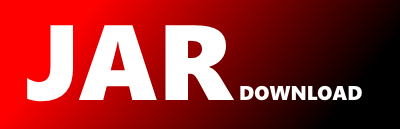
com.ats.executor.channels.Channel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.channels;
import java.io.FileWriter;
import java.io.IOException;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.ats.recorder.TestError;
import org.openqa.selenium.Proxy;
import org.openqa.selenium.WebElement;
import com.ats.AtsSingleton;
import com.ats.driver.AtsManager;
import com.ats.element.DialogBox;
import com.ats.element.FoundElement;
import com.ats.element.test.TestElement;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.ScriptStatus;
import com.ats.executor.TestBound;
import com.ats.executor.drivers.DriverManager;
import com.ats.executor.drivers.desktop.DesktopData;
import com.ats.executor.drivers.desktop.DesktopResponse;
import com.ats.executor.drivers.desktop.DesktopWindow;
import com.ats.executor.drivers.desktop.SystemDriver;
import com.ats.executor.drivers.engines.IDriverEngine;
import com.ats.executor.drivers.engines.SapDriverEngine;
import com.ats.executor.drivers.engines.SystemDriverEngine;
import com.ats.executor.drivers.engines.WebDriverEngine;
import com.ats.generator.ATS;
import com.ats.generator.objects.BoundData;
import com.ats.generator.objects.MouseDirection;
import com.ats.generator.objects.MouseDirectionData;
import com.ats.generator.variables.CalculatedProperty;
import com.ats.generator.variables.CalculatedValue;
import com.ats.recorder.IVisualRecorder;
import com.ats.recorder.TestSummary;
import com.ats.recorder.VisualRecorderNull;
import com.ats.script.ScriptHeader;
import com.ats.script.actions.Action;
import com.ats.script.actions.ActionApi;
import com.ats.script.actions.ActionCallscript;
import com.ats.script.actions.ActionChannelStart;
import com.ats.script.actions.ActionComment;
import com.ats.script.actions.neoload.ActionNeoload;
import com.ats.script.actions.neoload.ActionNeoloadStop;
import com.ats.script.actions.performance.octoperf.ActionOctoperfVirtualUser;
import com.ats.tools.ResourceContent;
import com.ats.tools.logger.ExecutionLogger;
import com.ats.tools.logger.MessageCode;
import com.ats.tools.logger.levels.AtsFailError;
import com.ats.tools.performance.proxy.AtsNoProxy;
import com.ats.tools.performance.proxy.AtsProxy;
import com.ats.tools.performance.proxy.IAtsProxy;
import com.fasterxml.jackson.databind.JsonNode;
import com.google.gson.JsonObject;
public class Channel {
private final static String SYSTEM_VALUE = "#SYSTEM_VALUE#::";
public final static String HTML = "html";
public final static String DESKTOP = "desktop";
public static final String DESKTOP_EXPLORER = "explorer";
public final static String MOBILE = "mobile";
public final static String MOBILE_CHROME = "mobile-chrome";
public final static String API = "api";
public final static String SAP = "sap";
public static final String HTTP = "http";
public static final String HTTPS = "https";
public static final String BASIC_AUTHENTICATION = "Basic";
public static final String BEARER_AUTHENTICATION = "Bearer";
protected IDriverEngine engine;
private ActionChannelStart actionStart;
private boolean current = false;
protected ActionTestScript mainScript;
private final int scrollUnit = AtsManager.getScrollUnit();
private TestBound dimension = AtsSingleton.getInstance().getApplicationBound();
private TestBound subDimension = new TestBound();
private String driverVersion = "";
private String driverUrl = "";
protected SystemValues systemValues = new SystemValues();
private byte[] icon = new byte[0];
private String screenServer;
private ArrayList operations = new ArrayList<>();
private int winHandle = -1;
private long processId = 0;
private String warning = "";
private String neoloadDesignApi;
private String type = HTML;
private ArrayList systemProperties = new ArrayList<>();
public void addSystemProperties(Iterator iterator) {
if(iterator != null) {
while (iterator.hasNext()) {
systemProperties.add(iterator.next().asText());
}
}
}
private ArrayList systemButtons = new ArrayList<>();
public void addSystemButtons(Iterator iterator) {
if(iterator != null) {
while (iterator.hasNext()) {
systemButtons.add(iterator.next().asText());
}
}
}
//----------------------------------------------------------------------------------------------------------------------
// Constructor
//----------------------------------------------------------------------------------------------------------------------
public Channel() {}
public Channel(ActionChannelStart action, ActionTestScript script, DriverManager driverManager, String testName, int testLine) {
ActionStatus status = action.getStatus();
final SystemDriver systemDriver = new SystemDriver(status, script);
if (status.isPassed()) {
status.setChannel(this);
systemValues.setOsName(systemDriver.getOsName());
systemValues.setApplicationName(action.getApplication().getCalculated());
systemValues.setOsCountry(systemDriver.getCountryCode());
systemValues.setMachineName(systemDriver.getMachineName());
systemValues.setOsVersion(systemDriver.getOsVersion());
systemValues.setOsBuild(systemDriver.getOsBuildVersion());
systemValues.setScreenWidth(systemDriver.getScreenWidth().intValue());
systemValues.setScreenHeight(systemDriver.getScreenHeight().intValue());
this.mainScript = script;
this.current = true;
this.actionStart = action;
this.engine = driverManager.getDriverEngine(this, status, systemDriver, script);
if (status.isPassed()) {
systemValues.setApplicationPath(engine.getApplicationPath());
refreshLocation();
engine.started(script, status);
script.startChannel(action.getName());
//createVisualAction(true, action, testLine, testName, System.currentTimeMillis(), false);
}else {
script.startChannelFailed(testName, testLine, status.getChannelApplication(), status.getFailMessage());
//script.addShadowActionError(action, testLine, script.getStatus(), status.getFailMessage());
}
} else {
throw new AtsFailError(
action.getClass().getSimpleName(),
testName,
testLine,
"unable to start system driver, " + status.getErrorType(),
status.getMessage(),
script.getLogger());
}
status.endDuration();
}
public void waitBeforeMouseMoveToElement(WebDriverEngine webDriverEngine) {
AtsSingleton.getInstance().getWaitGuiReady().waitBeforeMouseMoveToElement(this, webDriverEngine);
}
public void waitBeforeSwitchWindow(WebDriverEngine webDriverEngine) {
AtsSingleton.getInstance().getWaitGuiReady().waitBeforeSwitchWindow(this, webDriverEngine);
}
public void waitBeforeSearchElement(WebDriverEngine webDriverEngine) {
AtsSingleton.getInstance().getWaitGuiReady().waitBeforeSearchElement(this, webDriverEngine);
}
public void waitBeforeEnterText(WebDriverEngine webDriverEngine) {
AtsSingleton.getInstance().getWaitGuiReady().waitBeforeEnterText(this, webDriverEngine);
}
public void waitBeforeGotoUrl(WebDriverEngine webDriverEngine) {
AtsSingleton.getInstance().getWaitGuiReady().waitBeforeGotoUrl(this, webDriverEngine);
}
//----------------------------------------------------------------------------------------------------------------------
//----------------------------------------------------------------------------------------------------------------------
public static String systemValueObj(String value) {
return SYSTEM_VALUE + value;
}
public static String checkSystemValue(Object o) {
final String s = o.toString();
if(s.startsWith(SYSTEM_VALUE)) {
return AtsSingleton.getInstance().getSystemValue(s.substring(16));
}
return s;
}
public String getSystemValue(String name) {
return systemValues.get(name);
}
public ActionTestScript getTestScript() {
return mainScript;
}
public String getTopScriptPackage() {
final String topScriptName = mainScript.getTopScript().getTestName();
final int lastDot = topScriptName.lastIndexOf(".");
if(lastDot > 0) {
return topScriptName.substring(0, lastDot);
}else {
return topScriptName;
}
}
public ActionStatus newActionStatus() {
return new ActionStatus(this, "", 0);
}
public void newActionStatus(Action action, IVisualRecorder recorder, String testName, int testLine, boolean start) {
final ActionStatus st = new ActionStatus(this, testName, testLine);
action.setStatus(st);
if(st.isPassed() && start) {
action.initAction(this, st.getTestLine(), recorder, testName, testLine);
}
}
public SystemDriver getSystemDriver() {
if(engine != null) {
return engine.getSystemDriver();
}
return null;
}
public boolean isDesktopDriverEnabled() {
return engine.getSystemDriver() != null && engine.getSystemDriver().isEnabled();
}
public void cleanHandle() {
winHandle = -1;
setWindowToFront();
}
public void setWinHandle(int hdl) {
winHandle = hdl;
}
public void updateWinHandle(SystemDriver drv, int index) {
winHandle = getHandle(drv, index);
getSystemDriver().updateWindowHandle(this);
}
public int getHandle(SystemDriver drv) {
if(winHandle < 0 && isDesktopDriverEnabled()) {
winHandle = getHandle(drv, 0);
}
return winHandle;
}
public int getHandle() {
return winHandle;
}
public int getHandle(SystemDriver drv, int index) {
List processWindows = drv.getWindowsByPid(getProcessId());
if(processWindows != null && processWindows.size() > index) {
return processWindows.get(index).getHandle();
}
return -1;
}
public void refreshLocation(){
engine.updateDimensions();
}
public void setDimensions(TestBound dim1, TestBound dim2) {
setDimension(dim1);
setSubDimension(dim2);
}
public double getOffsetY() {
return dimension.getHeight() - subDimension.getHeight();
}
public void refreshMapElementLocation(){
refreshLocation();
engine.refreshElementMapLocation();
}
public void defineRoot(String id) {
getSystemDriver().defineRoot(dimension, id);
}
public void toFront(){
if(isDesktopDriverEnabled()) {
getSystemDriver().setChannelToFront(getHandle(getSystemDriver()), processId);
}
}
public void setWindowToFront(){
if(isDesktopDriverEnabled()) {
engine.setWindowToFront();
}
}
public String rootKeys(ActionStatus status, CalculatedValue text){
final String textValue = text.uncrypt(getTestScript(), text.getCalculated());
getSystemDriver().rootKeys(getHandle(getSystemDriver()), textValue);
actionTerminated(status);
return textValue;
}
public String getSource(){
return engine.getSource();
}
//---------------------------------------------------------------------------
// Screen shot management
//---------------------------------------------------------------------------
public byte[] getScreenShot(TestBound dim) {
dim.setX(dim.getX() + dimension.getX());
dim.setY(dim.getY() + dimension.getY());
return getScreenShotEngine(dim);
}
public byte[] getScreenShot(){
return getScreenShotEngine(dimension);
}
protected byte[] getScreenShotEngine(TestBound dim) {
mainScript.sleep(100);
return getSystemDriver().getScreenshotByte(dim.getX(), dim.getY(), dim.getWidth(), dim.getHeight());
}
//---------------------------------------------------------------------------
//---------------------------------------------------------------------------
public String getAuthenticationValue() {
return actionStart.getAuthenticationValue();
}
public ArrayList getArguments() {
return actionStart.getArguments();
}
public boolean isUseCookie() {
return actionStart.isUseCookie();
}
public void setNeoloadDesignApi(String value) {
this.neoloadDesignApi = value;
}
//--------------------------------------------------------------------------------------------------
// Api webservices init
//--------------------------------------------------------------------------------------------------
public void setApplicationData(String type, String os) {
setType(type);
setIcon(ResourceContent.getAtsByteLogo());
driverVersion = ATS.getAtsVersion();
dimension = new TestBound(0D, 0D, 1D, 1D);
systemValues.setOsName(os);
}
public void setApplicationData(String type, String os, String serviceType, ArrayList op, byte[] ico) {
setType(type);
setIcon(ico);
systemValues.setOsName(os);
systemValues.setApplicationName(serviceType);
dimension = new TestBound(0D, 0D, 1D, 1D);
operations = op;
}
public void setApplicationData(String type, String os, String serviceType, byte[] ico) {
setType(type);
setIcon(ico);
systemValues.setOsName(os);
systemValues.setApplicationName(serviceType);
dimension = new TestBound(0D, 0D, 1D, 1D);
}
//--------------------------------------------------------------------------------------------------
//--------------------------------------------------------------------------------------------------
public void setApplicationData(String type, String os, String version, String dv, long pid, byte[] ico, String screen) {
setType(type);
setIcon(ico);
this.driverVersion = dv;
this.processId = pid;
this.screenServer = screen;
this.systemValues.setOsName(os);
this.systemValues.setApplicationVersion(version);
}
public void setApplicationData(String type, String os, int handle, byte[] ico) {
setType(type);
setIcon(ico);
this.driverVersion = "";
this.winHandle = handle;
this.systemValues.setOsName(os);
}
public void setApplicationData(String type, String version, byte[] ico) {
setType(type);
setIcon(ico);
this.systemValues.setOsVersion(version);
}
public void setApplicationData(String type, String os, String version, String dVersion, long pid, byte[] icon) {
setApplicationData(type, os, version, dVersion, pid, icon, "");
}
public void setApplicationData(String type, String os, String name, String version, String dVersion, long pid, int handle, byte[] icon, String warn) {
setApplicationData(type, os, name, version, dVersion, pid, handle, icon);
this.warning = warn;
}
public void setApplicationData(String type, String os, String name, String version, String dVersion, long pid, int handle, byte[] icon, String warn, String sapUrl) {
setApplicationData(type, os, name, version, dVersion, pid, handle, icon, warn);
this.driverUrl = sapUrl;
}
public void setApplicationData(String type, String os, String name, String version, String dVersion, long pid, int handle, byte[] icon) {
setApplicationData(type, os, version, dVersion, pid, icon, "");
this.systemValues.setApplicationName(name);
this.winHandle = handle;
}
public void setApplicationData(String type, String os, String name, String version, String dVersion, long pid) {
setApplicationData(type, os, version, dVersion, pid, new byte[0], "");
this.systemValues.setApplicationName(name);
}
public void setApplicationData(String type, String os, String version, String dVersion, long pid, long handle) {
setApplicationData(type, os, version, dVersion, pid, new byte[0], "");
this.winHandle = (int) handle;
}
//--------------------------------------------------------------------------------------------------
//--------------------------------------------------------------------------------------------------
public void switchToFrame(String id) {
engine.switchToFrameId(id);
}
public void clearData() {
setIcon(null);
operations.clear();
}
//----------------------------------------------------------------------------------------------------------------------
// Elements
//----------------------------------------------------------------------------------------------------------------------
public FoundElement getElementFromPoint(Boolean syscomp, Double x, Double y){
return engine.getElementFromPoint(syscomp, x, y);
}
public FoundElement getElementFromRect(Boolean syscomp, Double x, Double y, Double w, Double h){
return engine.getElementFromRect(syscomp, x, y, w, h);
}
public void loadParents(FoundElement hoverElement) {
if(hoverElement != null) {
engine.loadParents(hoverElement);
}
}
public CalculatedProperty[] getCssAttributes(FoundElement element){
return engine.getCssAttributes(element);
}
public CalculatedProperty[] getHtmlAttributes(FoundElement element){
return engine.getHtmlAttributes(element);
}
public CalculatedProperty[] getAttributes(FoundElement element){
return engine.getAttributes(element, false);
}
public List findSelectOptions(TestElement element){
return engine.loadSelectOptions(element);
}
public String getAttribute(ActionStatus status, FoundElement element, String attributeName, int maxTry){
return engine.getAttribute(status, element, attributeName, maxTry + AtsSingleton.getInstance().getMaxTryProperty());
}
public void setSysProperty(String attributeName, String attributeValue) {
engine.setSysProperty(attributeName, attributeValue);
}
//----------------------------------------------------------------------------------------------------------------------
public String getOs() {
return systemValues.getOsName();
}
public String getApplication() {
return systemValues.getApplicationName();
}
public String getApplicationVersion() {
return systemValues.getApplicationVersion();
}
public boolean attachToExistingProcess() {
if(actionStart != null) {
return actionStart.isAttach();
}
return false;
}
public boolean isSameName(String value) {
return actionStart.getName().equalsIgnoreCase(value);
}
public boolean isDesktop() {
return engine instanceof SystemDriverEngine || engine instanceof SapDriverEngine;
}
public boolean isSap() {
return engine instanceof SapDriverEngine;
}
public void setZoom(double d) {
int z = (int) Math.round(d);
if(z != 100) {
warning += "Browser zoom is " + z + "% (it should be 100%)";
}
}
public void tryAndWait(int code, String message, int max, int left) {
sendWarningLog(code, message, left);
progressiveWait(max - left);
}
//----------------------------------------------------------------------------------------------------------------------
// logs
//----------------------------------------------------------------------------------------------------------------------
public void sendLog(int code, String message, Object value) {
mainScript.getLogger().sendLog(code, message, value);
}
public void sendWarningLog(int code, String message, Object value) {
mainScript.getLogger().sendWarning(code, message, value);
}
public void sendWarningLog(String message, Object value) {
mainScript.getLogger().sendWarning(MessageCode.ACTION_IN_PROGRESS, message, value);
}
//----------------------------------------------------------------------------------------------------------------------
// Getter and setter for serialization
//----------------------------------------------------------------------------------------------------------------------
public String getWarning() {
return warning;
}
public void setWarning(String warning) {
this.warning = warning;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public ArrayList getSystemProperties() {
return systemProperties;
}
public void setSystemProperties(ArrayList props) {
systemProperties = props;
}
public ArrayList getSystemButtons() {
return systemButtons;
}
public void setSystemButtons(ArrayList buttons) {
systemButtons = buttons;
}
public ArrayList getOperations() {
return operations;
}
public void setOperations(ArrayList operations) {
this.operations = operations;
}
public SystemValues getSystemValues(){
return systemValues;
}
public void setSystemValues(SystemValues value) {} //read only
public String getDriverVersion() {
return driverVersion;
}
public void setDriverVersion(String url) {} // read only
public String getName() {
return actionStart.getName();
}
public void setName(String name) {} // read only
public String getAuthentication() {
if(actionStart.getAuthentication() != null && actionStart.getAuthenticationValue() != null && actionStart.getAuthentication().length() > 0 && actionStart.getAuthenticationValue().length() > 0) {
return actionStart.getAuthentication();
}
return "";
}
public void setAuthentication(String value) {} // read only
public int getPerformance() {
return actionStart.getPerformance();
}
public void setPerformance(int value) {
actionStart.setPerformance(value);
}
public boolean isCurrent() {
return current;
}
public void setCurrent(boolean value) {
this.current = value;
if(value){
setWindowToFront();
}
}
public byte[] getIcon() {
return icon;
}
public void setIcon(byte[] value) {
this.icon = value;
}
public String getScreenServer() {
return screenServer;
}
public void setScreenServer(String value) {
this.screenServer = value;
}
public TestBound getDimension() {
return dimension;
}
public void setFullScreenSize(long w, long h) {
this.dimension = new TestBound(0D, 0D, (double)w, (double)h);
}
public void setDimension(TestBound dimension) {
this.dimension = dimension;
}
public String getBoundDimension() {
return dimension.getX().intValue() + "," + dimension.getY().intValue() + "," + dimension.getWidth().intValue() + "," + dimension.getHeight().intValue();
}
public long getProcessId() {
return processId;
}
public void setProcessId(long value) {} //read only
public TestBound getSubDimension(){
return subDimension;
}
public void setSubDimension(TestBound dimension){
this.subDimension = dimension;
}
public String getDriverUrl() {
return driverUrl;
}
public void setDriverUrl(String value) {
this.driverUrl = value;
}
//----------------------------------------------------------------------------------------------------------------------
//----------------------------------------------------------------------------------------------------------------------
public void close(){
close(newActionStatus());
}
public void close(ActionStatus status){
if(stopNeoloadRecord != null) {
neoloadAction(stopNeoloadRecord, "", 0);
}
closeAtsProxy();
engine.close();
AtsSingleton.getInstance().channelClosed(status, this);
}
//----------------------------------------------------------------------------------------------------------
// Browser's secific parameters
//----------------------------------------------------------------------------------------------------------
public void progressiveWait(int value) {
sleep(200 + value*50);
}
public void sleep(int ms){
mainScript.sleep(ms);
}
public void actionTerminated(ActionStatus status){
engine.waitAfterAction(status);
}
//----------------------------------------------------------------------------------------------------------
// driver actions
//----------------------------------------------------------------------------------------------------------
public WebElement getRootElement() {
return engine.getRootElement(this);
}
public void switchWindow(ActionStatus status, int index, int tries, int delay, boolean refresh){
if(delay > 0 && delay < 100) {
sleep(delay*1000);
}
boolean reload = engine.switchWindow(status, index, tries, refresh);
if(status.isPassed()) {
engine.updateDimensions();
if(reload) {
updateWinHandle(getSystemDriver(), index);
}
}
}
public String updateWindowBound(ActionStatus actionStatus, BoundData x, BoundData y, BoundData w, BoundData h) {
String bounds = engine.setWindowBound(x, y, w, h);
engine.updateDimensions();
sleep(100);
getSystemDriver().updateVisualImage(getDimension(),false);
return bounds;
}
public void closeWindow(ActionStatus status){
engine.closeWindow(status);
}
public void windowState(ActionStatus status, String state){
engine.windowState(status, this, state);
}
public Object executeScript(ActionStatus status, String script, Object ... params){
return engine.executeScript(status, script, params);
}
public DialogBox switchToAlert() {
return engine.switchToAlert();
}
public void switchToDefaultContent(boolean dialog) {
engine.switchToDefaultContent(dialog);
}
public void navigate(ActionStatus status, String url) {
engine.goToUrl(status, url);
}
public void api(ActionStatus status, ActionApi api) {
engine.api(status, api);
}
public IDriverEngine getSystemDriverEngine() {
return getSystemDriver().getEngine();
}
public IDriverEngine getDriverEngine() {
return engine;
}
public List getShapes(String duration, String device, int[] rect) throws Exception {
return getSystemDriver().getShapes(dimension, duration, device, rect);
}
public void saveAppSource(String testName) {
/* final Path outPath = getTestScript().getSuiteOutputPath().resolve(testName + "_fail.html");
try {
final FileWriter fw = new FileWriter(outPath.toFile());
fw.write(getDriverEngine().getSource());
fw.close();
} catch (IOException e) {
e.printStackTrace();
} */
}
//----------------------------------------------------------------------------------------------------------
//----------------------------------------------------------------------------------------------------------
public void scroll(int delta) {
engine.scroll(delta*scrollUnit);
}
public void scroll(FoundElement foundElement, int delta) {
engine.scroll(foundElement, delta*scrollUnit);
}
public void mouseMoveToElement(ActionStatus status, FoundElement foundElement) {
engine.mouseMoveToElement(foundElement);
actionTerminated(status);
}
public void mouseMoveToElement(ActionStatus status, FoundElement foundElement, MouseDirection position) {
engine.mouseMoveToElement(status, foundElement, position, false, 0, 0);
actionTerminated(status);
}
public void buttonClick(ActionStatus status, String buttonType) {
engine.buttonClick(status, buttonType);
}
//----------------------------------------------------------------------------------------------------------
// Performance
//----------------------------------------------------------------------------------------------------------
private ActionNeoloadStop stopNeoloadRecord = null;
public void neoloadAction(ActionNeoload action, String testName, int testLine) {
newActionStatus(action, new VisualRecorderNull(), testName, testLine, true);
if(getPerformance() == ActionChannelStart.NEOLOAD) {
if(neoloadDesignApi != null) {
action.executeRequest(this, neoloadDesignApi);
}else {
action.getStatus().setPassed(false);
action.getStatus().setMessage("Neoload design API is not defined in .atsProperties !");
}
}else {
action.getStatus().setPassed(true);
}
}
public void setStopNeoloadRecord(ActionNeoloadStop value) {
this.stopNeoloadRecord = value;
}
//----------------------------------------------------------------------------------------------------------
private IAtsProxy atsProxy = new AtsNoProxy();
public Proxy startAtsProxy(AtsManager ats) {
atsProxy = new AtsProxy(getName(), getApplication(), ats.getBlackListServers(), ats.getTrafficIdle(), ats.getOctoperf());
return atsProxy.startProxy();
}
public void startHarServer(ActionStatus status, List whiteList, int trafficIddle, int latency, long sendBandWidth, long receiveBandWidth) {
atsProxy.startRecord(status, whiteList, trafficIddle, latency, sendBandWidth, receiveBandWidth);
}
public void pauseHarRecord() {
atsProxy.pauseRecord();
}
public void resumeHarRecord() {
atsProxy.resumeRecord();
}
public void startHarAction(Action action, String testLine) {
atsProxy.startAction(action, testLine);
}
public void endHarAction() {
atsProxy.endAction();
}
public void sendToOctoperfServer(ActionOctoperfVirtualUser action) {
atsProxy.sendToOctoperfServer(this, action);
}
public void closeAtsProxy() {
atsProxy.terminate(getName());
atsProxy = new AtsNoProxy();
}
public void addStepComment(ActionComment action, IVisualRecorder recorder, String type, String calculated) {
recorder.update(type, calculated);
}
public void updateSummary(ActionComment action, IVisualRecorder recorder, String testName, int testLine, String calculated) {
recorder.updateSummary(testName, testLine, calculated);
}
//----------------------------------------------------------------------------------------------------------
// Visual reporting
//----------------------------------------------------------------------------------------------------------
//we can now use 'engine.getDriverInfo().getScreenshotUrl()' to send to the json value to set alternative function to capture screenshot
//JsonNode node = engine.getDriverInfo().toJson();
public void addShadowActionError(Action action, int line, TestError.TestErrorStatus testErrorStatus) {}
public void addShadowAction(Action action, int line) {}
public void switchTo(ActionStatus status, ActionTestScript ts, String testName, int testLine, String name) {
status.setNoError();
if(AtsSingleton.getInstance().switchChannel(status, name)) {
getSystemDriver().updateVisualImage(AtsSingleton.getInstance().getChannel(name).getDimension(), false);
ts.getRecorder().update(name);
//status.setValue(name);
}else {
/*ExecutionLogger logger = ts.getTopScript().getLogger();
status.setValue("channel '" + name + "' not found !");
status.setError(testLine, name);
executionLogger.sendWarning("switch channel is not possible !", "channel '" + name + "' is not running");*/
ts.getRecorder().update(ActionStatus.CHANNEL_NOT_FOUND, 0, name, "channel is not running");
status.setError(ActionStatus.CHANNEL_NOT_FOUND, "channel '" + name + "' is not running");
}
}
public void closeChannel(ActionStatus status, ActionTestScript ts, String testName, int testLine, String name) {
status.setNoError();
if(AtsSingleton.getInstance().closeChannel(status, name)) {
ts.getRecorder().update(name, "{\"name\":\"" + name + "\",\"app\":\"" + status.getValue() + "\"}");
ts.getRecorder().updateScreen(false);
status.setValue(name);
}else {
ts.getRecorder().update(ActionStatus.CHANNEL_NOT_FOUND, 0, name, "{\"name\":\"" + name + "\",\"app\":\"[N/A]\", \"error\":\"channel is not running\"}");
status.setError(ActionStatus.CHANNEL_NOT_FOUND, "channel '" + name + "' is not running");
}
}
public void updateVisualCallScript(String calledScript, IVisualRecorder recorder, ActionCallscript action, String testName, int line) {
recorder.update(calledScript);
}
public void updateRecorderData(ActionTestScript ts, String calledScript, JsonObject paramsData) {
if (paramsData != null) {
ts.getRecorder().update(calledScript, paramsData.toString());
}
}
public void updateRecorderFilePathData(ActionTestScript ts, String calledScript, JsonObject paramsData, String filePath) {
if (paramsData == null) {
paramsData = new JsonObject();
}
if (filePath.startsWith("file")) {
paramsData.addProperty("filePath", filePath);
} else if (filePath.startsWith("http")) {
paramsData.addProperty("http", filePath);
} else if (filePath.startsWith("asset")) {
paramsData.addProperty("asset", filePath);
}
if (paramsData != null && !paramsData.isEmpty()) {
updateRecorderData(ts, calledScript, paramsData);
}
}
public void createVisualAction(IVisualRecorder recorder, Action action, String testName, int line) {
recorder.createVisualAction(action, testName, line);
}
public void createVisualAction(boolean stop, Action action, int scriptLine, String scriptName, long timeline, boolean sync) {
if(engine != null) {
engine.createVisualAction(this, stop, action.getClass().getName(), scriptLine, scriptName, timeline, sync);
}
}
public void updateVisualAction(boolean isRef) {
if(engine != null) {
engine.updateScreenshot(this.dimension, isRef);
}
}
public DesktopResponse startVisualRecord(ScriptHeader header, int quality, long started) {
if(getSystemDriver() != null) {
return getSystemDriver().startVisualRecord(header, quality, started);
}
return null;
}
public void stopVisualRecord(ScriptStatus status, TestSummary summary) {
if(getSystemDriver() != null) {
getSystemDriver().saveSummary(status, summary);
getSystemDriver().stopVisualRecord();
}
}
public void saveVisualReportFile(Path path, String fileName, ExecutionLogger logger) {
if(getSystemDriver() != null) {
getSystemDriver().saveVisualReportFile(path.resolve(fileName), logger);
}
}
public void updateVisualAction(String value) {
getSystemDriver().updateVisualValue(value);
}
public void updateVisualAction(String value, String data) {
getSystemDriver().updateVisualData(value, data);
}
public void updateVisualAction(String type, MouseDirectionData hdir, MouseDirectionData vdir) {
getSystemDriver().updateVisualPosition(type, hdir, vdir);
}
public void updateVisualAction(TestElement element) {
getSystemDriver().updateVisualElement(element);
}
public void updateVisualAction(int error, long duration) {
getSystemDriver().updateVisualStatus(error, duration);
}
public void updateVisualAction(int error, long duration, String value) {
getSystemDriver().updateVisualStatus(error, duration);
getSystemDriver().updateVisualValue(value);
}
public void updateVisualAction(int error, long duration, String value, String data) {
if(getSystemDriver() != null) {
getSystemDriver().updateVisualStatus(error, duration);
getSystemDriver().updateVisualData(value, data);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy