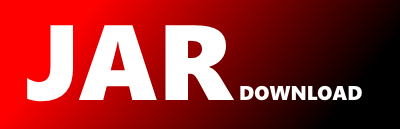
com.ats.executor.drivers.engines.browsers.IEDriverEngine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers.engines.browsers;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.Set;
import org.openqa.selenium.ie.InternetExplorerOptions;
import org.testng.collections.Sets;
import com.ats.driver.ApplicationProperties;
import com.ats.element.FoundElement;
import com.ats.element.test.TestElement;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.SendKeyData;
import com.ats.executor.channels.Channel;
import com.ats.executor.drivers.IDriverInfo;
import com.ats.executor.drivers.desktop.SystemDriver;
import com.ats.executor.drivers.engines.WebDriverEngine;
import com.ats.generator.objects.MouseDirection;
import com.ats.tools.ResourceContent;
public class IEDriverEngine extends WebDriverEngine {
private final Set windows = Sets.newLinkedHashSet();
public IEDriverEngine(Channel channel, ActionStatus status, IDriverInfo driverInfo, SystemDriver systemDriver, ApplicationProperties props, boolean enableLearning) {
super(channel, driverInfo, systemDriver, props, enableLearning);
JS_ELEMENT_PARENTS = ResourceContent.getParentElementJavaScriptIE();
JS_ELEMENT_FROM_POINT = "var result=null;var parent=document;var e=parent.elementFromPoint(arguments[1],arguments[2]);if(e && e!=arguments[0]){var r=e.getBoundingClientRect();result=[e, e.tagName, e.getAttribute('inputmode')=='numeric', e.getAttribute('type')=='password', r.x+0.0001, r.y+0.0001, r.width+0.0001, r.height+0.0001, r.left+0.0001, r.top+0.0001, 0.0001, 0.0001, {},false];};";
//JS_SCROLL_IF_NEEDED = "var e=arguments[0], bo=arguments[1], result=[];var r=e.getBoundingClientRect();if(r.top < 0 || r.left < 0 || r.bottom > (window.innerHeight || document.documentElement.clientHeight) || r.right > (window.innerWidth || document.documentElement.clientWidth)) {e.scrollIntoView(true);r=e.getBoundingClientRect();result=[r.left+0.0001, r.top+0.0001];}";
//final InternetExplorerOptions ieOptions = new InternetExplorerOptions();
/*if(props.getOptions() != null && props.getOptions().length > 0) {
addOptions(props.getOptions(), ieOptions);
}else {
ieOptions.introduceFlakinessByIgnoringSecurityDomains();
ieOptions.ignoreZoomSettings();
}*/
final InternetExplorerOptions ieOptions = new InternetExplorerOptions();
ieOptions.attachToEdgeChrome();
ieOptions.withEdgeExecutablePath(systemDriver.getMsEdgePath());
ieOptions.setCapability("ignoreProtectedModeSettings", true);
//ieOptions.introduceFlakinessByIgnoringSecurityDomains();
//ieOptions.ignoreZoomSettings();
launchDriver(status, ieOptions);
if(status.isPassed() && !"11".equals(channel.getApplicationVersion())) {
status.setError(ActionStatus.CHANNEL_START_ERROR, "cannot start channel with IE" + channel.getApplicationVersion() + " (Only IE11 is supported by ATS)");
}
executeToFront();
}
@Override
public void setWindowToFront() {
super.setWindowToFront();
executeToFront();
}
private void executeToFront() {
try {
driver.executeAsyncScript("var callback=arguments[arguments.length-1];var result=setTimeout(function(){window.focus();},1000);callback(result);");
}catch (Exception e) {}
}
@Override
public void scroll(FoundElement element) {
try {
super.scroll(element);
}catch(Exception e) {}
}
@Override
public void drag(ActionStatus status, FoundElement element, MouseDirection position, int offsetX, int offsetY, boolean offset) {
desktopMoveToElement(element, position, (int)(element.getWidth()/2), (int)(element.getHeight()/2) - 8);
getSystemDriver().mouseDown();
}
@Override
public void drop(FoundElement element, MouseDirection md, boolean desktopDriver) {
getSystemDriver().mouseRelease();
}
@Override
public void middleClick(ActionStatus status, MouseDirection position, TestElement element) {
getSystemDriver().mouseMiddleClick();
}
@Override
public void doubleClick() {
getSystemDriver().doubleClick();
}
@Override
public void sendTextData(ActionStatus status, TestElement element, ArrayList textActionList, int waitSearch, ActionTestScript topScript) {
if(element.isNumeric()) {
for(SendKeyData sequence : textActionList) {
element.executeScript(status, "value='" + sequence.getData() + "'", true);
}
}else {
try {
for(SendKeyData sequence : textActionList) {
element.getWebElement().sendKeys(sequence.getSequenceWeb(true));
}
}catch(Exception e) {
status.setError(ActionStatus.ENTER_TEXT_FAIL, e.getMessage());
}
}
}
@Override
public boolean switchWindow(ActionStatus status, int index, int tries, boolean refresh) {
super.switchWindow(status, index, tries, refresh);
if(status.isPassed()) {
executeJavaScript(status, "setTimeout(function(){window.focus();},1000);", true);
channel.setWindowToFront();
executeToFront();
channel.sleep(2000);
getSystemDriver().updateWindowHandle(channel);
}
return true;
}
@Override
protected Set getDriverWindowsList() {
final Set driverList = super.getDriverWindowsList();
driverList.parallelStream().forEach(s -> windows.add(s));
for (Iterator iterator = windows.iterator(); iterator.hasNext();) {
if(!driverList.contains(iterator.next())) {
iterator.remove();
}
}
return windows;
}
/*@Override
protected Object runJavaScript(ActionStatus status, String javaScript, Object... params) {
status.setPassed(true);
try {
return driver.executeAsyncScript(javaScript + ";arguments[arguments.length-1](result);", params);
}catch(StaleElementReferenceException e0) {
status.setPassed(false);
throw e0;
}catch(Exception e1) {
status.setException(ActionStatus.JAVASCRIPT_ERROR, e1);
}
return null;
}*/
/*private void addOptions(String[] options, InternetExplorerOptions ieOptions) {
final ArrayList extra = new ArrayList<>();
for (String o : options) {
if("useShellWindowsApiToAttachToIe".equalsIgnoreCase(o)) {
ieOptions.useShellWindowsApiToAttachToIe();
}else if("enablePersistentHovering".equalsIgnoreCase(o)) {
ieOptions.enablePersistentHovering();
}else if("destructivelyEnsureCleanSession".equalsIgnoreCase(o)) {
ieOptions.destructivelyEnsureCleanSession();
}else if("disableNativeEvents".equalsIgnoreCase(o)) {
ieOptions.disableNativeEvents();
}else if("ignoreZoomSettings".equalsIgnoreCase(o)) {
ieOptions.ignoreZoomSettings();
}else if("useCreateProcessApiToLaunchIe".equalsIgnoreCase(o)) {
ieOptions.useCreateProcessApiToLaunchIe();
}else if("requireWindowFocus".equalsIgnoreCase(o)) {
ieOptions.requireWindowFocus();
}else if("usePerProcessProxy".equalsIgnoreCase(o)) {
ieOptions.usePerProcessProxy();
}else {
extra.add(o);
}
}
if(extra.size() > 0) {
ieOptions.addCommandSwitches(extra.toArray(new String[extra.size()]));
}
}*/
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy