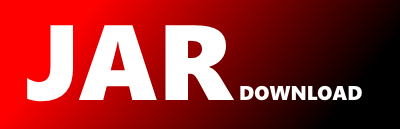
com.ats.script.ScriptInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.script;
import java.io.File;
import java.util.Date;
import java.util.List;
import com.ats.generator.parsers.Lexer;
import com.ats.script.actions.Action;
import com.ats.script.actions.ActionCallscript;
public class ScriptInfo {
private int actions = 0;
private int callscripts = 0;
private String nativePath;
private long size;
private ScriptHeader header;
public ScriptInfo(ScriptHeader h, File f) {
header = h;
size = f.length();
nativePath = f.getAbsolutePath();
}
public ScriptInfo(ScriptHeader header) {
this(header, new File(header.getPath()));
}
public ScriptInfo(Lexer lexer, File f) {
this(lexer.loadScript(f), f);
}
public ScriptInfo(ScriptLoader sc, File f) {
this(sc.getHeader(), f);
sc.getActions().parallelStream().forEach(this::count);
}
public ScriptInfo(Project project, File scriptFile) {
this(new ScriptHeader(project, scriptFile), scriptFile);
}
private void count(Action a) {
if(a instanceof ActionCallscript) {
callscripts++;
}else {
actions++;
}
}
//-------------------------------------------------------------------------------------------------
// getters and setters for serialization
//-------------------------------------------------------------------------------------------------
public int getActions() {
return actions;
}
public void setActions(int actions) {
this.actions = actions;
}
public int getCallscripts() {
return callscripts;
}
public void setCallscripts(int callscripts) {
this.callscripts = callscripts;
}
public String getAuthor() {
return header.getAuthor();
}
public void setAuthor(String author) {
header.setAuthor(author);
}
public String getDescription() {
return header.getDescription();
}
public void setDescription(String description) {
header.setDescription(description);
}
public String getId() {
return header.getId();
}
public void setId(String id) {
header.setId(id);
}
public List getGroups() {
return header.getGroups();
}
public void setGroups(List groups) {
header.setGroups(groups);
}
public String getPrerequisite() {
return header.getPrerequisite();
}
public void setPrerequisite(String prerequisite) {
header.setPrerequisite(prerequisite);
}
public Date getCreatedAt() {
return header.getCreatedAt();
}
public void setCreatedAt(Date createdAt) {
header.setCreatedAt(createdAt);
}
public Date getModifiedAt() {
return header.getModifiedAt();
}
public void setModifiedAt(Date modifiedAt) {
header.setModifiedAt(modifiedAt);
}
public String getModifiedBy() {
return header.getModifiedBy();
}
public void setModifiedBy(String name) {
header.setModifiedBy(name);
}
public String getName() {
return header.getName();
}
public void setName(String name) {
header.setName(name);
}
public String getPackageName() {
return header.getPackageName();
}
public void setPackageName(String packageName) {
header.setPackageName(packageName);
}
public long getSize() {
return size;
}
public void setSize(long size) {
this.size = size;
}
public String getExternalId() {
return header.getExternalId();
}
public void setExternalId(String id) {
header.setExternalId(id);
}
public String getNativePath() {
return nativePath;
}
public void setNativePath(String nativePath) {
this.nativePath = nativePath;
}
public String getError() {
return header.getError();
}
public void setError(String error) {
header.setError(error);
}
public String getMemo() {
return header.getMemo();
}
public void setMemo(String memo) {
header.setMemo(memo);
}
public String getPreprocessing() {
return header.getPreprocessing();
}
public void setPreprocessing(String preprocessing) {
header.setPreprocessing(preprocessing);
}
public String getPostprocessing() {
return header.getPostprocessing();
}
public void setPostprocessing(String postprocessing) {
header.setPostprocessing(postprocessing);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy