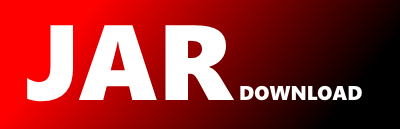
com.actelion.research.calc.combinatorics.CombinationGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
package com.actelion.research.calc.combinatorics;
import com.actelion.research.util.ArrayUtils;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.stream.IntStream;
/**
*
*
* CombinationGenerator
* Copyright: Actelion Ltd., Inc. All Rights Reserved
* This software is the proprietary information of Actelion Pharmaceuticals, Ltd.
* Use is subject to license terms.
* @author Modest von Korff
* @version 1.0
* Oct 12, 2012 MvK: Start implementation
*/
public class CombinationGenerator {
/**
* Get all possible index combinations, order independent.
* For a list containing the numbers from 0 to
* nObjects for arrays of the size 'sampleSize'.
* @param nObjects so many indices will be permuted.
* @param sampleSize Size of the array containing the permutations.
* @return
*/
public static List getAllOutOf(int nObjects, int sampleSize) {
List li = new ArrayList();
int [] arrCounters = new int [sampleSize];
if(nObjects==sampleSize){
int [] arr = new int [sampleSize];
for (int i = 0; i < arr.length; i++) {
arr[i]=i;
}
li.add(arr);
return li;
}else if (sampleSize==1){
for (int i = 0; i < nObjects; i++) {
int [] arr = new int [1];
arr[0]=i;
li.add(arr);
}
return li;
}else if (sampleSize>nObjects){
return null;
}
// init
for (int i = 0; i < arrCounters.length; i++) {
arrCounters[i]=i;
}
boolean proceed = true;
while(proceed){
int [] arr = new int [sampleSize];
for (int i = 0; i < sampleSize; i++) {
arr[i]=arrCounters[i];
}
li.add(arr);
int depth = sampleSize-1;
arrCounters[depth]++;
boolean counterFieldReset = false;
if(arrCounters[depth]>=nObjects){
counterFieldReset=true;
}
while(counterFieldReset){
counterFieldReset=false;
depth--;
arrCounters[depth]++;
for (int i = depth + 1; i < sampleSize; i++) {
arrCounters[i] = arrCounters[i-1]+1;
if(arrCounters[i] >= nObjects){
counterFieldReset=true;
}
}
if(depth==0)
break;
}
if(counterFieldReset)
proceed = false;
}
return li;
}
public static List getCombinations(List li){
int nCombinations = 1;
for (int[] arr : li) {
nCombinations *= arr.length;
}
List liComb = new ArrayList<>(nCombinations);
for (int i = 0; i < nCombinations; i++) {
int [] arrComb = new int[li.size()];
liComb.add(arrComb);
}
int nCombCol=1;
for (int col = 0; col < li.size(); col++) {
nCombCol *= li.get(col).length;
int nRepetitions = nCombinations / nCombCol;
int [] arr = li.get(col);
int indexArr = 0;
int row = 0;
while (row < nCombinations) {
for (int i = 0; i < nRepetitions; i++) {
int [] arrComb = liComb.get(row);
arrComb[col]=arr[indexArr];
row++;
}
indexArr++;
if(indexArr==arr.length) {
indexArr=0;
}
}
}
return liComb;
}
private static void swap(int[] input, int a, int b) {
int tmp = input[a];
input[a] = input[b];
input[b] = tmp;
}
/**
*
* @param elements
* @param n
* @return
*/
public static List getPermutations(int[] elements, int n){
List permutations = new ArrayList();
int[] indexes = new int[n];
int i = 0;
permutations.add(Arrays.copyOf(elements, elements.length));
while (i < n) {
if (indexes[i] < i) {
swap(elements, i % 2 == 0 ? 0: indexes[i], i);
permutations.add(Arrays.copyOf(elements, elements.length));
indexes[i]++;
i = 0;
}
else {
indexes[i] = 0;
i++;
}
}
return permutations;
}
/**
* https://en.wikipedia.org/wiki/Cartesian_product
* generates all possible combinations of elements from a list of lists
* @param
* @param lists
* @return
*/
public static List> cartesianProduct(List> lists) {
List> resultLists = new ArrayList>();
if (lists.size() == 0) {
resultLists.add(new ArrayList());
return resultLists;
} else {
List firstList = lists.get(0);
List> remainingLists = cartesianProduct(lists.subList(1, lists.size()));
for (T condition : firstList) {
for (List remainingList : remainingLists) {
ArrayList resultList = new ArrayList();
resultList.add(condition);
resultList.addAll(remainingList);
resultLists.add(resultList);
}
}
}
return resultLists;
}
public static BigInteger getFactorial (int n) {
BigInteger fact = BigInteger.ONE;
for (int i = n; i > 1; i--) {
fact = fact.multiply (new BigInteger (Integer.toString (i)));
}
return fact;
}
/**
* Calculate binomial coefficient or
* n choose k
*
* @param n
* @param k
* @return
*/
public static BigInteger getBinomialCoefficient(int n, int k){
BigInteger nFac = getFactorial(n);
BigInteger kFac = getFactorial(k);
BigInteger nMinus_k_Fac = getFactorial(n-k);
BigInteger dev = nMinus_k_Fac.multiply(kFac);
BigInteger bc = nFac.divide(dev);
return bc;
}
public static void main(String[] args) {
int l = 3;
int [] r = new int[l];
for (int i = 0; i < l; i++) {
r[i]=i;
}
List permutations = getPermutations(r,r.length);
for(int[] per : permutations) {
//System.out.println(Arrays.toString(per));
System.out.println(Arrays.toString(per));
}
System.out.println(permutations.size());
// int n = 12;
// int k= 3;
//
// BigInteger bc = getBinomialCoefficient(n,k);
//
// System.out.println(bc.toString());
}
// public static void main(String[] args) {
// List li = new ArrayList<>();
//
//// int [] a1 = {0,1};
//// int [] a2 = {0};
//// int [] a3 = {1,2};
// int [] a1 = {1,2,3,4,5,6,7,8};
// int [] a2 = {1,2,3};
// //int [] a3 = {5,6,7};
// //int [] a4 = {8};
//
// //li.add(a1);
// //li.add(a2);
//
//
// //li.add(a3);
// //li.add(a4);
//
// //List liComb = getCombinations(li);
// /*
// List liComb = getAllOutOf(9,3);
//
//
// li = new ArrayList<>();
// for(int[] l : liComb)
// //getPermutations(l,l.length);
// System.out.println(Arrays.toString(l));
// */
// int n = 3;
// int[] elements = IntStream.range(1,n).toArray();
// List liComb = getAllOutOf(7,n-1);
//
// for(int[] r : liComb) {
// List permutations = getPermutations(r,r.length);
// System.out.println("#####");
// //System.out.println(Arrays.toString(r));
// for(int[] per : permutations) {
// //System.out.println(Arrays.toString(per));
// int[] arr = new int[per.length+1];
// arr[0] = 0;
// IntStream.range(0, per.length).forEach(e -> {
// arr[e+1] = per[e]+1;});
// System.out.println(Arrays.toString(arr));
// }
// }
//
// }
// public static void main(String[] args) {
//
// int sizeList = 9;
//
// int sumCombinations=0;
// for (int i = 4; i < sizeList+1; i++) {
//
// List li = CombinationGenerator.getAllOutOf(sizeList, i);
//
// sumCombinations += li.size();
// for (int j = 0; j < li.size(); j++) {
// int [] a = li.get(j);
// System.out.println(ArrayUtils.toString(a));
//
// }
// }
//
// System.out.println("sum combinations " + sumCombinations);
//
//
//
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy