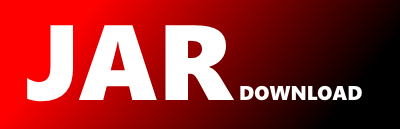
com.actelion.research.gui.swing.SwingDrawContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openchemlib Show documentation
Show all versions of openchemlib Show documentation
Open Source Chemistry Library
package com.actelion.research.gui.swing;
import com.actelion.research.gui.LookAndFeelHelper;
import com.actelion.research.gui.generic.GenericDrawContext;
import com.actelion.research.gui.generic.GenericImage;
import com.actelion.research.gui.generic.GenericPolygon;
import com.actelion.research.gui.generic.GenericRectangle;
import javax.swing.*;
import java.awt.*;
import java.awt.font.GlyphVector;
import java.awt.geom.Ellipse2D;
import java.awt.geom.GeneralPath;
import java.awt.geom.Line2D;
import java.awt.geom.Rectangle2D;
public class SwingDrawContext implements GenericDrawContext {
private static boolean isMac = (System.getProperty("os.name").toLowerCase().indexOf("mac") >= 0);
private Graphics2D mG;
public SwingDrawContext(Graphics2D g) {
mG= g;
}
@Override
public int getFontSize() {
return mG.getFont().getSize();
}
@Override
public void setFont(int size, boolean isBold, boolean isItalic) {
int style = (isBold ? Font.BOLD : 0) + (isItalic ? Font.ITALIC : 0);
mG.setFont(mG.getFont().deriveFont(style, (float)size));
}
@Override
public void drawLine(double x1, double y1, double x2, double y2) {
// Lines on OSX are shifted left and down when drawn in Graphics2D by 0.5. We must compensate.
if (isMac)
mG.draw(new Line2D.Double(x1-0.5, y1-0.5, x2-0.5, y2-0.5));
else
mG.draw(new Line2D.Double(x1, y1, x2, y2));
}
@Override
public void drawDottedLine(double x1, double y1, double x2, double y2) {
Stroke stroke = mG.getStroke();
float width = ((BasicStroke)stroke).getLineWidth();
mG.setStroke(new BasicStroke(width, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND, width, new float[] {3.0f*width}, 0f));
drawLine(x1, y1, x2, y2);
mG.setStroke(stroke);
}
@Override
public void drawRectangle(double x, double y, double w, double h) {
mG.draw(new Rectangle2D.Double(x, y, w, h));
}
@Override
public void fillRectangle(double x, double y, double w, double h) {
mG.fill(new Rectangle2D.Double(x, y, w, h));
}
@Override
public void drawCircle(double x, double y, double d) {
if (isMac)
mG.draw(new Ellipse2D.Double(x-0.5f, y-0.5f, d, d));
else
mG.draw(new Ellipse2D.Double(x, y, d, d));
}
@Override
public void fillCircle(double x, double y, double d) {
if (isMac)
mG.fill(new Ellipse2D.Double(x-0.5f, y-0.5f, d, d));
else
mG.fill(new Ellipse2D.Double(x, y, d, d));
}
@Override
public void drawPolygon(GenericPolygon p) {
GeneralPath polygon = new GeneralPath(GeneralPath.WIND_NON_ZERO, p.getSize());
if (isMac) {
polygon.moveTo((float)p.getX(0)-0.5f, (float)p.getY(0)-0.5f);
for (int i = 1; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy