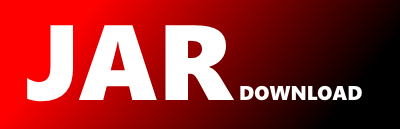
com.ats.tools.AtsClassLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.jar.Attributes;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
import java.util.jar.Manifest;
import com.ats.driver.AtsManager;
import com.ats.executor.ActionStatus;
import com.ats.executor.ActionTestScript;
import com.ats.tools.logger.MessageCode;
import com.ats.tools.wait.IWaitGuiReady;
import com.ats.tools.wait.WaitGuiReady;
import com.ats.tools.wait.WaitGuiReadyInfo;
import com.ats.tools.wait.WaitGuiReadyWithValues;
public class AtsClassLoader extends ClassLoader{
private ArrayList testClasses = new ArrayList();
public Class loadTestScriptClass(ActionStatus status, String name){
Class testScriptClass = loadAtsClass(name);
//last chance to find class
if(testScriptClass == null) {
for(String s : testClasses) {
if(name.equalsIgnoreCase(s)) {
return loadAtsClass(s);
}
}
status.setError(MessageCode.SCRIPT_NOT_FOUND, "ATS script not found -> " + name + "\n");
}
return testScriptClass;
}
@SuppressWarnings("unchecked")
private Class loadAtsClass(String name){
try {
return (Class) loadClass(name);
} catch (ClassNotFoundException e) {
return (Class) findClass(name);
}catch (Error | Exception e) {
return null;
}
}
@Override
public Class> findClass(String name) {
byte[] bt = loadAtsScriptClass(name);
if(bt != null) {
try {
return defineClass(name, bt, 0, bt.length);
}catch(NoClassDefFoundError e) {}
}
return null;
}
private byte[] loadAtsScriptClass(String className) {
final InputStream is = getClass().getClassLoader().getResourceAsStream(className.replace(".", "/") + ".class");
if(is != null) {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int len = 0;
try {
while((len = is.read())!=-1){
bos.write(len);
}
} catch (IOException e) {}
final byte[] data = bos.toByteArray();
try {
bos.close();
is.close();
} catch (IOException e) {}
return data;
}
return null;
}
//------------------------------------------------------------------------------------------------------
// Instance management
//------------------------------------------------------------------------------------------------------
private IWaitGuiReady waitGuiReady;
public IWaitGuiReady getWaitGuiReady() {
return waitGuiReady;
}
public AtsClassLoader(AtsManager atsManager) {
final Class wait = findCustomWaitClass();
if(wait != null) {
try {
waitGuiReady = wait.getDeclaredConstructor().newInstance();
} catch (InstantiationException | IllegalAccessException | IllegalArgumentException | InvocationTargetException | NoSuchMethodException | SecurityException e) {}
}
if(waitGuiReady == null) {
if(atsManager.getWaitEnterText() > 0 || atsManager.getWaitGotoUrl() > 0 || atsManager.getWaitMouseMove() > 0 || atsManager.getWaitSearch() > 0 || atsManager.getWaitSwitchWindow() > 0) {
waitGuiReady = new WaitGuiReadyWithValues(atsManager.getWaitEnterText(), atsManager.getWaitGotoUrl(), atsManager.getWaitMouseMove(), atsManager.getWaitSearch(), atsManager.getWaitSwitchWindow());
}else {
waitGuiReady = new WaitGuiReady();
}
}
ArrayList files = new ArrayList();
try {
Enumeration res = getClass().getClassLoader().getResources("");
while(res.hasMoreElements()){
URL url = res.nextElement();
if("file".equals(url.getProtocol())) {
files.add(url.getFile());
}
}
} catch (IOException e) {}
files.forEach(f -> walkFiles(f.length()-1, f));
}
private void walkFiles(int offset, String path) {
File root = new File(path);
File[] list = root.listFiles();
if (list == null) return;
for (File f : list) {
if (f.isDirectory()) {
walkFiles(offset, f.getAbsolutePath());
} else if(f.getName().endsWith(".class")) {
final String relativePath = f.getPath().substring(offset);
testClasses.add(relativePath.substring(0, relativePath.length()-6).replace(File.separator, "."));
}
}
}
private Class findCustomWaitClass() {
final String classpath = System.getProperty("java.class.path");
final String[] files = classpath.split(System.getProperty("path.separator"));
for (String path : files) {
final Class customWaitClass = findWaitGuiClass(path);
if(customWaitClass != null) {
return customWaitClass;
}
}
return null;
}
private Class findWaitGuiClass(String filePath){
File f = new File(filePath);
if(f.exists()) {
return findWaitGuiClass(f);
}
return null;
}
@SuppressWarnings("unchecked")
private Class findWaitGuiClass(File file) {
if(file.exists()) {
if (file.isDirectory()) {
for (File child : file.listFiles()) {
return findWaitGuiClass(child);
}
} else if (file.getName().toLowerCase().endsWith(".jar")) {
Class waitClass = null;
try {
JarFile jar = new JarFile(file);
final Enumeration entries = jar.entries();
final Manifest man = jar.getManifest();
if(man != null) {
Attributes attr = man.getMainAttributes();
if("WaitGuiReady".equals(attr.getValue("Ats-Type"))) {
while (entries.hasMoreElements()) {
final String entryName = entries.nextElement().getName();
if (entryName.endsWith(".class")) {
final String className = entryName.replace('/', '.').substring(0, entryName.length() - 6);
final Class> c = loadClass(className);
if(c.isAnnotationPresent(WaitGuiReadyInfo.class)) {
if(IWaitGuiReady.class.isAssignableFrom(c)) {
waitClass = (Class) c;
}
}
}
}
}
}
jar.close();
}catch(IOException | ClassNotFoundException e) {}
return waitClass;
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy