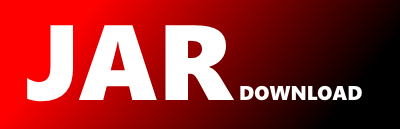
com.ats.element.JsonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.element;
import java.util.Base64;
import java.util.Map;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
public class JsonUtils {
public static T getJsonValue(JsonNode node, String key, T defaultValue, Class clazz) {
if (node.has(key)) {
JsonNode valueNode = node.get(key);
if (clazz == String.class && valueNode.isTextual()) return clazz.cast(valueNode.asText());
if (clazz == Integer.class && valueNode.isInt()) return clazz.cast(valueNode.asInt());
if (clazz == Double.class && valueNode.isDouble()) return clazz.cast(valueNode.asDouble());
if (clazz == Boolean.class && valueNode.isBoolean()) return clazz.cast(valueNode.asBoolean());
}
return defaultValue;
}
public static byte[] getBase64Image(JsonNode node, String key) {
String base64Image = node.get(key).asText();
return Base64.getDecoder().decode(base64Image);
}
public static ObjectNode createPostData(ObjectMapper mapper, Map values) {
final ObjectNode postData = mapper.createObjectNode();
final ObjectNode valueNode = mapper.createObjectNode();
for (Map.Entry entry : values.entrySet()) {
valueNode.putPOJO(entry.getKey(), entry.getValue());
}
postData.set("value", valueNode);
return postData;
}
public static ObjectNode createPostData(Object pojo) {
final ObjectMapper mapper = new ObjectMapper();
final ObjectNode postData = mapper.createObjectNode();
postData.set("value", mapper.valueToTree(pojo));
return postData;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy