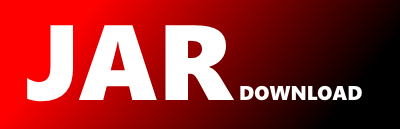
com.ats.executor.drivers.desktop.DesktopResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers.desktop;
import java.beans.Transient;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import com.ats.element.AtsBaseElement;
import com.ats.element.AtsElement;
import com.ats.element.FoundElement;
import com.ats.element.JsonUtils;
import com.ats.executor.TestBound;
import com.ats.generator.variables.CalculatedProperty;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.databind.JsonNode;
public class DesktopResponse {
private List elements;
private List windows;
private List attributes;
private int errorCode = 0;
private String errorMessage = null;
private byte[] image;
public DesktopResponse() {}
public DesktopResponse(String error) {
this.errorMessage = error;
this.errorCode = -999;
}
public DesktopResponse(JsonNode node) {
jsonDeserialize(node);
}
//-------------------------------------------------------------------------------------------------------
// getters and setters for serialization
//-------------------------------------------------------------------------------------------------------
@JsonGetter("elements")
public List getElements() {
return elements;
}
@JsonSetter("elements")
public void setElements(List elements) {
this.elements = elements;
}
@JsonGetter("windows")
public List getWindows() {
if(windows == null) {
return Collections.emptyList();
}
return windows;
}
@JsonSetter("windows")
public void setWindows(List windows) {
this.windows = windows;
}
@JsonGetter("attributes")
public List getAttributes() {
return attributes;
}
@JsonSetter("attributes")
public void setAttributes(List data) {
this.attributes = data;
}
@JsonGetter("errorCode")
public int getErrorCode() {
return errorCode;
}
@JsonSetter("errorCode")
public void setErrorCode(int errorCode) {
this.errorCode = errorCode;
}
@JsonGetter("errorMessage")
public String getErrorMessage() {
return errorMessage;
}
@JsonSetter("errorMessage")
public void setErrorMessage(String errorMessage) {
this.errorMessage = errorMessage;
}
@JsonGetter("image")
public byte[] getImage() {
return image;
}
@JsonSetter("image")
public void setImage(byte[] image) {
this.image = image;
}
//-------------------------------------------------------------------------------------------------------
public List getData(){
if(attributes == null || attributes.size() == 0) {
return Collections.emptyList();
}
return attributes;
}
@Transient
public List getFoundElements(TestBound channelDimension) {
if(elements == null) {
return Collections.emptyList();
}
return elements.stream().map(e -> new FoundElement(e, channelDimension)).collect(Collectors.toCollection(ArrayList::new));
}
/*@Transient
public List getData() {
if(data == null) {
return Collections.emptyList();
}
return data;
}*/
/*@Transient
public List getWindows() {
if(windows == null) {
return Collections.emptyList();
}
return windows;
}*/
@Transient
public DesktopWindow getWindow() {
if(windows != null && windows.size() > 0) {
return windows.get(0);
}
return null;
}
@Transient
public FoundElement getParentsElement(TestBound dimension) {
FoundElement current = null;
if(elements != null) {
for(Object obj : elements) {
final FoundElement elem = new FoundElement((AtsElement)obj, dimension);
elem.setParent(current);
current = elem;
}
}
return current;
}
@Transient
public CalculatedProperty[] getElementAttributes() {
if(attributes == null || attributes.size() == 0) {
return new CalculatedProperty[0];
}
CalculatedProperty[] result = new CalculatedProperty[attributes.size()];
int loop = 0;
for (DesktopData data : attributes) {
result[loop] = data.getCalculatedProperty();
loop++;
}
return result;
}
@Transient
public List getFoundElements(Predicate predicate, TestBound dimension) {
if(elements == null) {
return Collections.emptyList();
}
return elements.parallelStream().filter(predicate).map(e -> new FoundElement(e, dimension)).collect(Collectors.toCollection(ArrayList::new));
}
@Transient
public String getFirstAttribute() {
if(attributes != null && attributes.size() > 0) {
return attributes.get(0).getValue();
}
return null;
}
public void jsonDeserialize(JsonNode node){
// DesktopResponse result = new DesktopResponse();
if(node != null) {
if(node.get("error") != null && node.get("error").asInt() != 0) {
setErrorCode(node.get("error").asInt());
setErrorMessage(node.get("message").asText());
}
else if(node.get("errorCode") != null && node.get("errorCode").asInt() != 0) {
setErrorCode(node.get("errorCode").asInt());
setErrorMessage(node.get("errorMessage").asText());
}
else {
elements=new ArrayList<>();
JsonNode jsonElements = node.get("elements");
if(jsonElements != null) {
if(jsonElements.isArray() ) {
for(JsonNode jsonElement : jsonElements){
AtsElement atsElement = new AtsElement(jsonElement);
elements.add(atsElement) ;
}
}
else elements = null;
}
else elements = null;
windows=new ArrayList<>();
JsonNode jsonWindows = node.get("windows");
if(jsonWindows != null) {
if(jsonWindows.isArray() ) {
for(JsonNode jsonWindow : jsonWindows){
DesktopWindow desktopWindow = new DesktopWindow(jsonWindow);
windows.add(desktopWindow);
}
}
else windows = null;
}
else windows = null;
attributes=new ArrayList<>();
JsonNode jsonAttributes = node.get("attributes");
if(jsonAttributes != null) {
if(jsonAttributes.isArray()) {
for(JsonNode jsonAttribute : jsonAttributes){
DesktopData desktopData = new DesktopData(jsonAttribute);
attributes.add(desktopData) ;
}
}
else attributes = null;
}
else attributes = null;
if(node.has("image") ){
image = JsonUtils.getBase64Image(node,"image");
}
else image = null;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy