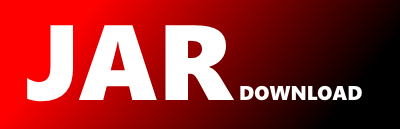
com.ats.executor.drivers.DriverManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers;
import java.util.ArrayList;
import com.ats.AtsSingleton;
import com.ats.driver.ApplicationProperties;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.channels.Channel;
import com.ats.executor.channels.MobileChannel;
import com.ats.executor.channels.MobileChromeChannel;
import com.ats.executor.drivers.desktop.SystemDriver;
import com.ats.executor.drivers.engines.ApiDriverEngine;
import com.ats.executor.drivers.engines.IDriverEngine;
import com.ats.executor.drivers.engines.MobileDriverEngine;
import com.ats.executor.drivers.engines.SapDriverEngine;
import com.ats.executor.drivers.engines.SystemDriverEngine;
import com.ats.executor.drivers.engines.browsers.BraveDriverEngine;
import com.ats.executor.drivers.engines.browsers.ChromeDriverEngine;
import com.ats.executor.drivers.engines.browsers.ChromiumDriverEngine;
import com.ats.executor.drivers.engines.browsers.FirefoxDriverEngine;
import com.ats.executor.drivers.engines.browsers.IEDriverEngine;
import com.ats.executor.drivers.engines.browsers.MsEdgeDriverEngine;
import com.ats.executor.drivers.engines.browsers.OperaDriverEngine;
import com.ats.executor.drivers.engines.browsers.SafariDriverEngine;
import com.ats.executor.drivers.engines.desktop.ExplorerDriverEngine;
import com.ats.executor.drivers.engines.mobiles.MobileChromeDriverEngine;
public class DriverManager {
public static final String CHROME_BROWSER = "chrome";
public static final String BRAVE_BROWSER = "brave";
public static final String CHROMIUM_BROWSER = "chromium";
public static final String FIREFOX_BROWSER = "firefox";
public static final String IE_BROWSER = "ie";
public static final String MSEDGE_BROWSER = "msedge";
public static final String OPERA_BROWSER = "opera";
public static final String SAFARI_BROWSER = "safari";
public static final String SAP_DRIVER = "sap";
private ArrayList mobileDrivers = new ArrayList<>();
//--------------------------------------------------------------------------------------------------------------
public IDriverEngine getDriverEngine(Channel channel, ActionStatus status, SystemDriver systemDriver, ActionTestScript script) {
final String application = channel.getApplication();
final ApplicationProperties props = AtsSingleton.getInstance().getApplicationProperties(application);
final String appName = props.getName().toLowerCase();
boolean enableAtsLearning = true;
if (CHROME_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new ChromeDriverEngine(channel, status, systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else if (MSEDGE_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new MsEdgeDriverEngine(channel, status, systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else if (BRAVE_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new BraveDriverEngine(channel, status, systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else if (OPERA_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new OperaDriverEngine(channel, status, systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else if (FIREFOX_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new FirefoxDriverEngine(channel, status, systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else if (SAFARI_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new SafariDriverEngine(channel, status, systemDriverInfo, systemDriver, props, enableAtsLearning);
} else {
return null;
}
} else if (IE_BROWSER.equals(appName)) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new IEDriverEngine(channel, status, systemDriverInfo, systemDriver, props, enableAtsLearning);
} else {
return null;
}
} else if (CHROMIUM_BROWSER.equals(appName)) {
if (props.getDriver() != null && props.getUri() != null) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, appName, props.getUri(), script);
if (status.isPassed()) {
return new ChromiumDriverEngine(channel, status, props.getName(), systemDriverInfo, systemDriver, props, AtsSingleton.getInstance().getProxy(), enableAtsLearning);
} else {
return null;
}
} else {
status.setError(ActionStatus.CHANNEL_START_ERROR, "missing Chromium properties ('path' and 'driver') in .atsProperties file");
return null;
}
}else {
enableAtsLearning = false; //TODO change when atsLearning is ready for other types of channels
if (props.isSap() || application.toLowerCase().startsWith(Channel.SAP + "://")) {
IDriverInfo systemDriverInfo = getSystemDriverInfo(status, systemDriver, SAP_DRIVER, props.getUri(), script);
if (status.isPassed()) {
return new SapDriverEngine(channel, status, application, systemDriverInfo, systemDriver, props, enableAtsLearning);
} else {
return null;
}
} else if (channel instanceof MobileChannel) {
final String token = getChannelToken(application);
final MobileDriverEngine mobileDriverEngine =
new MobileDriverEngine(
(MobileChannel)channel,
status,
application,
new DriverInfo(application, script),
systemDriver,
props,
token,
enableAtsLearning);
if (status.isPassed()) {
mobileDrivers.add(mobileDriverEngine);
}
if (channel instanceof MobileChromeChannel) {
return new MobileChromeDriverEngine(
(MobileChromeChannel)channel,
status,
new DriverInfoMobile(application),
systemDriver,
mobileDriverEngine,
props,
enableAtsLearning);
} else {
return mobileDriverEngine;
}
} else if (props.isApi() || application.startsWith(Channel.HTTP + "://") || application.startsWith(Channel.HTTPS + "://")) {
return new ApiDriverEngine(
channel,
status,
application,
new DriverInfoApi(application),
systemDriver,
props,
script,
enableAtsLearning);
} else if (Channel.DESKTOP_EXPLORER.equals(appName)) {
return new ExplorerDriverEngine(
channel,
status,
new DriverInfoDesktop(appName, Channel.DESKTOP_EXPLORER),
systemDriver,
props,
enableAtsLearning);
}
return new SystemDriverEngine(
channel,
status,
application,
new DriverInfoDesktop(appName, Channel.DESKTOP),
systemDriver,
props,
enableAtsLearning);
}
}
private String getChannelToken(String applicationPath) {
final int start = applicationPath.indexOf("://");
if(start > -1) {
applicationPath = applicationPath.substring(start + 3);
}
final String[] appData = applicationPath.split("/");
final String endPoint = appData[0];
for (MobileDriverEngine engine: mobileDrivers)
{
if (engine.getEndPoint().equals(endPoint)) {
mobileDrivers.remove(engine);
return engine.getToken();
}
}
return null;
}
private DriverInfo getSystemDriverInfo(ActionStatus status, SystemDriver sysDriver, String driverName, String appPath, ActionTestScript script) {
final RemoteDriverInfo remoteDriver = sysDriver.getRemoteDriver(status, driverName, appPath);
if(remoteDriver != null && remoteDriver.getDriverUri() != null) {
status.setNoError();
return new DriverInfo(driverName, remoteDriver, script);
}else if(status.isPassed()) {
status.setError(ActionStatus.CHANNEL_START_ERROR, "unable to get remote driver url -> " + driverName);
}
return null;
}
public void tearDown(){
while(mobileDrivers.size() > 0) {
mobileDrivers.remove(0).tearDown();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy