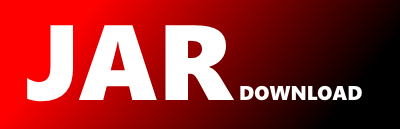
com.ats.tools.logger.ExecutionLogger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools.logger;
import java.io.PrintStream;
import org.testng.Reporter;
import com.ats.script.actions.Action;
import com.ats.tools.logger.levels.AtsLogger;
import com.ats.tools.logger.levels.ErrorLevelLogger;
import com.ats.tools.logger.levels.FullLevelLogger;
import com.ats.tools.logger.levels.InfoLevelLogger;
import com.ats.tools.logger.levels.WarningLevelLogger;
public class ExecutionLogger {
public final static String SILENT = "silent";
private final static String INFO_LEVEL = "info";
private final static String ERROR_LEVEL = "error";
private final static String ALL_LEVEL = "all";
private final static String WARNING_LEVEL = "warning";
public static final String ANSI_RESET = "\u001B[0m";
public static final String ANSI_RED = "\u001B[31m";
public static final String ANSI_GREEN = "\u001B[32m";
public static final String ANSI_YELLOW = "\u001B[33m";
public static final String ANSI_BLUE = "\u001B[34m";
private AtsLogger levelLogger;
public ExecutionLogger() {
levelLogger = new AtsLogger();
}
public ExecutionLogger(PrintStream sysout) {
this(sysout, INFO_LEVEL, AtsLogger.TYPE_TERM);
}
public ExecutionLogger(PrintStream sysout, String verbose, String type) {
if(ERROR_LEVEL.equalsIgnoreCase(verbose)) {
levelLogger = new ErrorLevelLogger(sysout, type, "Error");
}else if(INFO_LEVEL.equalsIgnoreCase(verbose)) {
levelLogger = new InfoLevelLogger(sysout, type, "Error + Info");
}else if(WARNING_LEVEL.equalsIgnoreCase(verbose)) {
levelLogger = new WarningLevelLogger(sysout, type, "Error + Info + Warning");
}else if(ALL_LEVEL.equalsIgnoreCase(verbose)) {
levelLogger = new FullLevelLogger(sysout, type, "Error + Info + Warning + Details");
}else {
levelLogger = new AtsLogger(sysout, type, "Disabled");
}
}
public void sendLog(int code, String message, Object value) {
if(code < 100 ) {
sendInfo(message, value.toString());
}else if (code < 399){
sendWarning(message, value.toString());
}else {
sendError(message, value.toString());
}
}
public void sendScriptInfo(String message) {
levelLogger.printScript(message);
Reporter.log("- " + message);
}
public void sendScriptFail(String actionName, String testName, int line, String app, String message) {
levelLogger.printFail(actionName, testName, line, app, message);
Reporter.log("- " + message);
}
public void sendScriptError(String info, String value) {
levelLogger.printError(info, value);
}
public void sendAction(Action action, String testName, int line) {
levelLogger.action(action, testName, line);
}
public void sendComment(String message) {
levelLogger.comment(message);
Reporter.log("- " + message);
}
public void sendWarning(int code, String message, Object value) {
levelLogger.warning(message + " -> " + value);
}
public void sendWarning(String message, String value) {
levelLogger.warning(message + " -> " + value);
}
public void sendInfo(String message, String value) {
levelLogger.info(message + " -> " + value);
}
public void sendError(String message, String value) {
levelLogger.error(message, value);
}
public void sendDriverLog(String value) {
levelLogger.driverLog(value);
}
public void sendDriverWarning(String value) {
levelLogger.driverWarning(value);
}
public void sendDriverError(String value) {
levelLogger.driverError(value);
}
public void sendDriverOutput(String value) {
levelLogger.driverOutput(value);
}
public void sendScriptSuite(String value) {
levelLogger.suiteOutput(value);
}
public void sendScriptTest(String value) {
levelLogger.testOutput(value);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy