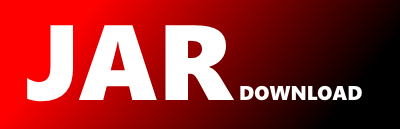
com.ats.element.SearchedElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.element;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.StringJoiner;
import java.util.function.Predicate;
import java.util.regex.Matcher;
import org.openqa.selenium.remote.RemoteWebElement;
import com.ats.element.test.TestElementRecord;
import com.ats.executor.ActionTestScript;
import com.ats.executor.drivers.engines.IDriverEngine;
import com.ats.generator.variables.CalculatedProperty;
import com.ats.generator.variables.CalculatedValue;
import com.ats.script.Project;
import com.ats.script.Script;
import com.ats.tools.Utils;
public class SearchedElement {
public static final String WILD_CHAR = "*";
private static final String DIALOG = "DIALOG";
private static final String SYSCOMP = "SYSCOMP";
public static final String IMAGE_TAG = "@IMAGE";
public static final String RECORD_TAG = "@RECORD";
public static final String PARAM_ELEMENT = "@PARAM_ELEMENT";
public static final String INDEX_PROPERTY = "@index";
public static final String SHADOW_ROOT = "@shadow-root";
private String tag = WILD_CHAR;
private SearchedElement parent;
private boolean loadAttributes = false;
private String selector = "";
private CalculatedValue index;
private boolean shadowRoot = false;
private ParameterElement parameterElement;
private ArrayList criterias;
private String[] attributes = new String[0];
private String[] attributesValues = new String[0];
private byte[] image;
public SearchedElement() {} // default constructor
public SearchedElement(String tag) {
this.tag = tag;
this.criterias = new ArrayList<>();
}
public SearchedElement(Script script, ArrayList elements) {
final String value = elements.remove(0);
final Matcher objectMatcher = Script.OBJECT_PATTERN.matcher(value);
setCriterias(new ArrayList<>());
index = new CalculatedValue(script, "0");
if (objectMatcher.find()) {
if(objectMatcher.groupCount() >= 1){
setTag(objectMatcher.group(1).trim());
if(objectMatcher.groupCount() >= 2){
Arrays.stream(objectMatcher.group(2).split(",")).map(String::trim).forEach(s -> addCriteria(script, s));
}
} else {
setTag(value.trim());
}
} else {
setTag(value.trim());
}
if(!elements.isEmpty()){
this.setParent(new SearchedElement(script, elements));
}
}
public SearchedElement(CalculatedValue index, boolean shadow, String tag, CalculatedProperty[] properties) {
this(null, index, shadow, tag, properties);
}
public SearchedElement(SearchedElement parent, CalculatedValue index, boolean shadow, String tag, CalculatedProperty[] properties) {
setParent(parent);
setIndex(index);
setShadowRoot(shadow);
setTag(tag);
setCriterias(Arrays.asList(properties));
}
public SearchedElement(SearchedElement parent, CalculatedValue index, boolean shadow, String tag) {
this(parent, index, shadow, tag, new CalculatedProperty[0]);
}
public void dispose() {
if(parent != null) {
parent.dispose();
}
if(criterias != null) {
while(!criterias.isEmpty()) {
final CalculatedProperty prop = criterias.remove(0);
prop.dispose();
}
}
}
public RemoteWebElement getStartElement(IDriverEngine engine) {
if(parent == null) {
if(parameterElement != null) {
return parameterElement.getRemoteWebElement(engine.getAtsRemoteDriver());
}
return null;
}else {
return parent.getStartElement(engine);
}
}
/*public List loadParamElement(IDriverEngine engine) {
return List.of(engine.getParamElement(getParameterElement()));
}*/
private void addCriteria(Script script, String data){
if(data.startsWith(INDEX_PROPERTY)) {
final String[] value = data.split("=");
if (value.length > 1) {
index = new CalculatedValue(script, value[1].trim());
}
}else if(SHADOW_ROOT.equals(data)) {
shadowRoot = true;
}else{
criterias.add(new CalculatedProperty(script, data));
}
}
public int[] updateRecordSelector() {
int duration = 3;
int device = 0;
final StringJoiner sb = new StringJoiner(",");
sb.add(SearchedElement.RECORD_TAG);
for(CalculatedProperty prop : getCriterias()) {
if(TestElementRecord.DEVICE.equals(prop.getName())) {
device = Utils.string2Int(prop.getValue().getCalculated());
sb.add(TestElementRecord.DEVICE + "=\"" + String.valueOf(device) + "\"");
}else if(TestElementRecord.DURATION.equals(prop.getName())) {
duration = Utils.string2Int(prop.getValue().getCalculated());
sb.add(TestElementRecord.DURATION + "=\"" + String.valueOf(duration) + "\"");
}
}
selector = sb.toString();
return new int[] {duration, device};
}
public Predicate getPredicate() {
final StringBuilder sb = new StringBuilder(getTag());
final int criteriasCount = getCriterias().size();
attributes = new String[criteriasCount];
attributesValues = new String[criteriasCount];
Predicate fullPredicate = Objects::nonNull;
StringJoiner props = new StringJoiner(",");
for (int i = 0; i < criteriasCount; i++) {
final CalculatedProperty property = getCriterias().get(i);
final String propertyName = property.getName();
final String propertyValue = property.getValue().getCalculated();
props.add(propertyName + " = " + propertyValue);
fullPredicate = property.getPredicate(fullPredicate);
attributes[i] = propertyName;
if (property.isRegexp()) {
attributesValues[i] = propertyName;
} else {
attributesValues[i] = propertyName + "\t" + propertyValue;
}
}
sb.append(" [").append(props.toString()).append("]");
if(getIndex().getCalculatedInteger() != 0) {
sb.append("[").append(getIndex().getCalculatedInteger()).append("]");
}
selector = sb.toString();
return fullPredicate;
}
public String[] getAttributes() {
return attributes;
}
public String[] getAttributesValues() {
return attributesValues;
}
public String getSelector() {
return selector;
}
//----------------------------------------------------------------------------------------------------------------
// image management
//----------------------------------------------------------------------------------------------------------------
public void setImage(byte[] value) {
this.image = value;
}
public byte[] getImage() {
if(image == null) {
final CalculatedProperty prop = criterias.stream().filter(c -> "source".equals(c.getName())).findFirst().orElse(null);
if(prop != null) {
final String imagePath = prop.getValue().getCalculated();
URL imageUrl = null;
if(imagePath.startsWith("http://") || imagePath.startsWith("https://") || imagePath.startsWith("file://")) {
try {
imageUrl = new URI(imagePath).toURL();
} catch (MalformedURLException | URISyntaxException ignored) {}
}else {
final String relativePath = Project.ASSETS_FOLDER + "/" + Project.RESOURCES_FOLDER + "/" + Project.IMAGES_FOLDER + "/" + imagePath;
imageUrl = getClass().getClassLoader().getResource(relativePath);
}
if(imageUrl != null) {
image = Utils.loadImage(imageUrl);
}
}
}
return image;
}
//----------------------------------------------------------------------------------------------------------------
// types
//----------------------------------------------------------------------------------------------------------------
public boolean isParamElement() {
return PARAM_ELEMENT.equals(tag.toUpperCase());
}
public boolean isImageSearch() {
return IMAGE_TAG.equals(tag.toUpperCase());
}
public boolean isRecordSearch() {
return RECORD_TAG.equals(tag.toUpperCase());
}
public boolean isDialog() {
return DIALOG.equals(tag.toUpperCase());
}
public boolean isScrollable() {
return tag.equals("RecyclerView") || tag.equals("Table") || tag.equals("CollectionView") || tag.equals("WebView");
}
public boolean isSysComp() {
if(parent != null) {
return parent.isSysComp();
}
return SYSCOMP.equals(tag.toUpperCase());
}
//----------------------------------------------------------------------------------------------------------------
//
//----------------------------------------------------------------------------------------------------------------
public String getJavaCode() {
final StringBuilder codeBuilder = new StringBuilder(ActionTestScript.JAVA_ELEMENT_FUNCTION_NAME);
codeBuilder.append("(");
if(parent != null){
codeBuilder.append(parent.getJavaCode());
codeBuilder.append(", ");
}
codeBuilder.append(index.getJavaCode());
codeBuilder.append(", ");
codeBuilder.append(shadowRoot);
codeBuilder.append(", \"");
codeBuilder.append(getTag());
if (criterias != null && !criterias.isEmpty()) {
codeBuilder.append("\", ");
final StringJoiner joiner = new StringJoiner(", ");
for (CalculatedProperty criteria : criterias){
joiner.add(criteria.getJavaCode());
}
codeBuilder.append(joiner);
}else{
codeBuilder.append("\"");
}
codeBuilder.append(")");
return codeBuilder.toString();
}
public ArrayList getKeywords() {
final ArrayList keywords = new ArrayList<>();
keywords.add(tag);
for (CalculatedProperty crit : criterias) {
keywords.addAll(crit.getKeywords());
}
if(parent != null) {
keywords.addAll(parent.getKeywords());
}
return keywords;
}
public String getAtsLearningCode() {
final StringBuilder sb = new StringBuilder(getTag()).append(" [");
final StringJoiner crits = new StringJoiner(", ");
for(CalculatedProperty prop : getCriterias()) {
crits.add(prop.getAtsLearningCode());
}
sb.append(crits).append("]");
return sb.toString();
}
public String getAtsLearning() {
final StringJoiner stack = new StringJoiner(" -> ");
stack.add(getAtsLearningCode());
SearchedElement p = parent;
while (p != null) {
stack.add(p.getAtsLearningCode());
p = p.parent;
}
return stack.toString();
}
//----------------------------------------------------------------------------------------------------------------------
// Getter and setter for serialization
//----------------------------------------------------------------------------------------------------------------------
public ParameterElement getParameterElement() {
return parameterElement;
}
public void setParameterElement(ParameterElement value) {
parameterElement = value;
}
public boolean isShadowRoot() {
return shadowRoot;
}
public void setShadowRoot(boolean shadowRoot) {
this.shadowRoot = shadowRoot;
}
public boolean getLoadAttributes() {
return loadAttributes;
}
public void setLoadAttributes(boolean value) {
this.loadAttributes = value;
}
public SearchedElement getParent() {
return parent;
}
public void setParent(SearchedElement value) {
this.parent = value;
}
public String getTag() {
return tag;
}
public void setTag(String tag) {
this.tag = tag;
}
public CalculatedValue getIndex() { return index; }
public void setIndex(CalculatedValue value) {
this.index = value;
}
public List getCriterias() {
return criterias;
}
public void setCriterias(List value) {
this.criterias = new ArrayList(value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy