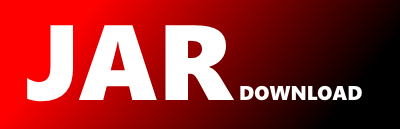
com.actelion.research.chem.phesaflex.EvaluableFlexibleOverlap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
package com.actelion.research.chem.phesaflex;
import java.util.Arrays;
import java.util.Map;
import com.actelion.research.chem.StereoMolecule;
import com.actelion.research.chem.forcefield.mmff.ForceFieldMMFF94;
import com.actelion.research.chem.optimization.Evaluable;
import com.actelion.research.chem.phesa.AtomicGaussian;
import com.actelion.research.chem.phesa.Gaussian3D;
import com.actelion.research.chem.phesa.MolecularVolume;
import com.actelion.research.chem.phesa.QuickMathCalculator;
import com.actelion.research.chem.phesa.VolumeGaussian;
import com.actelion.research.chem.phesa.PheSAAlignment;
import com.actelion.research.chem.phesa.pharmacophore.PPGaussian;
/**
* @author JW, Oktober 2019
* functionality for optimizing PheSA overlap (Pharmacophore+Shape) allowing for molecular flexibility
*/
public class EvaluableFlexibleOverlap implements Evaluable {
//private static final double SCALE = -250;
//private static final double DELTA = -0.01;
private static final double LAMBDA = 0.0625;
private double e0 = 0.0;
private StereoMolecule fitMol;
private PheSAAlignment shapeAlign;
private boolean[] isHydrogen;
private double[] v; //coordinates of the atoms
private double[][] precalcPow;
private double[] precalcExp;
private double oAA;
private double oAApp;
private ForceFieldMMFF94 ff;
private Map ffOptions;
private double ppWeight;
public EvaluableFlexibleOverlap(PheSAAlignment shapeAlign, StereoMolecule refMol, StereoMolecule fitMol, double ppWeight, boolean[] isHydrogen,double[] v, Map ffOptions) {
ForceFieldMMFF94.initialize(ForceFieldMMFF94.MMFF94SPLUS);
this.ffOptions = ffOptions;
ff = new ForceFieldMMFF94(fitMol, ForceFieldMMFF94.MMFF94SPLUS, this.ffOptions);
this.shapeAlign = shapeAlign;
this.fitMol = fitMol;
this.isHydrogen = isHydrogen;
this.ppWeight = ppWeight;
this.v = v;
for(int i=0;i0.0) {
totalOverlap += atomOverlap;
gradientPrefactor = atomOverlap*-2*refAt.getWidth()*fitAt.getWidth()/(refAt.getWidth()+fitAt.getWidth());
}
}
grad[3*a] += (2*xj-2*xi)*gradientPrefactor;
grad[3*a+1] += (2*yj-2*yi)*gradientPrefactor;
grad[3*a+2] += (2*zj-2*zi)*gradientPrefactor;
}
}
for(VolumeGaussian refVG:refMolGauss.getVolumeGaussians()){
double xi = refVG.getCenter().x;
double yi = refVG.getCenter().y;
double zi = refVG.getCenter().z;
for(AtomicGaussian fitAt:molGauss.getAtomicGaussians()){
int a = fitAt.getAtomId();
double atomOverlap = 0.0;
double xj = v[3*a];
double yj = v[3*a+1];
double zj = v[3*a+2];
double dx = xi-xj;
double dy = yi-yj;
double dz = zi-zj;
double Rij2 = dx*dx + dy*dy + dz*dz;
double alphaSum = refVG.getWidth() + fitAt.getWidth();
double gradientPrefactor=0.0;
if(Rij20.0) {
totalOverlap += atomOverlap;
gradientPrefactor = atomOverlap*-2*refVG.getWidth()*fitAt.getWidth()/(refVG.getWidth()+fitAt.getWidth());
}
}
grad[3*a] += (2*xj-2*xi)*gradientPrefactor;
grad[3*a+1] += (2*yj-2*yi)*gradientPrefactor;
grad[3*a+2] += (2*zj-2*zi)*gradientPrefactor;
}
}
return totalOverlap;
}
public double getFGValuePP(double[] grad) {
MolecularVolume molGauss = shapeAlign.getMolGauss();
MolecularVolume refMolGauss = shapeAlign.getRefMolGauss();
for(int i=0;i0.0) {
double sim = refPP.getSimilarity(fitPP);
atomOverlap *= sim;
totalOverlap += atomOverlap;
gradientPrefactor = atomOverlap*-2*refPP.getWidth()*fitPP.getWidth()/(refPP.getWidth()+fitPP.getWidth());
grad[3*a] += (2*xj-2*xi)*gradientPrefactor*sim;
grad[3*a+1] += (2*yj-2*yi)*gradientPrefactor*sim;
grad[3*a+2] += (2*zj-2*zi)*gradientPrefactor*sim;
fitPP.getPharmacophorePoint().getDirectionalityDerivativeCartesian(grad, v, fitPP.getPharmacophorePoint().getDirectionality(), sim); }
}
}
}
return totalOverlap;
}
public double getFGValueShapeSelf(double[] grad, MolecularVolume molGauss,boolean rigid) {
for(int i=0;i0.0) {
gradientPrefactor = atomOverlap*-2*refAt.getWidth()*fitAt.getWidth()/(refAt.getWidth()+fitAt.getWidth());
}
}
grad[3*b] += (2*xj-2*xi)*gradientPrefactor;
grad[3*b+1] += (2*yj-2*yi)*gradientPrefactor;
grad[3*b+2] += (2*zj-2*zi)*gradientPrefactor;
return atomOverlap;
}
public double getFGValueSelfPP(double[] grad, MolecularVolume molVol,boolean rigid) {
double xi,yi,zi,xj,yj,zj;
for(int i=0;i0.0) {
double sim = refPP.getSimilarity(fitPP);
atomOverlap *= sim;
totalOverlap += atomOverlap;
gradientPrefactor = atomOverlap*-2*refPP.getWidth()*fitPP.getWidth()/(refPP.getWidth()+fitPP.getWidth());
grad[3*a] += (2*xj-2*xi)*gradientPrefactor*sim;
grad[3*a+1] += (2*yj-2*yi)*gradientPrefactor*sim;
grad[3*a+2] += (2*zj-2*zi)*gradientPrefactor*sim;
fitPP.getPharmacophorePoint().getDirectionalityDerivativeCartesian(grad, v, fitPP.getPharmacophorePoint().getDirectionality(), sim); }
}
}
}
return totalOverlap;
}
@Override
public EvaluableFlexibleOverlap clone() {
return new EvaluableFlexibleOverlap(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy