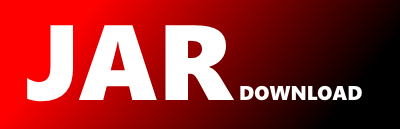
com.ats.executor.channels.ChannelManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.channels;
import java.util.ArrayList;
import java.util.Optional;
import com.ats.AtsSingleton;
import com.ats.driver.ApplicationProperties;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.drivers.DriverManager;
import com.ats.script.actions.ActionChannelStart;
import com.ats.tools.Utils;
import com.ats.tools.wait.IWaitGuiReady;
import com.ats.tools.wait.WaitGuiReady;
public class ChannelManager {
private Channel currentChannel;
private ActionTestScript mainScript;
private ArrayList channelsList = new ArrayList<>();
private DriverManager driverManager = new DriverManager();
public ChannelManager() {}
public void setMainScript(ActionTestScript script) {
this.mainScript = script;
final IWaitGuiReady guiReady = AtsSingleton.getInstance().getWaitGuiReady();
if(guiReady != null && !(guiReady instanceof WaitGuiReady)) {
script.getLogger().sendInfo("Custom WaitGuiReady class found ", guiReady.toString());
}
}
public int getMaxTry() {
return AtsSingleton.getInstance().getMaxTrySearch();
}
public int getMaxTryScroll() {
return AtsSingleton.getInstance().getMaxTryScrollSearch();
}
public Channel getCurrentChannel(){
if(currentChannel == null) {
currentChannel = new EmptyChannel();
}
return currentChannel;
}
public Channel[] getChannelsList(){
if(channelsList.size() > 0){
return channelsList.toArray(new Channel[channelsList.size()]);
}else{
return new Channel[0];
}
}
private void setCurrentChannel(Channel channel){
for(Channel cnl : channelsList){
cnl.setCurrent(cnl == channel);
}
channel.setCurrent(true);
currentChannel = channel;
}
public void closeAllChannels(){
while(channelsList.size() > 0){
channelsList.remove(0).close();
}
}
public Channel getChannel(String name){
for(Channel cnl : channelsList){
if(cnl.isSameName(name)){
currentChannel = cnl;
return cnl;
}
}
return new EmptyChannel();// Channel with name : does not exist or has been closed
}
public void startChannel(ActionChannelStart action, String testName, int testLine){
String name = action.getName();
if(name == null || name.isBlank()) {
name = "[CNL-" + Utils.getShortUid() + "]";
action.setName(name);
}
final String appName = action.getApplication().getCalculated();
final ApplicationProperties props = AtsSingleton.getInstance().getApplicationProperties(appName);
action.setStatus(new ActionStatus(testName, testLine));
Channel channel = getChannel(name);
if(channel instanceof EmptyChannel){
if (props.isMobile() || appName.startsWith(Channel.MOBILE + "://")) {
channel = new MobileChannel(action, mainScript, driverManager, testName, testLine);
}else if(appName.startsWith(Channel.MOBILE_CHROME + "://")) {
channel = new MobileChromeChannel(action, mainScript, driverManager, testName, testLine);
} else {
channel = new Channel(action, mainScript, driverManager, testName, testLine);
}
action.getStatus().setChannel(channel);
if(action.getStatus().isPassed()) {
for(Channel cn : channelsList) {
cn.clearData();
}
addChannel(channel);
mainScript.getRecorder().createVisualStartChannelAction(action, action.getStatus().getDuration(), testName, testLine);
}//else {
//mainScript.startChannelFailed(testName, testLine, appName, action.getStatus().getFailMessage());
//}
//action.getStatus().setData(getChannelsList());
}else {
setCurrentChannel(channel);
action.getStatus().setChannel(channel);
}
action.getStatus().setData(getChannelsList());
}
private void addChannel(Channel cnl) {
channelsList.add(cnl);
setCurrentChannel(cnl);
}
public void switchToLastChannel(ActionStatus status){
if(channelsList != null && channelsList.size() > 0){
switchChannel(status, channelsList.get(channelsList.size() - 1).getName());
}else {
currentChannel = new EmptyChannel();
}
}
public boolean switchChannel(ActionStatus status, String name){
status.startDuration();
if(channelsList != null){
for(Channel cnl : channelsList){
if(cnl.isSameName(name)){
if(!cnl.isCurrent()) {
setCurrentChannel(cnl);
status.setData(getChannelsList());
status.setChannel(cnl);
}
status.setPassed(true);
status.endDuration();
return true;
}
}
}
//status.setError(ActionStatus.CHANNEL_NOT_FOUND, "channel [" + name + "] is not running");
return false;
}
public boolean closeChannel(ActionStatus status, String channelName){
final Optional cn = channelsList.stream().filter(c -> c.isSameName(channelName)).findFirst();
if(cn.isPresent()) {
cn.get().close(status);
return true;
}
return false;
}
public void channelClosed(ActionStatus status, Channel channel){
status.startDuration();
if(channelsList.remove(channel)) {
if(channelsList.size() > 0){
if(channel.isCurrent()) {
final Channel current = channelsList.get(0);
setCurrentChannel(current);
status.setChannel(current);
}
}else{
currentChannel = new EmptyChannel();
}
status.setNoError();
status.setData(getChannelsList());
status.setValue(channel.getApplication());
}else {
status.setError(ActionStatus.CHANNEL_START_ERROR, "channel '" + channel.getName() + "' not found");
}
status.endDuration();
}
//----------------------------------------------------------------------------------------------------------------------
//----------------------------------------------------------------------------------------------------------------------
//TODO add channel management in execution as a suite context
public void tearDown() {
closeAllChannels();
closeMobileDriver();
}
public void closeMobileDriver() {
driverManager.tearDown();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy