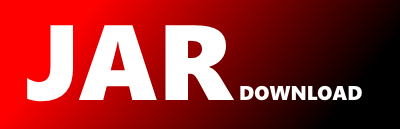
com.ats.recorder.TestSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.recorder;
import com.ats.executor.ScriptStatus;
import com.ats.executor.drivers.IDriverInfo;
import java.util.ArrayList;
import java.util.List;
public class TestSummary {
public static final String EMPTY_VALUE = "[empty]";
private int status = 1;
private String summary = "";
private String suiteName = "";
private String testName = "";
private int actions = 0;
private TestError error;
private List errors;
public void appendData(String value) {
summary += value + "
";
}
public void setFailData(String script, int line, String message, TestError.TestErrorStatus testErrorStatus) {
status = 0;
addError(script, line, message, testErrorStatus);
}
public void addFailPassData(String testName, int testLine, String errorMessage) {
status = 0;
addError(testName, testLine, errorMessage, TestError.TestErrorStatus.FAIL_PASS);
}
private void addError(String testName, int testLine, String errorMessage, TestError.TestErrorStatus testErrorStatus) {
if (errors == null) {
errors = new ArrayList<>();
errors.add(new TestError(testName, testLine, errorMessage, testErrorStatus));
} else {
if (TestError.TestErrorStatus.FAIL_STOP.equals(testErrorStatus) && errors.stream().anyMatch(testError -> TestError.TestErrorStatus.FAIL_STOP.equals(testError.getTestErrorStatus()))) {
return;
} else {
errors.add(new TestError(testName, testLine, errorMessage, testErrorStatus));
}
}
}
public RecorderSummaryData getRecordSummary(ScriptStatus st, IDriverInfo iDriverInfo) {
final RecorderSummaryData recordSummary = new RecorderSummaryData(st);
if (summary == null || summary.isEmpty()) summary = "";
recordSummary.setData(summary);
recordSummary.setStatus(status);
//if(status == 0) {
if (this.errors != null && !this.errors.isEmpty()) {
TestError multiError = new TestError(errors);
recordSummary.setError(multiError);
}
//}
recordSummary.setDriverInfo(iDriverInfo);
return recordSummary;
}
//--------------------------------------------------------
// getters and setters for serialization
//--------------------------------------------------------
public int getStatus() {
return status;
}
public void setStatus(int value) {
this.status = value;
}
public String getSummary() {
return summary;
}
public void setSummary(String value) {
if (value == null) {
value = "";
}
this.summary = value;
}
public String getSuiteName() {
return suiteName;
}
public void setSuiteName(String suiteName) {
this.suiteName = suiteName.replaceFirst("suite::", "");
}
public String getTestName() {
return testName;
}
public void setTestName(String testName) {
this.testName = testName.replaceFirst("script::", "");
}
public int getActions() {
return actions;
}
public void setActions(int actions) {
this.actions = actions;
}
public TestError getError() {
return error;
}
public void setError(TestError error) {
this.error = error;
}
public void setErrors(List errors) {
this.errors = errors;
}
public List getErrors() {
return this.errors;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy