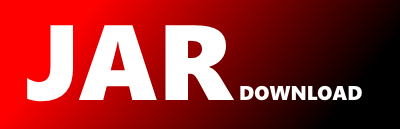
com.ats.driver.ApplicationProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.driver;
import com.ats.executor.drivers.DriverManager;
import com.ats.executor.drivers.desktop.SystemDriver;
import com.fasterxml.jackson.databind.JsonNode;
import com.google.common.collect.ObjectArrays;
public class ApplicationProperties {
public final static int BROWSER_TYPE = 0;
public final static int DESKTOP_TYPE = 1;
public final static int MOBILE_TYPE = 2;
public final static int API_TYPE = 3;
public final static int SAP_TYPE = 4;
private int type = BROWSER_TYPE;
private String name = DriverManager.CHROME_BROWSER;
private String driver;
private String uri;
private int wait = -1;
private int debugPort = -1;
private int check = -1;
private String lang;
private String userDataDir;
private String title;
private String[] options;
private String[] excludedOptions;
private String authentication;
public ApplicationProperties(String name) {
this.name = name;
}
public ApplicationProperties(String name, String connexion, String client, String authentication, String lang) {
this(name);
this.type = SAP_TYPE;
this.uri = connexion + ":" + client + ":" + lang;
this.authentication = authentication;
this.lang = lang;
}
public ApplicationProperties(int type, String name, String driver, String uri, int wait, int check, String lang, String userDataDir, String title, String[] options, String[] excludedOptions, int debugPort) {
this(name);
this.type = type;
this.driver = driver;
this.uri = uri;
this.wait = wait;
this.check = check;
this.lang = lang;
this.userDataDir = userDataDir;
this.title = title;
this.options = options;
this.excludedOptions = excludedOptions;
this.debugPort = debugPort;
}
public static String getUserDataPath(String userDataDir, String browserName, SystemDriver systemDriver) {
if(userDataDir != null) {
final JsonNode json = systemDriver.getUserFolder(userDataDir, browserName);
if(json.has("userDataPath")) {
return json.get("userDataPath").asText();
}
}
return null;
}
public boolean isWeb() {
return type == BROWSER_TYPE;
}
public boolean isDesktop() {
return type == DESKTOP_TYPE;
}
public boolean isMobile() {
return type == MOBILE_TYPE;
}
public boolean isApi() {
return type == API_TYPE;
}
public boolean isSap() {
return type == SAP_TYPE;
}
public String getName() {
return name;
}
public String getDriver() {
return driver;
}
public String getUri() {
return uri;
}
public int getWait() {
return wait;
}
public int getDebugPort() {
return debugPort;
}
public int getCheck() {
return check;
}
public String getLang() {
return lang;
}
public String getUserDataDir() {
return userDataDir;
}
public String getTitle() {
return title;
}
public String[] getOptions() {
return options;
}
public void addExcludedOptions(String opt) {
final int len = getExcludedOptions().length;
excludedOptions = new String[len+1];
excludedOptions[len] = opt;
}
public String[] getExcludedOptions() {
if(excludedOptions == null) {
return new String[0];
}
return excludedOptions;
}
public String[] getExcludedOptions(String logOption) {
final String[] data = new String[] {logOption};
if(excludedOptions == null) {
return data;
}
return ObjectArrays.concat(data, excludedOptions, String.class);
}
public String getAuthentication() {
return authentication;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy