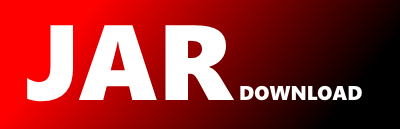
com.ats.script.actions.ActionPropertySet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.script.actions;
import java.util.ArrayList;
import java.util.function.Predicate;
import com.ats.executor.ActionTestScript;
import com.ats.generator.variables.CalculatedValue;
import com.ats.script.Script;
public class ActionPropertySet extends Action {
public static final String SCRIPT_LABEL = "property-set";
public static final Predicate PREDICATE = g -> SCRIPT_LABEL.equals(g);
private String name;
private CalculatedValue value;
public ActionPropertySet() { }
public ActionPropertySet(Script script, String name, String value) {
super(script);
setName(name);
setValue(new CalculatedValue(script, value));
}
public ActionPropertySet(Script script, String name, CalculatedValue value) {
super(script);
setName(name);
setValue(value);
}
//---------------------------------------------------------------------------------------------------------------------------------
//---------------------------------------------------------------------------------------------------------------------------------
@Override
public String execute(ActionTestScript ts, String testName, int line, int tryNum) {
super.execute(ts, testName, line, tryNum);
if (isPassed()) {
getCurrentChannel().setSysProperty(getName(), getValue().getCalculated());
status.endAction();
getCurrentChannel().sleep(50);
ts.getRecorder().updateScreen(false);
ts.getRecorder().update(status.getCode(), status.getDuration(), getName(), getValue().getCalculated());
}
return null;
}
//--------------------------------------------------------
// getters and setters for serialization
//--------------------------------------------------------
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public CalculatedValue getValue() { return value; }
public void setValue(CalculatedValue value) { this.value = value; }
//---------------------------------------------------------------------------------------------------------------------------------
// Code Generator
//---------------------------------------------------------------------------------------------------------------------------------
@Override
public StringBuilder getJavaCode() {
StringBuilder builder = super.getJavaCode();
builder.append("\"")
.append(name)
.append("\"")
.append(", ")
.append(value.getJavaCode())
.append(")");
return builder;
}
@Override
public ArrayList getKeywords() {
ArrayList keywords = super.getKeywords();
keywords.add(name);
keywords.add(value.getKeywords());
return keywords;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy