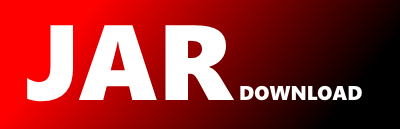
com.ats.script.ScriptLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.script;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.stream.Stream;
import org.ahocorasick.trie.Trie;
import com.ats.executor.ActionTestScript;
import com.ats.generator.GeneratorReport;
import com.ats.generator.parsers.Lexer;
import com.ats.generator.parsers.ScriptParser;
import com.ats.generator.variables.CalculatedValue;
import com.ats.generator.variables.Variable;
import com.ats.generator.variables.parameter.ParameterList;
import com.ats.script.actions.Action;
import com.ats.script.actions.ActionCallscript;
public class ScriptLoader extends Script {
public static final String SCRIPT_EXTENSION_ERROR = "ERR #198 - wrong file extension";
public static final String SCRIPT_LOADING_ERROR = "ERR #199 - the script cannot be loaded";
private ScriptParser parser;
private ScriptHeader header;
private String javaCode = null;
private Charset charset;
private ArrayList actions = new ArrayList<>();
private String projectGav = "";
private boolean update = false;
public ScriptLoader(){}
public ScriptLoader(Lexer lexer){
this.parser = new ScriptParser(lexer);
this.parser.addScript();
}
public ScriptLoader(String type, Lexer lexer, File file, Project projectData){
this(type, lexer, file, projectData, DEFAULT_CHARSET);
}
public ScriptLoader(String type, Lexer lexer, File file, Project prj, Charset charset){
final ScriptHeader header = new ScriptHeader(prj, file);
this.setHeader(header);
this.setCharset(charset);
this.projectGav = prj.getGav();
if(ATS_EXTENSION.equals(type)){
if(file.getName().endsWith(ATS_FILE_EXTENSION)) {
this.setParameterList(new ParameterList(1));
this.setVariables(new ArrayList<>());
this.parser = new ScriptParser(lexer);
this.parser.addScript();
try {
Stream lines = Files.lines(file.toPath(), this.charset);
lines
.map(String::trim)
.filter(a -> !a.isEmpty())
.filter(a -> !a.startsWith("["))
.forEach(a -> parser.parse(this, header, a));
lines.close();
} catch (Exception e) {
System.err.println("ATS load project error (" + SCRIPT_LOADING_ERROR + ") :\n" + file.getAbsolutePath());
header.setName(file.getName());
header.setPackageName("...");
header.setError(SCRIPT_LOADING_ERROR);
}
}else {
header.setName(file.getName());
header.setPackageName("...");
header.setError(SCRIPT_EXTENSION_ERROR);
}
}else if("java".equals(type)) {
try {
this.javaCode = new String(Files.readAllBytes(file.toPath()));
} catch (IOException e) {}
}
}
public ScriptLoader(Lexer lexer, String content, Project prj){
final ScriptHeader header = new ScriptHeader(prj);
this.setHeader(header);
this.setCharset(DEFAULT_CHARSET);
this.projectGav = prj.getGav();
this.setParameterList(new ParameterList(1));
this.setVariables(new ArrayList<>());
this.parser = new ScriptParser(lexer);
this.parser.addScript();
try {
parser.parse(this, header, content);
} catch (Exception e) {}
}
private void setCharset(Charset value) {
this.charset = value;
}
public void addAction(Action data, boolean disabled){
data.setDisabled(disabled);
data.setLine(actions.size());
actions.add(data);
}
public void addAction(Action data, boolean disabled, String atsCode){
addAction(data, disabled);
data.setCode(atsCode);
}
public boolean isSubscriptCalled(String scriptName) {
for (Action action : actions) {
if(action instanceof ActionCallscript) {
if(((ActionCallscript)action).isSubscriptCalled(scriptName)) {
return true;
}
}
}
return false;
}
public boolean getActionsKeywords(Trie trie) {
for (Action action : actions) {
final List actionKeywords = action.getKeywords();
for(String keywords : actionKeywords) {
if(trie.containsMatch(keywords)) {
return true;
}
}
}
return false;
}
public void updateSubscript(ArrayList result, String oldName, String newName) {
final ArrayList replacements = new ArrayList<>();
for(Action a : actions) {
if(a instanceof ActionCallscript) {
final ActionCallscript action = (ActionCallscript)a;
if(oldName.equals(action.getName().getData())) {
int start = a.getAtsCode().indexOf(oldName);
int end = start + oldName.length();
replacements.add(new String[] {a.getAtsCode(), a.getAtsCode().substring(0, start) + newName + a.getAtsCode().substring(end)});
}
}
}
saveUpdates(result, replacements);
}
public void updateDataFile(ArrayList result, String oldPath, String newPath) {
final ArrayList replacements = new ArrayList<>();
for(Action a : actions) {
if(a instanceof ActionCallscript) {
final ActionCallscript action = (ActionCallscript)a;
if(action.getParameterFilePath() != null && oldPath.equals(action.getParameterFilePath().getData())) {
replacements.add(new String[] {a.getAtsCode(), a.getAtsCode().replace(oldPath, newPath)});
}
}
}
saveUpdates(result, replacements);
}
private void saveUpdates(ArrayList result, ArrayList replacements) {
if(replacements.size() > 0) {
final ArrayList lines = new ArrayList<>();
final Path path = Paths.get(getHeader().getPath());
try {
Files.lines(path).forEach(l -> lines.add(l));
} catch (IOException e) {}
int i = 0;
String[] replace = replacements.remove(0);
for (String line : lines) {
if(line.equals(replace[0])) {
lines.set(i, replace[1]);
if(replacements.size() > 0) {
replace = replacements.remove(0);
}
}
i++;
}
try {
Files.write(path, lines, Charset.defaultCharset());
} catch (IOException e) {}
result.add(getHeader().getQualifiedName());
}
}
//---------------------------------------------------------------------------------------------------------------------------------
// Java Code Generator
//---------------------------------------------------------------------------------------------------------------------------------
public String getJavaCode(Project project){
if(javaCode != null) {
return javaCode;
}else {
final StringBuilder code = new StringBuilder(header.getJavaCode(project));
//-------------------------------------------------------------------------------------------------
// variables
//-------------------------------------------------------------------------------------------------
code.append("\r\n\r\n\t\t// ---< Variables >--- //\r\n");
final List variables = getVariables();
Collections.sort(variables);
for(Variable variable : variables){
code.append("\r\n\t\t")
.append(variable.getJavaCode())
.append(";");
}
//-------------------------------------------------------------------------------------------------
// actions
//-------------------------------------------------------------------------------------------------
code.append("\r\n\r\n\t\t// ---< Actions >--- //\r\n");
for(Action action : actions){
if(!action.isDisabled() && !action.isScriptComment()){
code.append("\r\n\t\t").append(action.getJavaCode()).append(");");
}
}
//-------------------------------------------------------------------------------------------------
// returns
//-------------------------------------------------------------------------------------------------
final CalculatedValue[] returnValues = getReturns();
if(returnValues != null) {
code.append("\r\n\r\n\t\t// ---< Return >--- //\r\n\r\n\t\t")
.append(ActionTestScript.JAVA_RETURNS_FUNCTION_NAME)
.append("(");
final ArrayList returnValuesCode = new ArrayList<>();
for(CalculatedValue ret : returnValues){
returnValuesCode.add(ret.getJavaCode());
}
code.append(String.join(", ", returnValuesCode)).append(");");
}
code.append("\r\n\t}\r\n}");
return code.toString();
}
}
public void generateJavaFile(Project project){
if(header.getJavaDestinationFolder() != null){
final File javaFile = header.getJavaFile();
try {
javaFile.getParentFile().mkdirs();
final BufferedWriter writer = new BufferedWriter(new OutputStreamWriter( new FileOutputStream(javaFile, false), charset));
writer.write(getJavaCode(project));
writer.close();
} catch (IOException e) {}
}
}
//-------------------------------------------------------------------------------------------------
// getters and setters for serialization
//-------------------------------------------------------------------------------------------------
public boolean getUpdate() {
return update;
}
public void setUpdate(boolean value) {
update = value;
}
public ScriptHeader getHeader() {
return header;
}
public void setHeader(ScriptHeader header) {
this.header = header;
}
public ArrayList getActions() {
return actions;
}
public void setActions(ArrayList value) {
this.actions = value;
}
public String getProjectGav() {
return projectGav;
}
public void setProjectGav(String value) {} // read only
//-------------------------------------------------------------------------------------------------
// transient getters and setters
//-------------------------------------------------------------------------------------------------
public Lexer getLexer() {
return parser.getLexer();
}
//----------------------------------------------------------------------------------------------------
// Script content java
//----------------------------------------------------------------------------------------------------
public static void main(String[] args) {
if (args.length == 1) {
final File scriptFile = new File(args[0]);
if (scriptFile.exists() && scriptFile.isFile() && scriptFile.getName().toLowerCase().endsWith(ATS_EXTENSION)){
final Project projectData = Project.getProjectData(scriptFile, null, null);
final GeneratorReport report = new GeneratorReport();
final Lexer lexer = new Lexer(projectData, report, ScriptLoader.DEFAULT_CHARSET);
lexer.loadScript(scriptFile);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy