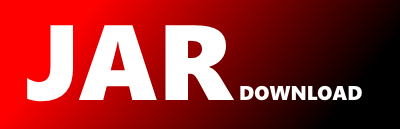
com.ats.generator.ATS Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.generator;
import java.io.File;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
import com.google.common.base.Charsets;
import com.google.common.io.CharStreams;
public class ATS {
public static final String WEB_SITE = "https://actiontestscript.org";
public static void logError(String msg) {
print("ERROR", msg);
}
public static void logInfo(String msg) {
print("INFO", msg);
}
public static void logWarn(String msg) {
print("WARN", msg);
}
private static void print(String type, String msg) {
System.out.println("[" + type + "] " + msg);
}
private static String atsVersion;
public static String getAtsVersion() {
if(atsVersion == null) {
InputStream resourceAsStream = ATS.class.getResourceAsStream("/com/ats/ats.properties");
try{
final String content = CharStreams.toString(new InputStreamReader(resourceAsStream, Charsets.UTF_8));
final Pattern pattern = Pattern.compile("version=(.*)", Pattern.CASE_INSENSITIVE);
final Matcher matcher = pattern.matcher(content);
if(matcher.find()) {
atsVersion = matcher.group(1);
}
}catch(Exception e) {
atsVersion = "N/A";
}
}
return atsVersion;
}
private static String atsServer;
public static String getAtsServer() {
if(atsServer == null) {
InputStream resourceAsStream = ATS.class.getResourceAsStream("/com/ats/ats.properties");
try{
final String content = CharStreams.toString(new InputStreamReader(resourceAsStream, Charsets.UTF_8));
final Pattern pattern = Pattern.compile("atsServer=(.*)", Pattern.CASE_INSENSITIVE);
final Matcher matcher = pattern.matcher(content);
if(matcher.find()) {
atsServer = matcher.group(1);
}
}catch(Exception e) {}
}
return atsServer;
}
private String[] args = null;
private Options options = new Options();
private File projectFolder = null;
private File destinationFolder = null;
private File reportFolder = null;
private File outputFolder = null;
private boolean compile = false;
public ATS(String[] args) {
this.args = args;
options.addOption("h", "help", false, "Show help");
options.addOption("f", "force", false, "Force Java files generation if files or folder exists");
options.addOption("comp", "compile", false, "Compile generated java files");
options.addOption("prj", "project", true, "ATS project folder");
options.addOption("dest", "destination", true, "Generated Java files destination folder");
options.addOption("rep", "report", true, "Execution report Java files destination folder");
options.addOption("suites", "suiteXmlFiles", true, "Execution suites to launch");
}
public void parse() {
final CommandLineParser parser = new DefaultParser();
CommandLine cmd = null;
try {
cmd = parser.parse(options, args);
} catch (ParseException e) {
ATS.logError("cannot parse command line : " + e.getMessage());
}
if(cmd != null) {
if (cmd.hasOption("h")) {
help();
}else {
final boolean force = cmd.hasOption("f");
compile = cmd.hasOption("comp");
String prjFolder = ".";
if (cmd.hasOption("prj")) {
prjFolder = cmd.getOptionValue("prj");
}
File file = new File(prjFolder);
if(file.exists()) {
projectFolder = new File(file.getAbsolutePath());
}else {
Path projectPath = Paths.get(prjFolder);
projectFolder = projectPath.toFile();
}
if(projectFolder.exists()) {
ATS.logInfo("using ATS project folder -> " + projectFolder.getAbsolutePath());
}else {
ATS.logError("project folder does not exists -> " + projectFolder.getAbsolutePath());
}
if (cmd.hasOption("dest")) {
destinationFolder = new File(cmd.getOptionValue("dest"));
if(destinationFolder.exists()) {
if(force) {
ATS.logWarn("destination folder exists ! (java files will be deleted)");
}else {
ATS.logError("destination folder exists, please delete folder or use '-force' option");
}
}
ATS.logInfo("using destination folder -> " + destinationFolder.getAbsolutePath());
}
if (cmd.hasOption("rep")) {
reportFolder = new File(cmd.getOptionValue("rep"));
if(reportFolder.exists()) {
if(force) {
ATS.logWarn("execution report folder found, it will be deleted");
}else {
ATS.logError("execution report folder exists, please delete folder or use '-force' option");
}
}
ATS.logInfo("using report folder : " + reportFolder.getAbsolutePath());
}
}
}
}
private void help() {
final HelpFormatter formater = new HelpFormatter();
formater.printHelp("ATS Java Code Generator", options);
}
//--------------------------------------------------------------------------------------------------------------------------------------------------------------------
public boolean isCompile() {
return compile;
}
public File getProjectFolder() {
return projectFolder;
}
public File getDestinationFolder() {
return destinationFolder;
}
public File getReportFolder() {
return reportFolder;
}
public File getOutputFolder() {
return outputFolder;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy