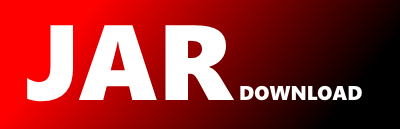
com.ats.executor.drivers.engines.mobiles.IosRootElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.executor.drivers.engines.mobiles;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
import java.util.TreeMap;
import java.util.UUID;
import javax.annotation.Nonnull;
import com.ats.data.Point;
import com.ats.data.Rectangle;
import com.ats.element.AtsMobileElement;
import com.ats.element.FoundElement;
import com.ats.element.MobileTestElement;
import com.ats.element.StructDebugDescription;
import com.ats.element.test.TestElement;
import com.ats.executor.ActionStatus;
import com.ats.executor.SendKeyData;
import com.ats.executor.drivers.engines.MobileDriverEngine;
import com.ats.generator.objects.MouseDirection;
import com.fasterxml.jackson.databind.JsonNode;
public class IosRootElement extends RootElement {
private final String regexSpaces = "^\\s+";
private final String regexBraces = "[{},]+";
private final double scale;
public IosRootElement(MobileDriverEngine driver, double scale) {
super(driver);
this.scale = scale;
}
@Override
public MobileTestElement getCurrentElement(FoundElement element, MouseDirection position) {
final Point point = calculateCoordinates(element, position);
return new MobileTestElement(element.getId(), point.x, point.y);
}
@Override
public void tap(ActionStatus status, FoundElement element, MouseDirection position) {
final Point point = calculateCoordinates(element, position);
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.TAP, point.x + "", point.y + "");
}
private Point calculateCoordinates(FoundElement element, MouseDirection position) {
final Rectangle rect = element.getRectangle();
final double offsetX;
final double offsetY;
double originX = element.getX();
double originY = element.getY();
if (element.getTag().equals("@IMAGE")) {
offsetX = driver.getOffsetX(rect, scale, position);
offsetY = driver.getOffsetY(rect, scale, position);
originX = originX / scale;
originY = originY / scale;
} else {
offsetX = driver.getOffsetX(rect, position);
offsetY = driver.getOffsetY(rect, position);
}
final int x = (int) (originX + offsetX);
final int y = (int) (originY + offsetY);
return new Point(x, y);
}
@Override
public void tap(FoundElement element, int count) {
if (element.getParent() != null) {
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.TAP, String.valueOf(count));
}
}
@Override
public void press(FoundElement element, ArrayList paths, int duration) {
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.PRESS, String.valueOf(paths), String.valueOf(duration));
}
@Override
public void swipe(MobileTestElement testElement, int hDirection, int vDirection) {
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.SWIPE, testElement.getOffsetX() + "", testElement.getOffsetY() + "", hDirection + "", vDirection + "");
}
@Override
public void textInput(TestElement element, ArrayList textActionList) {
for (SendKeyData sequence : textActionList) {
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.INPUT, sequence.getSequenceMobile());
}
}
@Override
public void clearInput(TestElement element) {
driver.executeRequest(MobileDriverEngine.ELEMENT, MobileDriverEngine.INPUT, SendKeyData.EMPTY_DATA);
}
@Override
public Object scripting(String script, FoundElement element) {
String coordinates = element.getX() + ";" + element.getY() + ";" + element.getWidth() + ";" + element.getHeight();
return driver.executeRequest(MobileDriverEngine.ELEMENT, element.getId(), MobileDriverEngine.SCRIPTING, "0", "0", coordinates, script);
}
@Override
public Object scripting(String script) {
return scripting(script, getValue().getFoundElement());
}
@Override
public void refresh(@Nonnull JsonNode node) {
final String debugDescription = node.get("root").asText();
final String[] debugDescriptionArray = debugDescription.split("\n");
double width = 0.0;
double height = 0.0;
final ArrayList elementInfoArray = new ArrayList<>();
for (String item : debugDescriptionArray) {
int level = countSpaces(item);
if (level >= 4 && !item.contains("Application, ")) {
// if (level >= 4 && !item.contains("Application, pid:")) {
String trimmedLine = item.replaceAll(regexSpaces, "");
if (trimmedLine.startsWith("Window (Main)")) {
String regexBracesAndSpaces = "[\\s{},]+";
final String[] arraySize = trimmedLine.split(regexBracesAndSpaces);
width = Double.parseDouble(arraySize[5]);
height = Double.parseDouble(arraySize[6]);
}
elementInfoArray.add(new StructDebugDescription((level / 2) - 1, trimmedLine));
}
}
final AtsMobileElement rootElement = new AtsMobileElement(UUID.randomUUID().toString(), "root", width, height, 0.0, 0.0, false, new HashMap<>());
int currentElementIndex = 0;
for (StructDebugDescription elementInfo : elementInfoArray) {
final String[] arraySize = elementInfo.getContent().split(regexBraces);
final String tag = arraySize[0].replaceAll(regexSpaces, "");
final ArrayList stringArray = new ArrayList<>();
for (String s : arraySize) {
if (!s.replaceAll(regexSpaces, "").equals("")) {
stringArray.add(s);
}
}
int firstSizeIndex = 2;
if (elementInfo.getContent().contains("pid:")) {
firstSizeIndex++;
}
final double currentX = Double.parseDouble(stringArray.get(firstSizeIndex));
final double currentY = Double.parseDouble(stringArray.get(firstSizeIndex + 1));
final double currentWidth = Double.parseDouble(stringArray.get(firstSizeIndex + 2));
final double currentHeight = Double.parseDouble(stringArray.get(firstSizeIndex + 3));
final AtsMobileElement element = new AtsMobileElement(elementInfo.getUuid().toString(), tag, currentWidth, currentHeight, currentX, currentY, true, getAttributes(elementInfo.getContent()));
if (elementInfo.getLevel() == 1) {
rootElement.addChildren(element);
} else {
// get parentLevel
int i = currentElementIndex;
UUID uuidParent = null;
while ((i - 1) > -1) {
if (elementInfoArray.get(i - 1).getLevel() + 1 == elementInfo.getLevel()) {
uuidParent = elementInfoArray.get(i - 1).getUuid();
break;
}
i--;
}
if (uuidParent != null) {
searchAndAdd(uuidParent.toString(), rootElement, element);
}
}
currentElementIndex++;
}
// Many Windows
final AtsMobileElement[] rootElementChildren = rootElement.getChildren();
if (rootElementChildren != null && rootElementChildren.length > 0) {
// Window children
final AtsMobileElement[] frontElements = rootElementChildren[0].getChildren();
if (frontElements != null && frontElements.length > 1) {
final AtsMobileElement lastElement = frontElements[frontElements.length - 1];
final boolean containsElements = checkConsistency(lastElement.getChildren(), false);
if (containsElements) {
rootElement.getChildren()[0].setChildren(lastElement.getChildren());
} else {
rootElement.getChildren()[0].setChildren(frontElements[0].getChildren());
}
}
}
this.value = rootElement;
}
public boolean checkConsistency(AtsMobileElement[] child, boolean val) {
for (AtsMobileElement atsMobileElement : child) {
if (atsMobileElement != null && atsMobileElement.getChildren().length > 0) {
val = checkConsistency(atsMobileElement.getChildren(), val);
} else {
if (atsMobileElement != null && !atsMobileElement.getTag().equalsIgnoreCase("other")) {
val = true;
}
}
}
return val;
}
/* public AtsMobileElement[] fixErrorsInDOM(AtsMobileElement domStructure) {
AtsMobileElement[] children = domStructure.getChildren();
for (int i = 0; i < children.length; i++) {
if (children[i] != null && children[i].getChildren().length > 0) {
children[i].setChildren(fixErrorsInDOM(children[i]));
if (children[i].getChildren().length == 0 && children[i].getTag().equalsIgnoreCase("other")) {
children = removeTheElement(children, i);
i--;
}
} else {
if (children[i] != null && children[i].getTag().equalsIgnoreCase("other")
&& children[i].getChildren().length == 0) {
children = removeTheElement(children, i);
i--;
}
}
}
if (children.length == 0) {
return null;
}
return children;
}
// Function to remove the element
public static AtsMobileElement[] removeTheElement(AtsMobileElement[] arr, int index) {
// If the array is empty
// or the index is not in array range
// return the original array
if (arr == null || index < 0 || index >= arr.length) {
return arr;
}
// Create another array of size one less
AtsMobileElement[] anotherArray = new AtsMobileElement[arr.length - 1];
// Copy the elements except the index
// from original array to the other array
for (int i = 0, k = 0; i < arr.length; i++) {
// if the index is
// the removal element index
if (i == index) {
continue;
}
// if the index is not
// the removal element index
anotherArray[k++] = arr[i];
}
// return the resultant array
return anotherArray;
} */
private Map getAttributes(String str) {
TreeMap result = new TreeMap<>();
String[] arraySize = str.split(regexBraces);
if (arraySize[0].contains("checkbox")) {
result.put("checkable", "true");
} else {
result.put("checkable", "false");
}
for (String s : arraySize) {
final String cleanString = cleanAttribute(s);
if (s.contains("label")) {
result.put("text", cleanString);
}
if (s.contains("placeholderValue")) {
result.put("description", cleanString);
}
if (s.contains("identifier")) {
result.put("identifier", cleanString);
}
if (s.contains("value")) {
result.put("value", cleanString);
}
if (s.contains("Disabled")) {
result.put("enabled", "false");
result.put("editable", "false");
}
if (s.contains("Selected")) {
result.put("selected", "true");
}
}
if (!result.containsKey("text")) {
result.put("text", "");
}
if (!result.containsKey("description")) {
result.put("description", "");
}
if (!result.containsKey("identifier")) {
result.put("identifier", "");
}
if (!result.containsKey("value")) {
result.put("value", "");
}
if (!result.containsKey("enabled")) {
result.put("enabled", "true");
result.put("editable", "true");
}
if (!result.containsKey("selected")) {
result.put("selected", "false");
}
if (!result.containsKey("numeric")) {
result.put("numeric", "false");
}
return result;
}
private String cleanAttribute(String str) {
return str.split(":")[str.split(":").length - 1].replace("'", "").replaceAll(regexSpaces, "");
}
private int countSpaces(String str) {
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == ' ') {
count++;
} else {
break;
}
}
return count;
}
private void searchAndAdd(String uuid, AtsMobileElement node, AtsMobileElement elementToAdd) {
if (node.getId().equals(uuid)) {
node.addChildren(elementToAdd);
} else {
final AtsMobileElement[] children = node.getChildren();
if (children != null) {
for (int i = 0; i < node.getChildren().length; i++) {
searchAndAdd(uuid, node.getChildren()[i], elementToAdd);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy