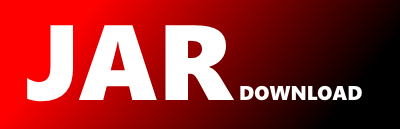
com.ats.AtsSingleton Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats;
import java.io.File;
import java.net.URI;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import org.graalvm.polyglot.Context;
import org.graalvm.polyglot.Context.Builder;
import org.graalvm.polyglot.Engine;
import org.graalvm.polyglot.io.IOAccess;
import com.ats.driver.ApplicationProperties;
import com.ats.driver.AtsManager;
import com.ats.driver.AtsProxy;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.TestBound;
import com.ats.executor.channels.Channel;
import com.ats.executor.channels.ChannelManager;
import com.ats.executor.drivers.DriverInfo;
import com.ats.executor.drivers.DriverInfoLocal;
import com.ats.executor.drivers.IDriverInfo;
import com.ats.executor.scripts.AtsCallSubscriptJavascript;
import com.ats.executor.scripts.AtsCallSubscriptPython;
import com.ats.generator.variables.Variable;
import com.ats.script.Project;
import com.ats.script.actions.ActionChannelStart;
import com.ats.script.actions.neoload.ActionNeoload;
import com.ats.tools.logger.ExecutionLogger;
import com.ats.tools.wait.IWaitGuiReady;
public class AtsSingleton {
private static AtsSingleton instance;
public static AtsSingleton getInstance() {
if (instance == null) {
synchronized (AtsSingleton.class) {
if (instance == null) {
instance = new AtsSingleton();
}
}
}
return instance;
}
private AtsSingleton(){}
//-----------------------------------------------------------------------------------------------------------
// Instance variables
//-----------------------------------------------------------------------------------------------------------
private IDriverInfo systemDriver;
private ChannelManager channelManager = new ChannelManager();
private List scriptCallTree = new ArrayList<>();
//------------------------------------------------------------------------------------------
// Shared values
//------------------------------------------------------------------------------------------
private String jsonSuitesFile;
public void setJsonSuitesFilePath(String value) {
this.jsonSuitesFile = value;
}
public File getJsonSuitesFile() {
if (jsonSuitesFile != null && !jsonSuitesFile.isBlank()) {
return Paths.get(jsonSuitesFile).toFile();
}
return null;
}
private String atsOutputFolder;
public void setAtsOutputFolder(String value) {
this.atsOutputFolder = value;
}
public String getAtsOutputFolder() {
return atsOutputFolder;
}
private Builder pythonBuilder;
public Builder getPythonBuilder() {
if(pythonBuilder == null) {
final Engine engine = Engine.newBuilder()
.option("engine.WarnInterpreterOnly", "false")
.build();
pythonBuilder = Context.
newBuilder(AtsCallSubscriptPython.PYTHON_LANGUAGE).
engine(engine).
allowIO(IOAccess.ALL).
allowAllAccess(true);
}
return pythonBuilder;
}
private Context javaScriptContext;
public Context getJavaScriptContext() {
if(javaScriptContext == null) {
final Engine engine = Engine.newBuilder()
.option("engine.WarnInterpreterOnly", "false")
.build();
javaScriptContext = Context.
newBuilder(AtsCallSubscriptJavascript.JS_LANGUAGE).
engine(engine).build();
}
return javaScriptContext;
}
//------------------------------------------------------------------------------------------
// Logs level
//------------------------------------------------------------------------------------------
private String logLevel = ExecutionLogger.SILENT;
public void setLoglevel(String value) {
this.logLevel = value;
}
public String getLogLevel() {
return logLevel;
}
//------------------------------------------------------------------------------------------
// Ats management
//------------------------------------------------------------------------------------------
private AtsManager ats = new AtsManager();
public String getAtsKey() {
return ats.getAtsKey();
}
public String getAtsError() {
return ats.getError();
}
public IWaitGuiReady getWaitGuiReady() {
return ats.getWaitGuiReady();
}
public int getMaxTrySearch() {
return ats.getMaxTrySearch();
}
public int getMaxTryScrollSearch() {
return ats.getMaxTryScrollSearch();
}
public ApplicationProperties getApplicationProperties(String appName) {
return ats.getApplicationProperties(appName);
}
public int getMaxTryInteractable() {
return ats.getMaxTryInteractable();
}
//------------------------------------------------------------------------------------------
// System driver management
//------------------------------------------------------------------------------------------
public void setSystemDriver(IDriverInfo sysDriver) {
if(sysDriver == null && systemDriver != null) {
systemDriver.close();
}
systemDriver = sysDriver;
}
private boolean init = false;
public IDriverInfo getSystemDriver(ActionStatus status, ActionTestScript script) {
if(systemDriver == null || !systemDriver.isAlive()) {
IDriverInfo driver = null;
status.setError(0, "no remote agent defined");
for(URI sysUri : ats.getSystemDriverUris()) {
script.getLogger().sendInfo("try to connect to ats-agent", sysUri.toString());
driver = new DriverInfo(status, Channel.DESKTOP, sysUri, script);
if(status.isPassed()) {
break;
}
}
if(status.isPassed()) { //Remote driver is listening
systemDriver = driver;
}else {
driver = new DriverInfoLocal(
status,
ats.getDriverPath(),
getLogLevel(),
script);
if(status.isPassed()) {
systemDriver = driver;
}else {
return systemDriver;
}
}
}
if(!init) {
init = true;
}
return systemDriver;
}
public IDriverInfo relaunchSystemDriver(ActionStatus status, ActionTestScript script) {
closeSystemDriver();
return getSystemDriver(status, script);
}
public boolean closeSystemDriver() {
if(systemDriver != null) {
systemDriver.close();
systemDriver = null;
return true;
}
return false;
}
//------------------------------------------------------------------------------------------
// Channels management
//------------------------------------------------------------------------------------------
public String getSystemValue(String name) {
final Channel cnl = channelManager.getCurrentChannel();
if (cnl != null) {
return cnl.getSystemValue(name);
}
return Channel.systemValueObj(name);
}
public void closeChannels(ActionStatus status, final List channels) {
while(channels.size() > 0) {
closeChannel(status, channels.remove(0));
}
channelManager.switchToLastChannel(status);
channelManager.closeMobileDriver();
}
public boolean closeChannel(ActionStatus status, String name) {
return channelManager.closeChannel(status, name);
}
public void channelClosed(ActionStatus status, Channel channel) {
channelManager.channelClosed(status, channel);
}
public boolean isDesktopChannel() {
return channelManager.getCurrentChannel().isDesktop();
}
public boolean switchChannel(ActionStatus status, String name) {
return channelManager.switchChannel(status, name);
}
public Channel getChannel(String name) {
return channelManager.getChannel(name);
}
public Channel getCurrentChannel() {
return channelManager.getCurrentChannel();
}
public Channel getCurrentChannel(ActionTestScript script) {
channelManager.setMainScript(script);
return channelManager.getCurrentChannel();
}
public void startChannel(ActionChannelStart actionChannelStart, ActionTestScript ts, String testName, int testLine) {
channelManager.setMainScript(ts);
channelManager.startChannel(actionChannelStart, testName, testLine);
}
public int getMaxTry() {
return channelManager.getMaxTry();
}
public int getMaxTryScroll() {
return channelManager.getMaxTryScroll();
}
public void sleep(int delay) {
channelManager.getCurrentChannel().sleep(delay);
}
public void neoloadAction(ActionNeoload actionNeoload, String testName, int testLine) {
channelManager.getCurrentChannel().neoloadAction(actionNeoload, testName, testLine);
}
//------------------------------------------------------------------------------------------
// Callscripts management
//------------------------------------------------------------------------------------------
public void addCalledScript(ActionTestScript sc, String name) {
final Optional opScript = scriptCallTree.stream().filter(a -> a.getCanonicalName().equals(name)).findFirst();
if (opScript.isPresent()) {
scriptCallTree.remove(opScript.get());
}
scriptCallTree.add(sc);
}
public String getGlobalVariableValue(String varPath) {
final int lastDot = varPath.lastIndexOf(".");
if (lastDot > -1) {
final String scriptPath = varPath.substring(0, lastDot);
final Optional sc = scriptCallTree.stream().filter(a -> a.getCanonicalName().equals(scriptPath)).findFirst();
if (sc.isPresent()) {
final Variable scVar = sc.get().getVariable(varPath.substring(lastDot + 1));
if (scVar != null) {
return scVar.getCalculatedValue();
}
}
}
return null;
}
public Map getGlobalVariables() {
final HashMap result = new HashMap<>();
scriptCallTree.stream().forEach(s -> addGlobalVar(result, s));
return result;
}
private static void addGlobalVar(HashMap map, ActionTestScript ts) {
final String scriptName = ts.getTestName();
ts.getVariables().forEach(v -> map.put(scriptName + "." + v.getName(), v.getCalculatedValue()));
}
private HashMap projectVariables = new HashMap<>();
public void setProjectPath(String projectPath) {
File projectFile = new File(projectPath);
Project projectData = Project.getProjectData(projectFile, null, null);
projectVariables = projectData.getVariables();
}
public String getProjectVariableValue(String varName) {
return projectVariables.getOrDefault(varName, "");
}
public TestBound getApplicationBound() {
return ats.getApplicationBound();
}
public int getMaxTryProperty() {
return ats.getMaxTryProperty();
}
public int getMaxTryWebservice() {
return ats.getMaxTryWebservice();
}
public int getWebServiceTimeOut() {
return ats.getWebServiceTimeOut();
}
public String getNeoloadDesignApi() {
return ats.getNeoloadDesignApi();
}
public AtsProxy getNeoloadProxy() {
return ats.getNeoloadProxy();
}
public AtsProxy getProxy() {
return ats.getProxy();
}
public AtsManager getAts() {
return ats;
}
public int getMaxTryImageRecognition() {
return ats.getMaxTryImageRecognition();
}
public Class loadTestScriptClass(ActionStatus status, String scriptName) {
return ats.loadTestScriptClass(status, scriptName);
}
public int getRegexTimeOut() {
return ats.getRegexTimeOut();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy