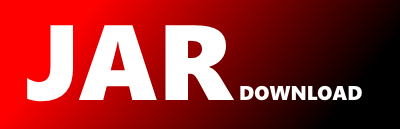
com.ats.tools.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools;
import com.ats.executor.ActionStatus;
import com.ats.generator.variables.parameter.ParameterDataFile;
import com.google.gson.JsonArray;
import com.google.gson.JsonParseException;
import com.google.gson.JsonParser;
import com.google.gson.JsonPrimitive;
import javax.net.ssl.HttpsURLConnection;
import java.io.*;
import java.lang.module.ModuleDescriptor.Version;
import java.net.*;
import java.nio.file.*;
import java.util.ArrayList;
import java.util.Base64;
import java.util.Collections;
import java.util.Comparator;
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class Utils {
private static final String WHITE_PIXEL = "iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAIAAACQd1PeAAAACXBIWXMAAC4jAAAuIwF4pT92AAAAB3RJTUUH5wYKDCwDjkpXLgAAABl0RVh0Q29tbWVudABDcmVhdGVkIHdpdGggR0lNUFeBDhcAAAAMSURBVAjXY/j//z8ABf4C/tzMWecAAAAASUVORK5CYII=";
private static final String VALID_JAVA_CHAR = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789_$";
public static final okhttp3.MediaType JSON_MEDIA = okhttp3.MediaType.get("application/json; charset=utf-8");
public static final okhttp3.MediaType TEXT_MEDIA = okhttp3.MediaType.get("text/plain");
public static String unescapeAts(String data) {
return data.replaceAll("&sp;", " ").replaceAll("&co;", ",").replaceAll("&eq;", "=").replaceAll("&rb;", "]").replaceAll("&lb;", "[");
}
public static String escapeHTML(String str) {
return str.codePoints().mapToObj(c -> c > 127 || "\"'<>&".indexOf(c) != -1 ?
"" + c + ";" : new String(Character.toChars(c)))
.collect(Collectors.joining());
}
public static boolean isNumeric(String numString) {
if (numString == null) {
return false;
}
try {
Double.parseDouble(numString);
} catch (NumberFormatException nfe) {
return false;
}
return true;
}
public static int string2Int(String value){
return string2Int(value, 0);
}
public static int string2Int(String value, int defaultValue){
try {
return Integer.parseInt(value.replaceAll("\\s", ""));
} catch (NullPointerException | NumberFormatException e) {
return defaultValue;
}
}
public static long string2Long(String value){
try {
return Long.parseLong(value.replaceAll("\\s", ""));
} catch (NullPointerException | NumberFormatException e) {
return 0L;
}
}
public static boolean isNotEmpty(String value){
return value != null && !value.isBlank();
}
public static double string2Double(String value){
try {
return Double.parseDouble(value.replaceAll("\\s", ""));
} catch (NullPointerException | NumberFormatException e) {
return 0D;
}
}
public static String truncateString(String value, int length)
{
if (value != null && value.length() > length)
value = value.substring(0, length);
return value;
}
public static JsonArray string2JsonArray(String value){
final char[] letters = value.toCharArray();
JsonArray array = new JsonArray();
for (final char ch : letters) {
array.add(new JsonPrimitive((int)ch));
}
return array;
}
public static String getShortUid() {
char[] chars = "ABSDEFGHIJKLMNOPQRSTUVWXYZ1234567890".toCharArray();
Random r = new Random(System.currentTimeMillis());
char[] id = new char[8];
for (int i = 0; i < 8; i++) {
id[i] = chars[r.nextInt(chars.length)];
}
return new String(id);
}
public static String checkJsonData(String jsonStr) {
if(jsonStr != null && jsonStr.length() > 0 && ((jsonStr.startsWith("{") && jsonStr.endsWith("}")) || (jsonStr.startsWith("[") && jsonStr.endsWith("]")))) {
try {
JsonParser.parseString(jsonStr) ;
return jsonStr;
} catch (JsonParseException e) {}
}
return null;
}
public static boolean isXmlLike(String inXMLStr) {
boolean retBool = false;
inXMLStr = inXMLStr.trim();
if(inXMLStr != null && inXMLStr.length() > 0 && inXMLStr.startsWith("<") && inXMLStr.endsWith(">")) {
Pattern pattern;
Matcher matcher;
final String XML_PATTERN_STR = "<(\\S+?)(.*?)>(.*?)(\\1>)?";
pattern = Pattern.compile(XML_PATTERN_STR,
Pattern.CASE_INSENSITIVE | Pattern.DOTALL | Pattern.MULTILINE);
matcher = pattern.matcher(inXMLStr);
retBool = matcher.matches();
}
return retBool;
}
public static String cleanHtmlCode(String value) {
return value.replaceAll("\\r?\\n", "").replaceAll(" ? ?", "");
}
//-------------------------------------------------------------------------------------------------------------------------------------------
// Files utils
//-------------------------------------------------------------------------------------------------------------------------------------------
public static boolean deleteRecursive(File path) throws FileNotFoundException{
if (!path.exists()) throw new FileNotFoundException(path.getAbsolutePath());
boolean ret = true;
if (path.isDirectory()){
for (final File f : path.listFiles()){
ret = ret && Utils.deleteRecursive(f);
}
}
return ret && path.delete();
}
public static void deleteRecursiveFiles(File f) throws FileNotFoundException{
if (!f.exists()) throw new FileNotFoundException(f.getAbsolutePath());
if (f.isDirectory()){
for (final File f0 : f.listFiles()){
if(f0.isDirectory()) {
deleteRecursiveFiles(f0);
if(f0.listFiles().length == 0) {
f0.delete();
}
}else if(f0.isFile()) {
f0.delete();
}
}
}
}
public static void deleteRecursiveJavaFiles(File f) throws FileNotFoundException{
if (!f.exists()) throw new FileNotFoundException(f.getAbsolutePath());
if (f.isDirectory()){
for (final File f0 : f.listFiles()){
if(f0.isDirectory()) {
deleteRecursiveJavaFiles(f0);
if(f0.listFiles().length == 0) {
f0.delete();
}
}else if(f0.isFile() && f0.getName().toLowerCase().endsWith(".java")) {
f0.delete();
}
}
}
}
public static void copyFiles(String src, String dest, boolean overwrite) {
try {
Files.walk(Paths.get(src)).forEach(a -> {
final String aPath = a.toString();
final Path b = Paths.get(dest, aPath.substring(src.length()));
final String fileName = a.getFileName().toString();
try {
if (!aPath.equals(src)) {
if(Files.isDirectory(a) || fileName.endsWith(".java") && fileName.substring(0, fileName.length()-5).chars().allMatch(c -> VALID_JAVA_CHAR.indexOf(c) != -1)) {
Files.copy(a, b, overwrite ? new CopyOption[]{StandardCopyOption.REPLACE_EXISTING} : new CopyOption[]{});
}
}
} catch (IOException e) {
e.printStackTrace();
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
//-------------------------------------------------------------------------------------------------------------------------------------------
// Files utils
//-------------------------------------------------------------------------------------------------------------------------------------------
public static boolean checkUrl(ActionStatus status, String urlPath){
URL url = null;
try {
url = new URI(urlPath).toURL();
}catch(MalformedURLException | URISyntaxException e) {
status.setPassed(false);
status.setCode(ActionStatus.MALFORMED_GOTO_URL);
status.setData(urlPath);
return false;
}
int responseCode;
try {
if(urlPath.startsWith("https")) {
HttpsURLConnection con = (HttpsURLConnection)url.openConnection();
con.setRequestMethod("HEAD");
responseCode = con.getResponseCode();
}else {
HttpURLConnection con = (HttpURLConnection)url.openConnection();
con.setRequestMethod("HEAD");
responseCode = con.getResponseCode();
}
}catch (IOException e) {
status.setPassed(false);
status.setCode(ActionStatus.UNKNOWN_HOST_GOTO_URL);
status.setData(e.getMessage());
return false;
}
if(responseCode == HttpURLConnection.HTTP_OK) {
return true;
}else {
status.setPassed(false);
status.setCode(ActionStatus.UNREACHABLE_GOTO_URL);
status.setData(urlPath);
return false;
}
}
public static String removeExtension(String s) {
String separator = System.getProperty("file.separator");
String filename;
int lastSeparatorIndex = s.lastIndexOf(separator);
if (lastSeparatorIndex == -1) {
filename = s;
} else {
filename = s.substring(lastSeparatorIndex + 1);
}
int extensionIndex = filename.lastIndexOf(".");
if (extensionIndex == -1)
return filename;
return filename.substring(0, extensionIndex);
}
public static String leftString(final String str, final int len) {
if (str == null) {
return null;
}
if (len < 0) {
return "";
}
if (str.length() <= len) {
return str;
}
return str.substring(0, len);
}
public static String getCauseStackTraceString(Throwable e, String indent) {
StringBuilder sb = new StringBuilder();
Throwable cause = e.getCause();
if (cause != null) {
sb.append(indent);
sb.append("Java exception caused by -> ");
sb.append(getCauseStackTraceString(cause, indent));
}else {
sb.append(e.toString());
sb.append("\n");
StackTraceElement[] stack = e.getStackTrace();
if (stack != null) {
for (StackTraceElement stackTraceElement : stack) {
sb.append(indent);
sb.append("\tat ");
sb.append(stackTraceElement.toString());
sb.append("\n");
}
}
Throwable[] suppressedExceptions = e.getSuppressed();
// Print suppressed exceptions indented one level deeper.
if (suppressedExceptions != null) {
for (Throwable throwable : suppressedExceptions) {
sb.append(indent);
sb.append("\tSuppressed: ");
sb.append(getCauseStackTraceString(throwable, indent + "\t"));
}
}
}
return sb.toString();
}
//-------------------------------------------------------------------------------------------------------------------------------------------
// Image utils
//-------------------------------------------------------------------------------------------------------------------------------------------
public static byte[] loadImage(URL url) {
ByteArrayOutputStream output = new ByteArrayOutputStream();
try (InputStream inputStream = url.openStream()) {
int n = 0;
byte [] buffer = new byte[ 1024 ];
while (-1 != (n = inputStream.read(buffer))) {
output.write(buffer, 0, n);
}
return output.toByteArray();
} catch (IOException e) {
}
return null;
}
public static byte[] getWhitePixel() {
return Base64.getDecoder().decode(WHITE_PIXEL);
}
//-------------------------------------------------------------------------------------------------------------------------------------------
// Groovy script
//-------------------------------------------------------------------------------------------------------------------------------------------
/*public static String[] runGroovyScript(String scriptName, String[] libsPath, String[] parameters) throws IOException, ResourceException, ScriptException {
final Binding binding = new Binding();
binding.setVariable("args", parameters);
final GroovyScriptEngine engine = new GroovyScriptEngine(libsPath);
final Object result = engine.run(scriptName, binding);
if(result == null) {
return new String[0];
}else if(result instanceof String[]) {
return (String[])result;
}else{
return new String[] {result.toString()};
}
}*/
//-------------------------------------------------------------------------------------------------------------------------------------------
// JSON CSV utils
//-------------------------------------------------------------------------------------------------------------------------------------------
public static ParameterDataFile loadData(String url) throws MalformedURLException, URISyntaxException{
return loadData(new ActionStatus(), new URI(url).toURL());
}
public static ParameterDataFile loadData(ActionStatus status,String url) throws MalformedURLException, URISyntaxException{
return loadData(status, new URI(url).toURL());
}
public static ParameterDataFile loadData(ActionStatus status, URL url){
return new ParameterDataFile(status, url);
}
//-------------------------------------------------------------------------------------------------------------------------------------------
// ATS artifacts utils
//-------------------------------------------------------------------------------------------------------------------------------------------
private final static Pattern SYS_VERSION_PATTERN = Pattern.compile("");
public static String getArtifactLastVersion(String folderUrl) {
String version = null;
try {
final HttpURLConnection con = (HttpURLConnection) new URI(folderUrl).toURL().openConnection();
if(con.getResponseCode() == 200) {
final InputStream inputStream = con.getInputStream();
final BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
final StringBuilder builder = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
builder.append(line);
}
inputStream.close();
final ArrayList versions = new ArrayList<>();
final Matcher matcher = SYS_VERSION_PATTERN.matcher(builder.toString());
int index = 0;
while (matcher.find(index)) {
versions.add(matcher.group(1));
index = matcher.end();
}
if(versions.size() > 0) {
final Version v = versions.stream()
.map(Version::parse)
.sorted(Collections.reverseOrder())
.findFirst().get();
if(v != null) {
version = v.toString().replace(".zip", "").replace(".tgz", "");
}
}
}
}catch(IOException | URISyntaxException e) {}
return version;
}
public static String escapeMathSymbolsOnce(String data) {
return data.replace("<", "").replace(">", "").replace("=","").replace("~","");
}
public static long getSecondsForTimeline(long millis) {
long milliseconds = millis % 60000;
long seconds = milliseconds / 1000;
long remainder = milliseconds % 1000;
if (remainder >= 500) {
seconds++;
}
return seconds;
}
public static long getMinutesForTimeLine(long millis) {
long minutes = millis / 60000;
if (minutes >= 60) {
return minutes - 60;
} else {
return minutes;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy