian : gaussians) {
gaussian.updateCoordinates(mol);
}
}
private void updateCoordinates(ArrayList extends Gaussian3D> gaussians, Conformer conf) {
for(Gaussian3D gaussian : gaussians) {
gaussian.updateCoordinates(conf);
}
}
public void translateToCOM(Coordinates com) {
for (AtomicGaussian ag : getAtomicGaussians()){
ag.getCenter().sub(com); //translate atomicGaussians. Moves center of mass to the origin.
}
for (PPGaussian pg : getPPGaussians()){
pg.getCenter().sub(com); //translate atomicGaussians. Moves center of mass to the origin.
}
for (VolumeGaussian vg : getVolumeGaussians()){
vg.translateRef(com.scaleC(-1.0)); //translate atomicGaussians. Moves center of mass to the origin.
}
for (Coordinates hydrogen : getHydrogens()){
hydrogen.sub(com); //translate atomicGaussians. Moves center of mass to the origin.
}
}
public String encodeFull() {
StringBuilder molVolString = new StringBuilder();
molVolString.append(Integer.toString(atomicGaussians.size()));
molVolString.append(" ");
for(AtomicGaussian ag : atomicGaussians) {
molVolString.append(ag.encode());
molVolString.append(" ");
}
molVolString.append(Integer.toString(ppGaussians.size()));
molVolString.append(" ");
for(PPGaussian pg : ppGaussians) {
molVolString.append(pg.encode().trim());
molVolString.append(" ");
}
molVolString.append(Integer.toString(volumeGaussians.size()));
molVolString.append(" ");
for(VolumeGaussian vg : volumeGaussians) {
molVolString.append(vg.encode());
molVolString.append(" ");
}
double[] hydrogenCoords = new double[3*hydrogens.size()];
for(int i=0;i referenceAtomicGaussians = reference.getAtomicGaussians();
ArrayList referencePPGaussians = reference.getPPGaussians();
ArrayList referenceVolGaussians = reference.getVolumeGaussians();
String[] splitString = string.split(" ");
double[] atomicGaussiansCoords = EncoderFloatingPointNumbers.decode(splitString[0]);
double[] ppGaussiansCoords = EncoderFloatingPointNumbers.decode(splitString[1]);
double[] ppGaussiansDirectionalities = EncoderFloatingPointNumbers.decode(splitString[2]);
double[] volumeGaussiansRefCoords = EncoderFloatingPointNumbers.decode(splitString[3]);
double[] volumeGaussiansShiftCoords = EncoderFloatingPointNumbers.decode(splitString[4]);
double[] hydrogensCoords = EncoderFloatingPointNumbers.decode(splitString[5]);
ArrayList atomicGaussians = new ArrayList();
ArrayList ppGaussians = new ArrayList();
ArrayList volumeGaussians = new ArrayList();
ArrayList hydrogens = new ArrayList();
int nrOfAtomicGaussians = atomicGaussiansCoords.length/3;
int nrOfHydrogens = hydrogensCoords.length/3;
int nrOfPPGaussians = ppGaussiansCoords.length/3;
int nrOfVolumeGaussians = volumeGaussiansRefCoords.length/3;
for(int i=0;i atomicGaussians = new ArrayList();
List ppGaussians = new ArrayList();
List volumeGaussians = new ArrayList();
List hydrogens = new ArrayList();
for(int i=firstIndex;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy