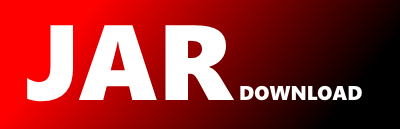
com.ats.executor.channels.EmptyChannel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.channels;
import java.nio.file.Path;
import com.ats.element.DialogBox;
import com.ats.element.test.TestElement;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.ScriptStatus;
import com.ats.executor.drivers.desktop.DesktopResponse;
import com.ats.generator.objects.BoundData;
import com.ats.generator.objects.MouseDirectionData;
import com.ats.recorder.IVisualRecorder;
import com.ats.recorder.TestError;
import com.ats.recorder.TestSummary;
import com.ats.script.ScriptHeader;
import com.ats.script.actions.Action;
import com.ats.script.actions.ActionCallscript;
import com.ats.script.actions.ActionComment;
import com.ats.tools.logger.ExecutionLogger;
import com.google.gson.JsonObject;
public class EmptyChannel extends Channel {
@Override
public String getType() {
return "";
}
@Override
public String getName() {
return "";
}
@Override
public String getAuthentication() {
return "";
}
@Override
public int getPerformance() {
return 0;
}
@Override
public boolean isCurrent() {
return false;
}
@Override
public byte[] getIcon() {
return new byte[0];
}
@Override
public String getScreenServer() {
return "";
}
@Override
public void actionTerminated(ActionStatus status) {
status.setError(ActionStatus.CHANNEL_NOT_STARTED, "No channel started !");
}
@Override
public void setWindowToFront() {
}
@Override
public void refreshLocation() {
}
@Override
public void sleep(int ms) {
}
@Override
public String getSystemValue(String name) {
return systemValueObj(name);
}
@Override
public void closeChannel(ActionStatus status, ActionTestScript ts, String testName, int testLine, String name) {
ts.getTopScript().getLogger().sendWarning("ActionChannelClose (" + testName + ":" + testLine + ")", "Cannot close Channel '" + getName() + "', this channel is not running !");
}
@Override
public void navigate(ActionStatus status, String url) {
setNoChannelError(status);
}
@Override
public void newActionStatus(Action action, IVisualRecorder recorder, String testName, int testLine, boolean start) {
ActionStatus status = new ActionStatus(this, testName, testLine);
setNoChannelError(status);
action.setStatus(status);
}
@Override
public void switchWindow(ActionStatus status, int index, int tries, int delay, boolean refresh){
setNoChannelError(status);
}
@Override
public void switchTo(ActionStatus status, ActionTestScript ts, String testName, int testLine, String name) {
ts.getLogger().sendWarning("ActionChannelSwitch (" + testName + ":" + testLine + ")", "Cannot switch to Channel '" + name + "', this channel is not running !");
}
@Override
public String updateWindowBound(ActionStatus actionStatus, BoundData x, BoundData y, BoundData w, BoundData h) {
return "";
}
@Override
public void closeWindow(ActionStatus status){
setNoChannelError(status);
}
@Override
public void windowState(ActionStatus status, String state){
setNoChannelError(status);
}
@Override
public Object executeScript(ActionStatus status, String script, Object ... params){
return new Object();
}
@Override
public DialogBox switchToAlert() {
return null;
}
@Override
public void addStepComment(ActionComment action, IVisualRecorder recorder, String type, String calculated) {
action.addShadowAction();
}
@Override
public void updateSummary(ActionComment action, IVisualRecorder recorder, String testName, int testLine, String calculated) {
}
@Override
public void addShadowAction(Action action, int line) {
action.addShadowAction();
}
private void setNoChannelError(ActionStatus status) {
status.setError(ActionStatus.CHANNEL_NOT_STARTED, "No channel started !");
}
//----------------------------------------------------------------------------------------------------------
// Override Visual reporting
//----------------------------------------------------------------------------------------------------------
@Override
public void addShadowActionError(Action action, int line, TestError.TestErrorStatus testErrorStatus) {
action.addShadowActionError(line, testErrorStatus);
}
@Override
public DesktopResponse startVisualRecord(ScriptHeader script, int quality, long started) {
return null;
}
@Override
public void updateVisualCallScript(String calledScript, IVisualRecorder recorder, ActionCallscript action, String testName, int line) {
action.addShadowAction();
}
@Override
public void updateRecorderData(ActionTestScript ts, String calledScript, JsonObject paramsData) {
}
@Override
public void updateRecorderFilePathData(ActionTestScript ts, String calledScript, JsonObject paramsData, String filePath) {
}
@Override
public void createVisualAction(IVisualRecorder recorder, Action action, String testName, int line) {
action.addShadowAction();
}
@Override
public void createVisualAction(boolean stop, Action action, int scriptLine, String scriptName, long timeline, boolean sync) {
action.addShadowAction();
}
@Override
public void stopVisualRecord(ScriptStatus status, TestSummary summary) {
}
@Override
public void saveVisualReportFile(Path path, String fileName, ExecutionLogger logger) {
}
@Override
public void updateVisualAction(boolean isRef) {
}
@Override
public void updateVisualAction(String value) {
}
@Override
public void updateVisualAction(String value, String data) {
}
@Override
public void updateVisualAction(String type, MouseDirectionData hdir, MouseDirectionData vdir) {
}
@Override
public void updateVisualAction(TestElement element) {
}
@Override
public void updateVisualAction(int error, long duration) {
}
@Override
public void updateVisualAction(int error, long duration, String value) {
}
@Override
public void updateVisualAction(int error, long duration, String value, String data) {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy