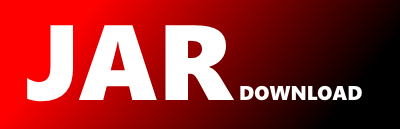
com.actelion.research.chem.phesa.PheSABindingHypothesis Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
package com.actelion.research.chem.phesa;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.stream.IntStream;
import com.actelion.research.chem.StereoMolecule;
/**
* @author joel
* Algorithm to create to align several active compounds in abscence of a bioactive conformation
*/
public class PheSABindingHypothesis {
private static final int SOLUTIONS = 5; //report 5 best hypotheses
private double[][] similarityMatrix;
private List actives;
private int[] nConfs;
private int nProcesses;
public PheSABindingHypothesis(List actives) {
this(actives,1);
}
public PheSABindingHypothesis(List actives, int nProcesses) {
this.actives = actives;
this.nProcesses = nProcesses;
}
public StereoMolecule[][] generate() {
List phesaModels = new ArrayList();
DescriptorHandlerShape dhs = new DescriptorHandlerShape();
actives.forEach(e -> phesaModels.add(dhs.createDescriptor(e)));
nConfs = new int[actives.size()];
IntStream.range(0,actives.size()).forEach(e -> nConfs[e]=phesaModels.get(e).getVolumes().size());
int totNrStrucs = Arrays.stream(nConfs).sum();
similarityMatrix = new double[totNrStrucs][totNrStrucs];
StereoMolecule[][] alignments = new StereoMolecule[SOLUTIONS][actives.size()];
if(nProcesses==1)
calculateSimilarityMatrix(phesaModels);
else
calculateSimilarityMatrixParallel(phesaModels);
int[][] bestEnsembles = getBestEnsembles();
int counter = 0;
for(int[] bestEnsemble : bestEnsembles) {
int referenceConfIndex = bestEnsemble[bestEnsemble.length-1]; //index in similarity matrix
int[] molIndexconfIndex = confIndexToMolIndex(referenceConfIndex);
int molIndex = molIndexconfIndex[0];
int confIndex = molIndexconfIndex[1];
PheSAMolecule refModel = phesaModels.get(molIndex);
MolecularVolume refVol = refModel.getVolumes().get(confIndex);
StereoMolecule refMol = refModel.getConformer(refVol);
StereoMolecule[] alignment = alignments[counter];
alignment[0] = refMol;
int i=0;
for(int index : bestEnsemble) {
if(index==referenceConfIndex)
continue;
else {
i++;
molIndexconfIndex = confIndexToMolIndex(index);
molIndex = molIndexconfIndex[0];
confIndex = molIndexconfIndex[1];
PheSAMolecule fitModel = phesaModels.get(molIndex);
MolecularVolume fitVol = fitModel.getVolumes().get(confIndex);
StereoMolecule fitMol = fitModel.getConformer(fitVol);
PheSAAlignmentOptimizer.align(fitModel, refVol, fitVol, fitMol,0.5);
alignment[i] = fitMol;
}
}
counter++;
}
return alignments;
}
private int[][] getBestEnsembles() {
double[] bestScores = new double[SOLUTIONS];
IntStream.range(0, bestScores.length).forEach(i -> bestScores[i]=-Double.MAX_VALUE);
int[][] bestEnsembles = new int[SOLUTIONS][nConfs.length+1]; //the last one is the reference
for(int refMol=0;refMolbestScores[i]) {
rank = i;
break;
}
}
for(int j=bestScores.length-1;j>rank;j--) {
bestScores[j] = bestScores[j-1];
bestEnsembles[j] = bestEnsembles[j-1];
}
bestScores[rank] = score;
bestEnsembles[rank] = ensemble;
}
}
private void calculateSimilarityMatrix(List phesaModels) {
for(int i=0;i phesaModels) {
ExecutorService executorService = Executors.newFixedThreadPool(nProcesses);
for(int i=0;i compareOneConfWithAll(refVol,molIndex,confIndex,phesaModels));
}
}
executorService.shutdown();
while(!executorService.isTerminated()){
try {
Thread.sleep(500);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
private void compareOneConfWithAll(MolecularVolume refVol,int molIndex,int confIndex, List phesaModels) {
for(int k=molIndex+1;kmaxValue) {
maxValue = arr[i];
maxIndex = i;
}
}
return maxIndex;
}
/* returns the index of the molecule and the index of the conformer with
*respect to this very molecule
*/
private int[] confIndexToMolIndex(int confIndex) {
int sum = 0;
int indexMol = 0;
int indexConf = 0;
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy