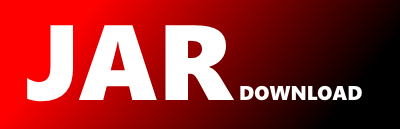
com.ats.tools.report.CampaignReportConcatenatorGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools.report;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FilenameFilter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.OutputStreamWriter;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.util.Arrays;
import java.util.Base64;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import javax.xml.transform.stream.StreamSource;
import org.w3c.dom.DOMException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import com.ats.executor.ActionTestScript;
import com.ats.generator.ATS;
import com.ats.tools.ResourceContent;
import com.ats.tools.Utils;
import com.ats.tools.logger.levels.AtsLogger;
import com.google.gson.Gson;
import com.google.gson.stream.JsonReader;
import net.sf.jasperreports.engine.JRException;
import net.sf.jasperreports.engine.JasperCompileManager;
import net.sf.jasperreports.engine.JasperExportManager;
import net.sf.jasperreports.engine.JasperFillManager;
public class CampaignReportConcatenatorGenerator {
public static final String ATS_REPORT = "ats-report";
public static final String MGT_REPORT = "mgt-report";
public static final String VALID_REPORT = "validation-report";
public static final String TEMPLATE_EXTENSION = "_html.xml";
private static final String REPORT_FOLDER = "reports";
private static final String TEMPLATE_FOLDER = "templates";
private static final String IMAGE_FOLDER = "images";
private static final String CUSTOM_TEMPLATES_FOLDER = "/" + REPORT_FOLDER + "/" + TEMPLATE_FOLDER + "/";
private static final String CUSTOM_IMAGES_FOLDER = "/" + REPORT_FOLDER + "/" + IMAGE_FOLDER + "/";
private final static String XML_SOURCE_NAME = ATS_REPORT + ".xml";
private final static String XML_SOURCE_ROOT = "ats-report";
private static String outputFolder = null;
private static int atsReportLvl = 0;
private static int managementReport = 0;
private static int validationReport = 0;
private static LinkedList inputFolders = null;
private static DocumentBuilder builderTest;
static {
try {
builderTest = DocumentBuilderFactory.newInstance().newDocumentBuilder();
} catch (ParserConfigurationException e) {
throw new RuntimeException(e);
}
}
private static Document outputXmlDocument = null;
private static Element concatRacineElement = null;
private static final List trueList = Arrays.asList("on", "true", "1", "yes", "y");
public static void main(String[] args) throws ParserConfigurationException, IOException, TransformerException, SAXException {
String[] SInputFolders = null;
for (int i = 0; i < args.length; i++) {
String string = args[i];
if (string.startsWith("--") && i + 1 < args.length) {
switch (string.substring(2).toLowerCase()) {
case "outputfolder":
case "output":
case "reportfolder":
outputFolder = args[i + 1].replaceAll("\"", "");
break;
case "details":
case "ats-report":
atsReportLvl = Utils.string2Int(args[i + 1], 0);
break;
case "validation-report":
validationReport = trueList.indexOf(args[i + 1]) > -1 ? 1 : 0;
break;
case "concat-report-folders":
SInputFolders = args[i + 1].split(";");
break;
default:
System.out.println("unavailable parameter : " + string.substring(2).toLowerCase());
break;
}
}
}
if (outputFolder == null) {
System.out.println("Error, output folder not defined !");
return;
}
final Path outputFolderPath = Paths.get(outputFolder).toAbsolutePath();
if (!outputFolderPath.toFile().exists()) {
System.out.println("Error, output folder path not found : " + outputFolder);
return;
}
if (SInputFolders != null) {
reportConcatenation(SInputFolders);
} else {
final File jsonSuiteFilesFile = outputFolderPath.resolve(CampaignReportGenerator.ATS_JSON_SUITES).toFile();
if (jsonSuiteFilesFile.exists()) {
try {
new CampaignReportGenerator(outputFolderPath, jsonSuiteFilesFile, atsReportLvl);
} catch (IOException | TransformerException | ParserConfigurationException | SAXException e) {
e.printStackTrace();
}
} else {
System.out.println("Suites file not found (" + CampaignReportGenerator.ATS_JSON_SUITES + ") at : " + outputFolderPath);
}
}
}
public static void initFolders(Path projectPath) {
try {
projectPath.resolve(REPORT_FOLDER).toFile().mkdirs();
projectPath.resolve(REPORT_FOLDER).resolve(CUSTOM_TEMPLATES_FOLDER).toFile().mkdirs();
projectPath.resolve(REPORT_FOLDER).resolve(CUSTOM_IMAGES_FOLDER).toFile().mkdirs();
} catch (Exception e) {
}
}
private static void copyReportsTemplate(Path fromPath, Path toPath) {
if (Files.exists(fromPath)) {
for (File f : fromPath.toFile().listFiles()) {
if (f.isFile()) {
try {
Files.copy(f.toPath(), toPath.resolve(f.getName()), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
}
}
}
}
}
private static void reportConcatenation(String[] SInputFolders) throws TransformerException, IOException, SAXException {
if (SInputFolders.length > 0) {
File f = null;
inputFolders = new LinkedList<>();
for (String sPath : SInputFolders) {
f = new File(sPath);
if (f.exists()) {
if (f.isDirectory()) {
inputFolders.add(Path.of(sPath));
} else {
System.out.println("Warning, inputFolder (" + sPath + ") is not a Folder, it will not used");
}
} else {
System.out.println("Warning, inputFolder (" + sPath + ") doesn't exist");
}
}
Path[] aInputFolders = inputFolders.toArray(new Path[inputFolders.size()]);
if (aInputFolders.length > 0) {
outputXmlDocument = builderTest.newDocument();
concatRacineElement = outputXmlDocument.createElement("ats-report-concatenation");
for (Path inputFolder : inputFolders) {
if (inputFolder.toFile().exists()) {
final File jsonSuiteFilesFile = inputFolder.resolve(CampaignReportGenerator.ATS_JSON_SUITES).toFile();
if (jsonSuiteFilesFile.exists()) {
try {
concatRacineElement.appendChild(ReportDataRecover(inputFolder, jsonSuiteFilesFile));
} catch (IOException | TransformerException | ParserConfigurationException |
SAXException e) {
e.printStackTrace();
}
} else {
System.out.println("Suites file not found (" + CampaignReportGenerator.ATS_JSON_SUITES + ") at : " + inputFolder);
}
}
}
if (concatRacineElement != null) {
outputXmlDocument.appendChild(concatRacineElement);
}
Path outputFolderPath = Path.of(outputFolder);
final Path atsXmlDataPath = outputFolderPath.resolve(XML_SOURCE_NAME);
writeXMLDatafile(atsXmlDataPath, null);
LinkedList reports;
reports = reportParametersSelection(Integer.toString(atsReportLvl), null, Integer.toString(validationReport), null);
htmlGeneration(outputFolderPath, atsXmlDataPath, reports);
} else {
System.out.println("No available folder found");
}
}
}
private static void htmlGeneration(Path outputFolderPath, Path atsXmlDataPath, LinkedList reports) throws IOException, TransformerException, SAXException {
if (outputFolderPath.toFile().isDirectory()) {
if (atsXmlDataPath.toFile().exists()) {
for (String reportFile : reports) {
final File htmlTemplateFile = copyResource(reportFile, outputFolderPath);
MinifyWriter filteredWriter = null;
Transformer htmlTransformer = null;
Path inputTemplate = null;
if ("suites_html.xml".equals(reportFile)) {
inputTemplate = outputFolderPath.resolve(CampaignReportGenerator.ATS_REPORT);
} else {
inputTemplate = outputFolderPath.resolve(reportFile.substring(0, reportFile.indexOf("_")) + ".html");
}
filteredWriter = new MinifyWriter(
Files.newBufferedWriter(inputTemplate, StandardCharsets.UTF_8));
htmlTransformer = TransformerFactory.newInstance().newTransformer(new StreamSource(htmlTemplateFile));
htmlTransformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
htmlTransformer.transform(
new DOMSource(builderTest.parse(new InputSource(
new InputStreamReader(Files.newInputStream(atsXmlDataPath), StandardCharsets.UTF_8)))),
new StreamResult(filteredWriter));
filteredWriter.close();
}
} else {
System.out.println("[ERROR] generation aborted : inputXmlDocument not defined!");
}
} else {
if (outputFolderPath.toFile().exists())
System.out.println("[ERROR] generation aborted : inputXmlDocument not defined!");
}
}
private static void writeXMLDatafile(Path XmlDataPath, Document DocumentToWrite) throws TransformerException, FileNotFoundException {
Document DOMDocument = DocumentToWrite != null ? DocumentToWrite : outputXmlDocument;
if (DOMDocument != null) {
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.transform(new DOMSource(DOMDocument),
new StreamResult(new OutputStreamWriter(
new FileOutputStream(XmlDataPath.toFile()),
StandardCharsets.UTF_8)));
} else {
System.out.println("[ERROR] generation aborted : OutputDOMxmlDocument not defined!");
}
}
private static Node ReportDataRecover(Path outputFolderPath, File jsonSuiteFilesFile) throws IOException, SAXException, ParserConfigurationException, TransformerException {
if (outputFolderPath.resolve(XML_SOURCE_NAME).toFile().exists()) {
final File XMLReportData = outputFolderPath.resolve(XML_SOURCE_NAME).toFile();
final Element reportData = builderTest.parse(XMLReportData).getDocumentElement();
reportData.setAttribute("path", outputFolderPath.toString());
reportData.setAttribute("devReportLevel", Integer.toString(atsReportLvl));
reportData.setAttribute("mgtReportLevel", Integer.toString(managementReport));
reportData.setAttribute("validReportLevel", Integer.toString(validationReport));
return outputXmlDocument.importNode(reportData, true);
} else {
return reportGenerator(outputFolderPath, jsonSuiteFilesFile);
}
}
public static Node reportGenerator(Path outputFolderPath, File jsonSuiteFilesFile)
throws IOException, TransformerException, ParserConfigurationException, SAXException, DOMException {
LinkedList reports = new LinkedList<>();
final Path reportPath = Paths.get(
com.ats.script.Project.SRC_FOLDER,
com.ats.script.Project.ASSETS_FOLDER,
com.ats.script.Project.RESOURCES_FOLDER,
REPORT_FOLDER);
if (Files.exists(reportPath)) {
copyReportsTemplate(reportPath.resolve(TEMPLATE_FOLDER), outputFolderPath);
copyReportsTemplate(reportPath.resolve(IMAGE_FOLDER), outputFolderPath);
}
SuitesReport suiteReport = null;
try {
final JsonReader reader = new JsonReader(new FileReader(jsonSuiteFilesFile));
suiteReport = new Gson().fromJson(reader, SuitesReport.class);
reader.close();
} catch (IOException e) {
}
if (suiteReport == null) {
System.out.println("No suites found, nothing to do !");
return null;
}
//create DOM Document
Document writeSuiteXMLElement = builderTest.newDocument();
final Element report = writeSuiteXMLElement.createElement(XML_SOURCE_ROOT);
report.setAttribute("atsVersion", ATS.getAtsVersion());
report.setAttribute("projectId", suiteReport.projectId);
report.setAttribute("projectUuid", suiteReport.projectUuid);
report.setAttribute("projectDescription", suiteReport.projectDescription);
report.setAttribute("path", outputFolderPath.toString());
writeSuiteXMLElement.appendChild(report);
insertBasicPicture(outputFolderPath, writeSuiteXMLElement);
report.appendChild(insertBasicPicture(outputFolderPath, writeSuiteXMLElement));
report.setAttribute("suitesCount", String.valueOf(suiteReport.suites.length));
int totalTests = 0;
int totalTestsPassed = 0;
int totalSuitesPassed = 0;
int totalActions = 0;
int totalDuration = 0;
for (SuitesReportItem info : suiteReport.suites) {
final Path suitePath = outputFolderPath.resolve(info.getName());
boolean suitePassed = true;
final Element suite = writeSuiteXMLElement.createElement("suite");
suite.setAttribute("name", info.getName());
suite.setAttribute("description", info.getDescription());
suite.setAttribute("dateOrder", info.getDateOrder());
final Element parameters = writeSuiteXMLElement.createElement("parameters");
suite.appendChild(parameters);
final Properties properties = new Properties();
final Path suiteParamPath = suitePath.resolve(ActionTestScript.SUITE_PARAMETERS);
reports = reportParametersSelection(info.devReportLvl, info.mgtReportLvl, info.validReportLvl, report);
if (Files.exists(suiteParamPath)) {
properties.load(Files.newBufferedReader(suiteParamPath));
}
for (Map.Entry entry : info.getParametersEntries()) {
final Element parameter = writeSuiteXMLElement.createElement("parameter");
parameter.setAttribute("name", entry.getKey());
parameter.setAttribute("value", properties.getProperty(entry.getKey(), entry.getValue()));
parameter.setAttribute("defaultValue", entry.getValue());
parameters.appendChild(parameter);
}
final Element tests = writeSuiteXMLElement.createElement("tests");
suite.appendChild(tests);
int testsPassed = 0;
int testsFailed = 0;
int actionsExecuted = 0;
int suiteDuration = 0;
suite.setAttribute("testsCount", String.valueOf(info.getTestsCount()));
for (String scriptName : info.tests) {
final File xmlDataFile = suitePath.resolve(scriptName + "_xml").resolve(XmlReport.REPORT_DATA_FILE).toFile();
if (xmlDataFile.exists()) {
totalTests++;
int testDuration = 0;
final Element atsTest = builderTest.parse(xmlDataFile).getDocumentElement();
final NodeList actionsList = atsTest.getElementsByTagName("action");
final NodeList summary = atsTest.getElementsByTagName("summary");
if (summary.getLength() > 0) {
if ("1".equals(summary.item(0).getAttributes().getNamedItem("status").getNodeValue())) {
testsPassed++;
totalTestsPassed++;
} else {
testsFailed++;
suitePassed = false;
}
actionsExecuted += Utils.string2Int(summary.item(0).getAttributes().getNamedItem("actions").getNodeValue(), actionsList.getLength());
}else {
actionsExecuted += actionsList.getLength();
}
LinkedList actionImagesList = new LinkedList<>();
for (int i = 0; i < actionsList.getLength(); i++) {
final Node action = actionsList.item(i);
Element eElement = (Element) action;
Node element = null;
if ((element = eElement.getElementsByTagName("duration").item(0)) != null) {
testDuration += Utils.string2Int(element.getTextContent());
}
element = null;
if ((element = eElement.getElementsByTagName("img").item(0)) != null) {
actionImagesList.add(element.getAttributes().getNamedItem("src").getNodeValue());
}
}
extractReportPicture(actionImagesList, suitePath, scriptName);
atsTest.setAttribute("duration", String.valueOf(testDuration));
suiteDuration += testDuration;
tests.appendChild(writeSuiteXMLElement.importNode(atsTest, true));
}
}
totalActions += actionsExecuted;
totalDuration += suiteDuration;
if (suitePassed) {
totalSuitesPassed++;
}
suite.setAttribute("passed", String.valueOf(suitePassed));
suite.setAttribute("duration", String.valueOf(suiteDuration));
suite.setAttribute("actions", String.valueOf(actionsExecuted));
suite.setAttribute("testsPassed", String.valueOf(testsPassed));
suite.setAttribute("testsFailed", String.valueOf(testsFailed));
report.appendChild(suite);
}
report.setAttribute("duration", String.valueOf(totalDuration));
report.setAttribute("tests", String.valueOf(totalTests));
report.setAttribute("testsPassed", String.valueOf(totalTestsPassed));
report.setAttribute("suitesPassed", String.valueOf(totalSuitesPassed));
report.setAttribute("actions", String.valueOf(totalActions));
final Path atsXmlDataPath = outputFolderPath.resolve(XML_SOURCE_NAME);
writeXMLDatafile(atsXmlDataPath, writeSuiteXMLElement);
htmlGeneration(outputFolderPath, atsXmlDataPath, reports);
jasperGeneration(outputFolderPath);
return outputXmlDocument.importNode(report, true);
}
private static Node insertBasicPicture(Path outputFolderPath, Document writeSuiteXMLElement) throws IOException {
final Element picsList = writeSuiteXMLElement.createElement("pics");
final String[] defaultImages = new String[]{"logo.png", "true.png", "false.png", "warning.png", "noStop.png", "api.png", "pdf.png",
"external_link_10_white","external_link_12_blue","external_link_12_blue_visited","external_link_12_grey","external_link_16_grey","external_link_16_white", "run_16"};
for (String img : defaultImages) {
final Element pic = writeSuiteXMLElement.createElement("pic");
pic.setAttribute("name", img.replace(".png", ""));
byte[] imgBytes = null;
if (Files.exists(outputFolderPath.resolve(img))) {
imgBytes = Files.readAllBytes(outputFolderPath.resolve(img));
} else {
imgBytes = ResourceContent.class.getResourceAsStream(CUSTOM_IMAGES_FOLDER + img).readAllBytes();
}
pic.setTextContent("data:image/png;base64," + getBase64DefaultImages(imgBytes));
picsList.appendChild(pic);
}
return picsList;
}
private static void extractReportPicture(LinkedList actionImagesList, Path suitePath, String scriptName) throws ParserConfigurationException, TransformerException, IOException {
//for (String img : actionImagesList) {
//DocumentBuilder builderScreen = DocumentBuilderFactory.newInstance().newDocumentBuilder();
//Document writeXmlScreen = builderScreen.newDocument();
//Element screenshot = writeXmlScreen.createElement("Screenshot");
//writeXmlScreen.appendChild(screenshot);
//final Element pic = writeXmlScreen.createElement("pic");
//CampaignReportGenerator.saveB64Pic(suitePath.resolve(scriptName + "_xml").resolve(img));
/*Path imagePath = suitePath.resolve(scriptName + "_xml").resolve(img);
byte[] encoded = null;
if (Files.exists(imagePath)) {
try {
final File f = new File(imagePath.toString());
final FileInputStream fileInputStreamReader = new FileInputStream(f);
final byte[] fileContent = new byte[(int) f.length()];
fileInputStreamReader.read(fileContent);
encoded = Base64.getEncoder().encode(fileContent);
fileInputStreamReader.close();
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("image loading error");
continue;
}
//final String newPathImg = imagePath.toString().substring(0, imagePath.toString().lastIndexOf(".")) + ".b64";
File outputFile = new File(imagePath.toString().substring(0, imagePath.toString().lastIndexOf(".")) + ".b64");
try (FileOutputStream outputStream = new FileOutputStream(outputFile)) {
outputStream.write("data:image/png;base64,".getBytes());
outputStream.write(encoded);
outputStream.write(" ".getBytes());
}*/
/*byte[] buffer = ("data:image/png;base64," + encodedString + " ").getBytes();
FileChannel rwChannel = new RandomAccessFile(newPathImg, "w").getChannel();
ByteBuffer wrBuf = rwChannel.map(FileChannel.MapMode.READ_WRITE, 0, buffer.length);
wrBuf.put(buffer);
rwChannel.close();*/
/*pic.setTextContent("data:image/png;base64," + encodedString);
screenshot.appendChild(pic);
final TransformerFactory tf = TransformerFactory.newInstance("com.sun.org.apache.xalan.internal.xsltc.trax.TransformerFactoryImpl", null);
final Transformer transformerScreen = tf.newTransformer();
transformerScreen.transform(new DOMSource(writeXmlScreen),
new StreamResult(new OutputStreamWriter(new FileOutputStream(newPathImg),
StandardCharsets.UTF_8)));*/
//}
}
private static LinkedList reportParametersSelection(String ATSReportFileSuite, String MGTReportFileSuite, String ValidReportFileSuite, Element report) {
final String SATSReport = System.getProperty(CampaignReportGenerator.ATS_REPORT);
final String SMGTReport = System.getProperty(CampaignReportGenerator.MGT_REPORT);
final String SVALIDReport = System.getProperty(CampaignReportGenerator.VALID_REPORT);
LinkedList reports = new LinkedList<>();
//Report level definition in data file
int devReport = 0;
int mgtReport = 0;
int validReport = 0;
if (atsReportLvl == 0) {
devReport = Utils.string2Int(SATSReport, Utils.string2Int(ATSReportFileSuite, atsReportLvl));
} else {
devReport = atsReportLvl;
}
if (SMGTReport != null && trueList.contains(SMGTReport.toLowerCase())) {
mgtReport = 1;
} else {
mgtReport = Utils.string2Int(MGTReportFileSuite, managementReport);
}
if (SVALIDReport != null && trueList.contains(SVALIDReport.toLowerCase())) {
validReport = 1;
} else {
validReport = Utils.string2Int(ValidReportFileSuite, validationReport);
}
String reportName = "suites" + TEMPLATE_EXTENSION;
if (devReport != 0 && (!reports.contains(reportName))) {
reports.add(reportName);
}
reportName = MGT_REPORT + TEMPLATE_EXTENSION;
if (mgtReport != 0 && (!reports.contains(reportName))) {
reports.add(reportName);
}
reportName = VALID_REPORT + TEMPLATE_EXTENSION;
if (validReport != 0 && (!reports.contains(reportName))) {
reports.add(reportName);
}
if (report != null) {
report.setAttribute("devReportLevel", Integer.toString(devReport));
report.setAttribute("mgtReportLevel", Integer.toString(mgtReport));
report.setAttribute("validReportLevel", Integer.toString(validReport));
}
return reports;
}
public static void jasperGeneration(Path outputFolderPath) throws ParserConfigurationException, IOException, TransformerException {
copyResource("summary.jrxml", outputFolderPath);
copyResource("suite.jrxml", outputFolderPath);
copyResource("test.jrxml", outputFolderPath);
final String outputPath = outputFolderPath.toAbsolutePath().toString();
final String reportName = "summary";
//-----------------------------------------------------------------------------------------------------
// Clean files
//-----------------------------------------------------------------------------------------------------
File[] filesToDelete = outputFolderPath.toFile().listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
return name.endsWith(".jrprint") || name.endsWith(".jasper") || name.equals(reportName + ".pdf");
}
});
for (File f : filesToDelete) {
try {
f.delete();
System.out.println("File : " + f.getAbsolutePath());
} catch (Exception e) {
}
}
try {
generatePdf(reportName, outputPath);
} catch (JRException e) {
e.printStackTrace();
}
}
private static void generatePdf(String reportName, String outputPath) throws JRException {
outputPath += File.separator;
final String reportFullName = outputPath + reportName + ".pdf";
final Map parameters = new HashMap<>();
parameters.put("workingDir", outputPath);
parameters.put("xmlSource", XML_SOURCE_NAME);
parameters.put("xmlSourceRoot", XML_SOURCE_ROOT);
AtsLogger.printLog("generate reports templates ...");
JasperCompileManager.compileReportToFile(outputPath + "test.jrxml", outputPath + "test.jasper");
JasperCompileManager.compileReportToFile(outputPath + "suite.jrxml", outputPath + "suite.jasper");
JasperCompileManager.compileReportToFile(outputPath + "summary.jrxml", outputPath + "summary.jasper");
String fileName = JasperFillManager.fillReportToFile(outputPath + reportName + ".jasper", parameters);
JasperExportManager.exportReportToPdfFile(fileName, reportFullName);
AtsLogger.printLog("PDF file -> " + reportFullName + " ... OK");
}
private static File copyResource(String resName, Path dest) throws IOException {
Path filePath = dest.resolve(resName);
if (!Files.exists(filePath)) {
InputStream is = ResourceContent.class.getResourceAsStream(CUSTOM_TEMPLATES_FOLDER + resName);
InputStream in = new BufferedInputStream(is);
File targetFile = dest.resolve(resName).toFile();
OutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile));
byte[] buffer = new byte[1024];
int lengthRead;
while ((lengthRead = in.read(buffer)) > 0) {
out.write(buffer, 0, lengthRead);
out.flush();
}
in.close();
is.close();
out.close();
}
return filePath.toFile();
}
private static String getBase64DefaultImages(byte[] b) throws IOException {
return Base64.getEncoder().encodeToString(b);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy