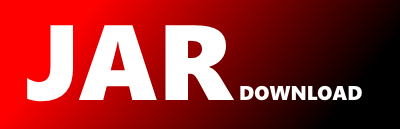
com.ats.executor.drivers.engines.webservices.RestApiExecutor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.executor.drivers.engines.webservices;
import java.io.File;
import java.io.PrintStream;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.Map.Entry;
import java.util.stream.Collectors;
import com.ats.executor.ActionStatus;
import com.ats.executor.channels.Channel;
import com.ats.generator.variables.CalculatedProperty;
import com.ats.generator.variables.CalculatedValue;
import com.ats.script.Script;
import com.ats.script.actions.ActionApi;
import okhttp3.MediaType;
import okhttp3.MultipartBody;
import okhttp3.OkHttpClient;
import okhttp3.Request.Builder;
import okhttp3.RequestBody;
public class RestApiExecutor extends ApiExecutor {
private static final String CONTENT_TYPE = "Content-Type";
//private static final int SLASH = 1;
private static final String APP_URL_ENCODED = "application/x-www-form-urlencoded";
private static final String MULTIPART_DATA = "multipart/form-data";
private static final String APP_JSON = "application/json";
private static final String APP_XML = "application/xml";
public RestApiExecutor(PrintStream logStream, AtsCookieJar cookieJar, OkHttpClient client, int timeout, int maxTry, Channel channel, String wsUrl) {
super(logStream, cookieJar, client, timeout, maxTry, channel);
if(!wsUrl.endsWith("/")) {
wsUrl += "/";
}
this.setUri(wsUrl);
}
private String getUrlEncodedData(CalculatedProperty p) {
final String key = p.getName();
if(key == null || key.isEmpty() || ActionApi.JSON_DATA.equals(key) || ActionApi.XML_DATA.equals(key)) {
return URLEncoder.encode(p.getValue().getCalculated(), StandardCharsets.UTF_8);
}else {
return URLEncoder.encode(p.getName(), StandardCharsets.UTF_8) + "=" + URLEncoder.encode(p.getValue().getCalculated(), StandardCharsets.UTF_8);
}
}
private void addDataPart(CalculatedProperty p, okhttp3.MultipartBody.Builder body, Script script) {
final String key = p.getName();
final CalculatedValue value = p.getValue();
value.uncrypt(script);
final String valueString = value.getCalculated();
if(key == null || key.isEmpty() || ActionApi.JSON_DATA.equals(key) || ActionApi.XML_DATA.equals(key)) {
if(!checkFileData(valueString, "file", body)) {
body.addFormDataPart("value", value.getCalculated());
}
}else {
if(!checkFileData(valueString, key, body)) {
body.addFormDataPart(key, valueString);
}
}
}
private boolean checkFileData(String value, String key, okhttp3.MultipartBody.Builder body) {
if(value.startsWith("file:///")) {
final File file = new File(value.substring(8));
if(file.exists()) {
final RequestBody fileBody = RequestBody.create(file, MediaType.parse("application/octet-stream; charset=utf-8"));
body.addFormDataPart(key, file.getName(), fileBody);
return true;
}
}
return false;
}
@Override
public void execute(ActionStatus status, final ActionApi api) {
super.execute(status, api);
if(api.getMethod().getCalculated().startsWith("file:///")){
loadLocalFile("json", api.getMethod().getCalculated());
return;
}
final String fullUri = getMethodUri().toString();
boolean addContentType = true;
boolean multipartData = false;
final Builder requestBuilder = new Builder();
for (Entry header : headerProperties.entrySet()) {
if(CONTENT_TYPE.equals(header.getKey())) {
addContentType = false;
if(header.getValue().contains(MULTIPART_DATA)){
multipartData = true;
}
}
requestBuilder.addHeader(header.getKey(), header.getValue());
}
final String apiType = api.getType().toUpperCase();
if(ActionApi.GET.equals(apiType) || ActionApi.DELETE.equals(apiType)) {
//final int dataType = 0;
String data = "";
if(api.getData() != null && api.getData().size() > 0) {
//if(dataType == SLASH) {
// data = "/" + api.getData().stream().map(p -> getUrlEncodedData(p)).collect(Collectors.joining("/"));
//}else {
data = "?" + api.getData().stream().map(p -> getUrlEncodedData(p)).collect(Collectors.joining("&"));
//}
}
requestBuilder.url(fullUri + data);
if(ActionApi.GET.equals(apiType)) {
requestBuilder.get();
}else {
requestBuilder.delete();
}
}else {
requestBuilder.url(fullUri);
RequestBody body = null;
if(api.getData() != null) {
final Script script = api.getScript();
if(api.getData().size() == 1 && !multipartData) {
final CalculatedProperty prop = api.getData().get(0);
prop.getValue().uncryptData(script);
if(addContentType) {
if(ActionApi.JSON_DATA.equals(prop.getName())) {
requestBuilder.addHeader(CONTENT_TYPE, APP_JSON);
}else if(ActionApi.XML_DATA.equals(prop.getName())) {
requestBuilder.addHeader(CONTENT_TYPE, APP_XML);
}else {
requestBuilder.addHeader(CONTENT_TYPE, APP_URL_ENCODED);
}
}
final CalculatedValue value = prop.getValue();
value.uncrypt(script);
body = RequestBody.Companion.create(value.getCalculated(), null);
}else {
final okhttp3.MultipartBody.Builder multipartBody = new MultipartBody.Builder().setType(MultipartBody.FORM);
api.getData().stream().forEach(p -> addDataPart(p, multipartBody, script));
if(addContentType) {
requestBuilder.addHeader(CONTENT_TYPE, MULTIPART_DATA);
}
body = multipartBody.build();
}
}
if(body == null) {
body = RequestBody.Companion.create("", null);
}
if(ActionApi.PATCH.equals(apiType)) {
requestBuilder.patch(body);
}else if(ActionApi.PUT.equals(apiType)) {
requestBuilder.put(body);
}else {
requestBuilder.post(body);
}
}
executeRequest(status, requestBuilder.build());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy