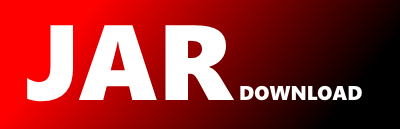
com.ats.executor.drivers.engines.browsers.BrowserArgumentsParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers.engines.browsers;
import java.util.ArrayList;
import com.ats.driver.ApplicationProperties;
import com.ats.executor.drivers.DriverManager;
import com.ats.executor.drivers.IDriverInfo;
import com.ats.executor.drivers.desktop.SystemDriver;
import com.ats.generator.variables.CalculatedValue;
public class BrowserArgumentsParser {
private final static String INCOGNITO_OPTION = "incognito";
private final static String PRIVATE_OPTION = "private";
private final static String IN_PRIVATE_OPTION = "inprivate";
private final static String HEADLESS_OPTION = "headless";
private final static String PROFILE_OPTION = "profile";
private final static String LANG_OPTION = "lang";
private final static String MAC_BRAVE_BROWSER_PATH = "/Applications/Brave Browser.app/Contents/MacOS/Brave Browser";
private String userDataPath;
private String binaryPath;
private boolean incognito = false;
private boolean headless = false;
private String lang;
private String[] moreOptions = null;
public BrowserArgumentsParser(
IDriverInfo driverInfo,
ArrayList arguments,
ApplicationProperties props,
String browserName,
String binary,
SystemDriver systemDriver) {
final ArrayList args = new ArrayList<>();
boolean firefoxLinux = false;
boolean braveMacOs = false;
if(DriverManager.FIREFOX_BROWSER.equals(browserName)) {
if(binary == null && driverInfo.getApplicationPath() != null) {
binary = driverInfo.getApplicationPath();
}
if(systemDriver.isLinux()) {
firefoxLinux = true;
}
}else if(DriverManager.BRAVE_BROWSER.equals(browserName) && systemDriver.getOsName().toLowerCase().contains("mac")) {
braveMacOs = true;
}
for (CalculatedValue calcv : arguments) {
final String arg = calcv.getCalculated();
if(arg.startsWith(PROFILE_OPTION)) {
final String profile = arg.replace(PROFILE_OPTION, "").replace("=", "").trim();
userDataPath = ApplicationProperties.getUserDataPath(profile, browserName, systemDriver);
}else if(arg.startsWith(LANG_OPTION)) {
lang = arg.replace(LANG_OPTION, "").replace("=", "").trim();
}else if(arg.contains(HEADLESS_OPTION)) {
headless = true;
}else if(arg.contains(INCOGNITO_OPTION) || arg.contains(PRIVATE_OPTION) || arg.contains(IN_PRIVATE_OPTION)) {
incognito = true;
}else {
args.add(arg);
}
}
if(props.getLang() != null) {
lang = props.getLang();
}
if(userDataPath == null) {
if (firefoxLinux)
userDataPath = ApplicationProperties.getUserDataPath("default", browserName, systemDriver);
else
userDataPath = ApplicationProperties.getUserDataPath(props.getUserDataDir(), browserName, systemDriver);
}
if(binary != null) {
binaryPath = binary;
}
if(braveMacOs){
binaryPath=MAC_BRAVE_BROWSER_PATH;
}
//force headless when interactive mode
if(!headless && !systemDriver.isInteractive()) headless=true;
if(props.getOptions() != null) {
for (String s: props.getOptions()) {
if(s.length() > 0) {
if(INCOGNITO_OPTION.equals(s) || PRIVATE_OPTION.equals(s)) {
incognito = true;
}else if(s.contains(HEADLESS_OPTION)) {
headless = true;
}else if(s.length() > 0) {
args.add(s);
}
}
}
}
if(args.size() > 0) {
moreOptions = args.toArray(new String[args.size()]);
}
driverInfo.setHeadless(headless);
}
public String getBinaryPath() {
return binaryPath;
}
public String[] getMoreOptions() {
if(moreOptions == null) {
return new String[0];
}
return moreOptions;
}
public String getUserDataPath() {
return userDataPath;
}
public boolean isIncognito() {
return incognito;
}
public boolean isHeadless() {
return headless;
}
public String getLang() {
return lang;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy