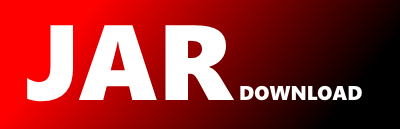
com.ats.tools.logger.levels.AtsLogger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools.logger.levels;
import java.io.PrintStream;
import com.ats.script.actions.Action;
import com.ats.tools.logger.NullPrintStream;
import com.ats.tools.logger.prefix.AzureDevOps;
import com.ats.tools.logger.prefix.ILogType;
import com.ats.tools.logger.prefix.Terminal;
import com.ats.tools.logger.prefix.TerminalColor;
public class AtsLogger {
public final static String TYPE_TERM = "term";
public final static String TYPE_AZD = "azd";
protected ILogType logType = new TerminalColor();
protected PrintStream out;
private static volatile AtsLogger instance;
public static AtsLogger getInstance() {
synchronized(AtsLogger.class) {
if (instance == null) {
instance = new AtsLogger();
}
return instance;
}
}
public static void printLog(StringBuilder value) {
printLog(value.toString());
}
public static void printLog(String value) {
getInstance().printScript(value);
}
public AtsLogger() {
this.out = new NullPrintStream();
}
public AtsLogger(PrintStream out, String type, String level) {
this.out = out;
String logsTypeName = "";
if(TYPE_TERM.equals(type)) {
logType = new TerminalColor();
logsTypeName = "[Terminal Color] ";
}else if(TYPE_AZD.equals(type)) {
logType = new AzureDevOps();
logsTypeName = "[Azure DevOps] ";
}else {
logType = new Terminal();
}
instance = this;
out.println(logType.getLoggerData() + logsTypeName + "logger level -> " + level);
}
public void suiteOutput(String value) {
out.println(logType.getSuiteData() + value);
}
public void testOutput(String value) {
out.println(logType.getTestData(value) + value);
}
public void printFail(String actionName, String testName, int line, String info, String details) {
out.println(logType.getFailData(actionName, testName, line, info, details));
}
public void printError(String info, String value) {
out.println(logType.getErrorData() + info + " -> " + value);
}
public void printInfo(String value) {
out.println(logType.getInfoData() + value);
}
public void printWarning(String value) {
out.println(logType.getWarningData() + value);
}
public void printScript(String value) {
out.println(logType.getScriptData(value) + value);
}
public void printAction(String value) {
out.println(logType.getActionData() + value);
}
public void comment(String message) {
out.println(logType.getCommentData(message));
}
public void driverLog(String value) {
out.println(logType.getDriverLog() + value);
}
public void driverWarning(String value) {
out.println(logType.getDriverWarning() + value);
}
public void driverError(String value) {
out.println(logType.getDriverError() + value);
}
public void driverOutput(String value) {}
public void warning(String message) {}
public void info(String message) {}
public void error(String info, String value) {}
public void action(Action action, String testName, int line) {}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy