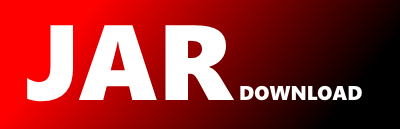
com.ats.executor.drivers.desktop.DesktopWindow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers.desktop;
import com.ats.element.AtsElement;
import com.ats.element.JsonUtils;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.NullNode;
public class DesktopWindow extends AtsElement {
private int pid = -1;
private int handle = -1;
private AppData app;
public DesktopWindow() {
super();
}
public DesktopWindow(JsonNode node) {
jsonDeserialize(node);
}
//-----------------------------------------------------------------------------------------------------------------
// getter setter for serialization
//-----------------------------------------------------------------------------------------------------------------
@JsonGetter("pid")
public int getPid() {
return pid;
}
@JsonSetter("pid")
public void setPid(int pid) {
this.pid = pid;
}
@JsonGetter("handle")
public int getHandle() {
return handle;
}
@JsonSetter("handle")
public void setHandle(int handle) {
this.handle = handle;
}
@JsonProperty("app")
public AppData getApp() {
return app;
}
@JsonProperty("app")
public void setApp(AppData app) {
this.app = app;
}
//-----------------------------------------------------------------------------------------------------------------
@JsonGetter("appPath")
public String getAppPath() {
if(app != null) {
return app.getPath();
}
return "";
}
@JsonGetter("appIcon")
public byte[] getAppIcon() {
if(app != null) {
return app.getIcon();
}
return new byte[1];
}
@JsonGetter("appName")
public String getAppName() {
if(app != null) {
return app.getName();
}
return "";
}
@JsonGetter("appVersion")
public String getAppVersion() {
if(app != null) {
return app.getVersion();
}
return "";
}
@JsonGetter("appBuildVersion")
public String getAppBuildVersion() {
if(app != null) {
return app.getBuild();
}
return "";
}
public static DesktopWindow createWindow(JsonNode node) {
final DesktopWindow win = new DesktopWindow();
if(node instanceof NullNode || node.get("error") != null) {
return win;
}
win.setId(node.get("id").asText());
win.setPid(node.get("pid").asInt(-1));
win.setHandle(node.get("handle").asInt(-1));
win.setTag(node.get("tag").asText());
win.setWidth(node.get("width").asDouble(0.0));
win.setHeight(node.get("height").asDouble(0.0));
win.setX(node.get("x").asDouble(0.0));
win.setY(node.get("y").asDouble(0.0));
final JsonNode obj = node.get("app");
if(obj != null) {
final ObjectMapper mapper = new ObjectMapper();
win.setApp(mapper.convertValue(obj, AppData.class));
}
return win;
}
@Override
public void jsonDeserialize(JsonNode node){
super.jsonDeserialize(node);
DesktopWindow defaultValue = new DesktopWindow();
if(node instanceof NullNode || node.get("error") != null) { return ; }
setPid(JsonUtils.getJsonValue(node, "pid", defaultValue.pid, Integer.class));
setHandle(JsonUtils.getJsonValue(node, "handle", defaultValue.handle, Integer.class));
JsonNode appData = node.get("app");
if(appData != null) {
setApp(new AppData(appData));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy