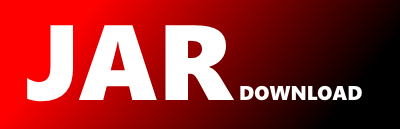
com.ats.script.actions.ActionAssertValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.script.actions;
import java.util.ArrayList;
import java.util.function.Predicate;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.generator.variables.CalculatedValue;
import com.ats.recorder.VisualAction;
import com.ats.script.Script;
import com.ats.script.ScriptLoader;
import com.ats.tools.Operators;
import com.google.gson.JsonObject;
public class ActionAssertValue extends ActionExecute {
public static final String SCRIPT_LABEL = "check-value";
public static final Predicate PREDICATE = g -> SCRIPT_LABEL.equals(g);
private CalculatedValue value1;
private CalculatedValue value2;
private Operators operatorExec = new Operators();
public ActionAssertValue() {}
public ActionAssertValue(ScriptLoader script, int stopPolicy, String compairData) {
super(script, stopPolicy);
final String[] operatorData = operatorExec.initData(compairData);
setValue1(new CalculatedValue(script, operatorData[0]));
setValue2(new CalculatedValue(script, operatorData[1]));
}
public ActionAssertValue(Script script, int stopPolicy, String operator, CalculatedValue value1, CalculatedValue value2) {
super(script, stopPolicy);
setOperator(operator);
setValue1(value1);
setValue2(value2);
}
@Override
public StringBuilder getActionLogs(String scriptName, int scriptLine, JsonObject data) {
data.addProperty("value1", value1.getCalculated());
data.addProperty("value2", value2.getCalculated());
return super.getActionLogs(scriptName, scriptLine, data);
}
//---------------------------------------------------------------------------------------------------------------------------------
// Code Generator
//---------------------------------------------------------------------------------------------------------------------------------
@Override
public StringBuilder getJavaCode() {
StringBuilder codeBuilder = super.getJavaCode();
codeBuilder.append(operatorExec.getJavaCode())
.append(", ")
.append(value1.getJavaCode())
.append(", ")
.append(value2.getJavaCode())
.append(")");
return codeBuilder;
}
@Override
public ArrayList getKeywords() {
final ArrayList keywords = super.getKeywords();
keywords.add(value1.getKeywords());
keywords.add(value2.getKeywords());
return keywords;
}
//---------------------------------------------------------------------------------------------------------------------------------
//---------------------------------------------------------------------------------------------------------------------------------
public void execute(ActionTestScript ts, String testName, int testLine) {
execute(ts, testName, testLine, 0);
}
@Override
public String execute(ActionTestScript ts, String testName, int testLine, int tryNum) {
super.execute(ts, testName, testLine, tryNum);
final String calculated1 = value1.getCalculated();
final String calculated2 = value2.getCalculated();
final String errorDescription = operatorExec.check(calculated1, calculated2);
if(errorDescription == null) {
status.setNoError(calculated1);
getCurrentChannel().addShadowAction(this, testLine);
}else {
final StringBuilder builder = new StringBuilder("Value1 '");
builder.append(calculated1)
.append("' ")
.append(errorDescription)
.append(" value2 '")
.append(calculated2)
.append("'");
status.setError(ActionStatus.VALUES_COMPARE_FAIL, builder.toString(), new String[]{calculated1, calculated2});
}
status.endDuration();
ts.getRecorder().update(status.getCode(), status.getDuration(), calculated1, operatorExec.getType() + " " + calculated2);
return null;
}
@Override
public VisualAction getVisualAction() {
return new VisualAction(this, timeLine);
}
//--------------------------------------------------------
// getters and setters for serialization
//--------------------------------------------------------
public CalculatedValue getValue1() {
return value1;
}
public void setValue1(CalculatedValue value1) {
this.value1 = value1;
}
public CalculatedValue getValue2() {
return value2;
}
public void setValue2(CalculatedValue value2) {
this.value2 = value2;
this.operatorExec.updatePattern(value2.getCalculated());
}
public String getOperator() {
return operatorExec.getType();
}
public void setOperator(String operator) {
this.operatorExec.setType(operator);
}
public static StringBuilder getAtsCodeStr(String subFolder) {
return new StringBuilder().append("callscript -> ").append(subFolder).append(".AssertValue");
// return new StringBuilder().append(SCRIPT_LABEL).append(" -> ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy