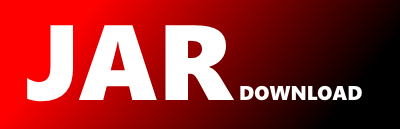
com.ats.element.test.TestElementRoot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.element.test;
import java.util.ArrayList;
import java.util.Base64;
import java.util.function.Predicate;
import com.ats.element.FoundElement;
import com.ats.element.SearchedElement;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.channels.Channel;
import com.ats.executor.drivers.engines.SapDriverEngine;
import com.ats.generator.objects.MouseDirection;
import com.ats.generator.variables.CalculatedProperty;
import com.ats.generator.variables.CalculatedValue;
public class TestElementRoot extends TestElement {
private final static String SOURCE = "source";
private final static String VERSION = "version";
private final static String RECTANGLE = "rectangle";
private final static String TITLE = "title";
private final static String URL = "url";
private final static String PROCESS_ID = "process-id";
private final static String SCREEN_SHOT = "screenshot";
private final static String WINDOWS = "windows";
private final static String COOKIES = "cookies";
private final static String HEADERS = "headers";
private final static String CURRENT_HANDLE = "current-handle";
public TestElementRoot() {
}
public TestElementRoot(ActionTestScript script, Channel channel, Predicate predicate) {
super(script, channel, predicate, 0);
}
@Override
protected void startSearch(boolean sysComp, SearchedElement searchedElement) {
//do nothing
}
@Override
public String enterText(ActionStatus status, CalculatedValue text, ActionTestScript script, int waitChar) {
if (engine instanceof SapDriverEngine) {
engine.sendTextData(status, this, text.getCalculatedText(script, false), waitChar, script.getTopScript());
return "";
}
return channel.rootKeys(status, text);
}
@Override
public Object executeScript(ActionStatus status, String script, boolean returnValue) {
return engine.executeJavaScript(status, script, returnValue);
}
@Override
public void mouseWheel(int delta) {
engine.scroll(delta);
channel.progressiveWait(1);
}
@Override
public void over(ActionStatus status, MouseDirection position, boolean desktopDragDrop, int offsetX, int offsetY) {
// do nothing, this is the root, no need to scroll over the root element
channel.setWindowToFront();
channel.refreshLocation();
super.over(status, position, desktopDragDrop, offsetX, offsetY);
}
@Override
public String getAttribute(ActionStatus status, String name) {
switch (name.toLowerCase()) {
case SOURCE:
return engine.getSource();
case RECTANGLE:
return channel.getBoundDimension();
case CLIENT_WIDTH:
return channel.getDimension().getWidth().toString();
case CLIENT_HEIGTH:
return channel.getDimension().getHeight().toString();
case SCREEN_SHOT:
return Base64.getEncoder().encodeToString(channel.getScreenShot());
case VERSION:
return channel.getApplicationVersion();
case PROCESS_ID:
return String.valueOf(channel.getProcessId());
case TITLE:
return engine.getTitle();
case URL:
return engine.getUrl();
case WINDOWS:
return String.valueOf(engine.getNumWindows());
case COOKIES:
return engine.getCookies();
case HEADERS:
return engine.getHeaders(status);
case CURRENT_HANDLE:
return engine.getCurrentHandle();
default:
reloadFoundElements();
return engine.getAttribute(status, getFoundElement(), name, 5);
}
}
@Override
public CalculatedProperty[] getAttributes(boolean reload) {
final ArrayList attributes = new ArrayList<>();
attributes.add(new CalculatedProperty(SOURCE, "[...]"));
attributes.add(new CalculatedProperty(VERSION, channel.getApplicationVersion()));
attributes.add(new CalculatedProperty(RECTANGLE, channel.getBoundDimension()));
attributes.add(new CalculatedProperty(PROCESS_ID, String.valueOf(channel.getProcessId())));
attributes.add(new CalculatedProperty(TITLE, engine.getTitle()));
attributes.add(new CalculatedProperty(URL, engine.getUrl()));
attributes.add(new CalculatedProperty(WINDOWS, String.valueOf(engine.getNumWindows())));
attributes.add(new CalculatedProperty(COOKIES, engine.getCookies()));
attributes.add(new CalculatedProperty(HEADERS, engine.getHeaders(new ActionStatus())));
attributes.add(new CalculatedProperty(CURRENT_HANDLE, engine.getCurrentHandle()));
return attributes.toArray(new CalculatedProperty[attributes.size()]);
}
@Override
public CalculatedProperty[] getCssAttributes() {
reloadFoundElements();
return super.getCssAttributes();
}
@Override
public CalculatedProperty[] getHtmlAttributes() {
return getAttributes(true);
}
@Override
public FoundElement getFoundElement() {
return new FoundElement(channel);
}
@Override
public Double[] getBound() {
return new Double[]{channel.getSubDimension().getX(), channel.getSubDimension().getY(), channel.getSubDimension().getWidth(), channel.getSubDimension().getHeight()};
}
@Override
public int getElementsCount() {
return 1;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy