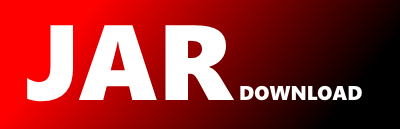
com.ats.script.actions.Action Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.script.actions;
import java.util.ArrayList;
import com.ats.recorder.TestError;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import com.ats.AtsSingleton;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.executor.channels.Channel;
import com.ats.recorder.IVisualRecorder;
import com.ats.recorder.VisualAction;
import com.ats.script.Script;
import com.ats.script.ScriptHeader;
import com.google.gson.JsonObject;
public abstract class Action {
public static final String DATA_JSON = "dataJson";
protected Script script;
protected ActionStatus status;
protected int line;
protected boolean disabled = false;
protected long timeLine;
private String code;
private Channel currentChannel;
//---------------------------------------------------------------------------------------------------------------------------------
// Constructors
//---------------------------------------------------------------------------------------------------------------------------------
public Action(){}
public Action(Script script){
this.script = script;
}
public void setCode(String value) {
code = value;
}
public String getAtsCode() {
return code;
}
public Element getXmlElement(Document document, ScriptHeader header, int index, String errorText) {
if(errorText == null) {
errorText = String.valueOf(ActionStatus.NO_ERROR);
}
final Element actionElem = document.createElement("action");
actionElem.setAttribute("index", String.valueOf(index));
actionElem.setAttribute("type", getClass().getName());
actionElem.appendChild(document.createElement("line")).setTextContent(String.valueOf(getLine()));
actionElem.appendChild(document.createElement("script")).setTextContent(header.getQualifiedName());
actionElem.appendChild(document.createElement("timeLine")).setTextContent(String.valueOf(timeLine));
actionElem.appendChild(document.createElement("error")).setTextContent(errorText);
actionElem.appendChild(document.createElement("stop")).setTextContent("true");
actionElem.appendChild(document.createElement("duration")).setTextContent("0");
actionElem.appendChild(document.createElement("passed")).setTextContent(String.valueOf(status.isPassed()));
Element img = document.createElement("img");
img.setAttribute("height", "100");
img.setAttribute("height", "150");
img.setAttribute("type", "png");
img.setAttribute("src", VisualAction.EMPTY_SCREEN);
actionElem.appendChild(img);
return actionElem;
}
public VisualAction getVisualAction() {
return new VisualAction(this, timeLine);
}
//---------------------------------------------------------------------------------------------------------------------------------
// Code Generator
//---------------------------------------------------------------------------------------------------------------------------------
public StringBuilder getJavaCode(){
StringBuilder codeBuilder = new StringBuilder(ActionTestScript.JAVA_EXECUTE_FUNCTION_NAME);
codeBuilder.append("(")
.append(getLine())
.append(",")
.append("new ")
.append(this.getClass().getSimpleName()).append("(this, ");
return codeBuilder;
}
public boolean isScriptComment() {
return false;
}
public ArrayList getKeywords() { return new ArrayList<>(); }
//---------------------------------------------------------------------------------------------------------------------------------
//---------------------------------------------------------------------------------------------------------------------------------
public boolean isPassed() {
return status.isPassed();
}
public long getExecutionDuration() {
return getStatus().getDuration();
}
public String execute(ActionTestScript ts, String testName, int line, int tryNum){
if(script == null) {
setScript(ts);
}
script.setLastAction(this);
execute(ts, testName, line, tryNum == 0);
return null;
}
protected void execute(ActionTestScript ts, String testName, int line, boolean firstTry){
this.line = line;
currentChannel = AtsSingleton.getInstance().getCurrentChannel();
currentChannel.newActionStatus(this, ts.getRecorder(), testName, line, firstTry);
}
public StringBuilder getActionLogs(String scriptName, int scriptLine, JsonObject data) {
if(!status.isPassed()) {
data = new JsonObject();
data.addProperty("status", "non blocking action");
data.addProperty("message", status.getFailMessage());
}
data.addProperty("passed", status.isPassed());
data.addProperty("duration", status.getDuration());
final JsonObject script = new JsonObject();
script.addProperty("name", scriptName);
script.addProperty("line", scriptLine);
data.add("script", script);
return new StringBuilder(getClass().getSimpleName()).append(" -> ").append(data.toString());
}
protected void saveAppSource() {
currentChannel.saveAppSource(script.getTestName());
}
public void failed(int errorCode, String errorMessage) {
status.setError(errorCode, errorMessage);
}
public void addShadowActionError(int line, TestError.TestErrorStatus testErrorStatus) {
if(getScript() != null) {
getScript().addShadowActionError(this, line, getStatus().getFailMessage(), testErrorStatus);
}
}
public void addShadowAction() {
if(getScript() != null) {
getScript().addShadowAction(this);
}
}
public void initAction(Channel channel, String testNameAndLine, IVisualRecorder recorder, String testName, int testLine) {
channel.startHarAction(this, testNameAndLine);
channel.createVisualAction(recorder, this, testName, testLine);
}
protected Channel getCurrentChannel() {
return currentChannel;
}
public void setTimeline(long value) {
this.timeLine = value;
}
//--------------------------------------------------------
// getters and setters for serialization
//--------------------------------------------------------
public boolean isDisabled() {
return disabled;
}
public void setDisabled(boolean disabled) {
this.disabled = disabled;
}
public int getLine() {
return line;
}
public void setLine(int line) {
this.line = line;
}
public Script getScript() {
return script;
}
public void setScript(Script script) {
this.script = script;
}
public ActionStatus getStatus() {
return status;
}
public void setStatus(ActionStatus status) {
this.status = status;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy