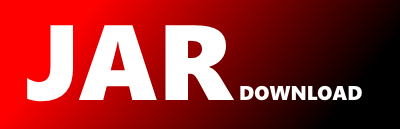
com.ats.element.AtsElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.element;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import org.openqa.selenium.remote.RemoteWebElement;
import com.ats.executor.drivers.desktop.DesktopData;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.NullNode;
@SuppressWarnings("unchecked")
public class AtsElement extends AtsBaseElement {
private static final String IFRAME = "IFRAME";
private static final String FRAME = "FRAME";
public static final String SELECT = "SELECT";
public static final String OPTION = "OPTION";
public final static String BODY = "BODY";
public final static String INPUT = "INPUT";
private RemoteWebElement element;
private boolean numeric = false;
private boolean password = false;
private int numChildren = 0;
private Double screenX = 0D;
private Double screenY = 0D;
private Double boundX = 0D;
private Double boundY = 0D;
private boolean visible = true;
private boolean clickable = true;
private boolean shadowRoot = false;
private ArrayList children;
public static boolean checkIframe(String value) {
return IFRAME.equalsIgnoreCase(value) || FRAME.equalsIgnoreCase(value);
}
public static boolean checkBody(String value) {
return BODY.equalsIgnoreCase(value);
}
public static boolean checkInput(String value) {
return INPUT.equalsIgnoreCase(value);
}
public AtsElement() {}
public AtsElement(JsonNode node){
// super(node);
jsonDeserialize(node);
}
public AtsElement(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy