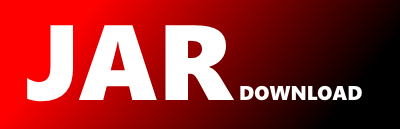
com.ats.executor.channels.SystemValues Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.channels;
public class SystemValues {
public static final String SYS_OS_NAME = "os-name";
public static final String SYS_OS_FAMILLY = "os-familly";
public static final String SYS_OS_VERSION = "os-version";
public static final String SYS_OS_BUILD = "os-build";
public static final String SYS_OS_COUNTRY = "os-country";
public static final String SYS_MACHINE_NAME = "machine-name";
public static final String SYS_APP_NAME = "app-name";
public static final String SYS_APP_VERSION = "app-version";
public static final String SYS_USER_NAME = "user-name";
public static final String SYS_SCREEN_WIDTH = "screen-width";
public static final String SYS_SCREEN_HEIGHT = "screen-height";
public final static String[] VALUES_NAME = new String[]{SYS_OS_NAME, SYS_OS_FAMILLY, SYS_OS_VERSION, SYS_OS_BUILD, SYS_OS_COUNTRY, SYS_MACHINE_NAME, SYS_APP_NAME, SYS_APP_VERSION, SYS_USER_NAME, SYS_SCREEN_WIDTH, SYS_SCREEN_HEIGHT};
//----------------------------------------------------------------------------------------------------------------------
// Instance
//----------------------------------------------------------------------------------------------------------------------
private String osName = "";
private String osFamilly = "";
private String osVersion = "";
private String osBuild = "";
private String osCountry = "";
private String machineName = "";
private String applicationName = "";
private String applicationPath = "";
private String applicationVersion = "";
private String userName = System.getProperty("user.name");
private int screenWidth = 0;
private int screenHeight = 0;
public String get(String name) {
if(name != null) {
switch(name) {
case SYS_OS_NAME:
return osName;
case SYS_OS_FAMILLY:
return osFamilly;
case SYS_OS_VERSION:
return osVersion;
case SYS_OS_BUILD:
return osBuild;
case SYS_OS_COUNTRY:
return osCountry;
case SYS_MACHINE_NAME:
return machineName;
case SYS_APP_NAME:
return applicationName;
case SYS_APP_VERSION:
return applicationVersion;
case SYS_USER_NAME:
return userName;
case SYS_SCREEN_WIDTH:
return String.valueOf(screenWidth);
case SYS_SCREEN_HEIGHT:
return String.valueOf(screenHeight);
}
}
return "";
}
//----------------------------------------------------------------------------------------------------------------------
// Getter and setter for serialization
//----------------------------------------------------------------------------------------------------------------------
public String getOsName() {
return osName;
}
public void setOsName(String value) {
this.osName = value;
setOsFamilly(value.toUpperCase());
}
public String getOsFamilly() {
return osFamilly;
}
public void setOsFamilly(String value) {
this.osFamilly = value;
}
public String getOsVersion() {
return osVersion;
}
public void setOsVersion(String value) {
this.osVersion = value;
}
public String getOsBuild() {
return osBuild;
}
public void setOsBuild(String value) {
this.osBuild = value;
}
public String getOsCountry() {
return osCountry;
}
public void setOsCountry(String value) {
this.osCountry = value;
}
public String getMachineName() {
return machineName;
}
public void setMachineName(String value) {
this.machineName = value;
}
public String getApplicationName() {
if(applicationName != null) {
return applicationName;
}
return "";
}
public void setApplicationName(String value) {
this.applicationName = value;
}
public String getApplicationPath() {
return applicationPath;
}
public void setApplicationPath(String value) {
this.applicationPath = value;
}
public String getApplicationVersion() {
return applicationVersion;
}
public void setApplicationVersion(String value) {
this.applicationVersion = value;
}
public String getUserName() {
return userName;
}
public void setUserName(String value) {
this.userName = value;
}
public int getScreenWidth() {
return screenWidth;
}
public void setScreenWidth(int value) {
this.screenWidth = value;
}
public int getScreenHeight() {
return screenHeight;
}
public void setScreenHeight(int value) {
this.screenHeight = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy