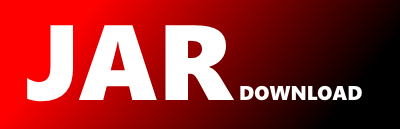
com.ats.tools.report.CampaignReportGenerator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
/*
Licensed to the Apache Software Foundation (ASF) under one
or more contributor license agreements. See the NOTICE file
distributed with this work for additional information
regarding copyright ownership. The ASF licenses this file
to you under the Apache License, Version 2.0 (the
"License"); you may not use this file except in compliance
with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
*/
package com.ats.tools.report;
import com.ats.executor.ActionTestScript;
import com.ats.generator.ATS;
import com.ats.tools.ResourceContent;
import com.ats.tools.Utils;
import com.ats.tools.logger.levels.AtsLogger;
import com.ats.tools.report.analytics.TestsResultBarChart;
import com.ats.tools.report.utils.AdvancedAtsLogger;
import com.ats.tools.report.utils.FileUtils;
import com.ats.tools.report.utils.ImageProcessingUtils;
import com.ats.tools.report.utils.MemoryMonitor;
import com.google.gson.Gson;
import com.google.gson.stream.JsonReader;
import net.sf.jasperreports.engine.*;
import net.sf.jasperreports.engine.export.HtmlExporter;
import net.sf.jasperreports.engine.fill.JRSwapFileVirtualizer;
import net.sf.jasperreports.engine.util.JRSwapFile;
import net.sf.jasperreports.export.SimpleExporterInput;
import net.sf.jasperreports.export.SimpleHtmlExporterConfiguration;
import net.sf.jasperreports.export.SimpleHtmlExporterOutput;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.*;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import javax.xml.transform.stream.StreamSource;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.util.*;
public class CampaignReportGenerator {
private static final String HTML_HEADER = "ats-report "
+ ""
+ "\r\n"
+ " "
+ ResourceContent.getJasperReportHtmlContent();
public static final String AGILITEST_RESOURCES_PREFIX = "AgilitestResources";
public static final String ATS_JSON_SUITES = "ats-suites.json";
public static final String ATS_REPORT = "ats-report";
public static final String ATS_TEST_REPORT = "ats-test-report";
public static final String ATS_REPORT_ENV = "ATS_REPORT";
public static final String MGT_REPORT = "mgt-report";
public static final String VALID_REPORT = "validation-report";
public static final String TEMPLATE_EXTENSION = "_html.xml";
private static final String REPORT_FOLDER = "reports";
private static final String TEMPLATE_FOLDER = "templates";
private static final String IMAGE_FOLDER = "images";
private static final String CUSTOM_TEMPLATES_FOLDER = "/" + REPORT_FOLDER + "/" + TEMPLATE_FOLDER + "/";
private static final String CUSTOM_IMAGES_FOLDER = "/" + REPORT_FOLDER + "/" + IMAGE_FOLDER + "/";
private final static String XML_SOURCE_ROOT = "ats-report";
private final static String XML_SOURCE_NAME = ATS_REPORT + ".xml";
public final static String ATS_REPORT_HTML = ATS_REPORT + ".html";
public final static String ATS_TEST_REPORT_HTML = ATS_TEST_REPORT + ".html";
private static int details = 0;
public static int DETAILS_AND_SCREEN = 3;
private static final List trueList = Arrays.asList(new String[]{"on", "true", "1", "yes", "y"});
private long reportGenerationStartTime;
public static void main(String[] args) {
String output = null;
List listArgs = Arrays.asList(args);
if (listArgs.contains("--help") || listArgs.contains("-h") || listArgs.contains("--h") || listArgs.contains("/?") || listArgs.contains("\\?")) {
usage();
return;
}
for (int i = 0; i < args.length; i++) {
String string = args[i];
if (string.startsWith("--") && i + 1 < args.length) {
switch (string.substring(2)) {
case "outputFolder":
case "output":
case "reportFolder":
output = args[i + 1].replaceAll("\"", "");
break;
case "details":
case "atsReport":
details = Utils.string2Int(args[i + 1], 0);
break;
}
}
}
if (output == null) {
System.out.println("Error, output folder not defined !");
return;
}
final Path outputFolderPath = Paths.get(output).toAbsolutePath();
if (!outputFolderPath.toFile().exists()) {
System.out.println("Error, output folder path not found : " + output);
return;
}
final File jsonSuiteFilesFile = outputFolderPath.resolve(ATS_JSON_SUITES).toFile();
if (jsonSuiteFilesFile.exists()) {
try {
new CampaignReportGenerator(outputFolderPath, jsonSuiteFilesFile, details);
} catch (IOException | TransformerException | ParserConfigurationException | SAXException e) {
e.printStackTrace();
}
} else {
System.out.println("Suites file not found : " + ATS_JSON_SUITES);
}
}
private static void usage() {
System.out.print("Usage : Experiment dev" + "\n" +
"This class is use to extract data from ATSV, convert and generate to other documents (HTML, PDF)" + "\n\n" +
"Parameters usage :" + "\n" +
"\t--output" + "\t\t\t" + "(mandatory)(absolute or relative path) : parameter used to" + "\n" +
"\t outputFolder" + "\t\t" + "specify the input and output folder of generated folder" + "\n" +
"\t reportFolder" + "\t\t" + "" + "\n" +
"\t--jasper" + "\t\t\t" + "(optional)(absolute or relative path) : parameter used to specify" + "\n" +
"\t\t\t\t\t\t" + "the folder of jasper software. If this one isn't specified" + "\n" +
"\t\t\t\t\t\t" + "the PDF report isn't generated" + "\n" +
"\t--details" + "\t\t\t" + "(optional) : specify the dev report verbosity" + "\n" +
"\t atsReport" + "\t\t" + "(0: disable, 1: standard, 2: detailed, 3: 2 + screenshots" + "\n" +
"\n\n\n\n");
}
public static void initFolders(Path projectPath) {
try {
projectPath.resolve(REPORT_FOLDER).toFile().mkdirs();
projectPath.resolve(REPORT_FOLDER).resolve(CUSTOM_TEMPLATES_FOLDER).toFile().mkdirs();
projectPath.resolve(REPORT_FOLDER).resolve(CUSTOM_IMAGES_FOLDER).toFile().mkdirs();
} catch (Exception e) {
}
}
private void copyReportsTemplate(Path path, Path toPath) {
if (Files.exists(path)) {
for (File f : path.toFile().listFiles()) {
if (f.isFile()) {
try {
Files.copy(f.toPath(), toPath.resolve(f.getName()), StandardCopyOption.REPLACE_EXISTING);
} catch (IOException e) {
}
}
}
}
}
public CampaignReportGenerator(Path outputFolderPath, File jsonSuiteFilesFile, int devReportLevel)
throws IOException, TransformerException, ParserConfigurationException, SAXException {
MemoryMonitor memoryMonitor = new MemoryMonitor();
memoryMonitor.startMonitoring();
reportGenerationStartTime = System.nanoTime();
Timer timer = AdvancedAtsLogger.displayMessageWithPeriodExecutionTimeAndMemoryUsed("report generation in progress -> ", 30, reportGenerationStartTime);
LinkedList reports = new LinkedList<>();
final Path reportPath = Paths.get(
com.ats.script.Project.SRC_FOLDER,
com.ats.script.Project.ASSETS_FOLDER,
com.ats.script.Project.RESOURCES_FOLDER,
REPORT_FOLDER);
if (Files.exists(reportPath)) {
copyReportsTemplate(reportPath.resolve(TEMPLATE_FOLDER), outputFolderPath);
copyReportsTemplate(reportPath.resolve(IMAGE_FOLDER), outputFolderPath);
}
SuitesReport suiteReport = null;
try {
final JsonReader reader = new JsonReader(new FileReader(jsonSuiteFilesFile));
suiteReport = new Gson().fromJson(reader, SuitesReport.class);
reader.close();
} catch (IOException e) {
}
if (suiteReport == null) {
System.out.println("no suites found, nothing to do !");
return;
}
final Path outputPath = outputFolderPath.toAbsolutePath();
//-----------------------------------------------------------------------------------------------------
// Clean files
//-----------------------------------------------------------------------------------------------------
File[] filesToDelete = outputFolderPath.toFile().listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
return name.endsWith(".jrprint") || name.endsWith(".jasper") || name.endsWith(".pdf");
}
});
for (File f : filesToDelete) {
try {
f.delete();
System.out.println("file: " + f.getAbsolutePath());
} catch (Exception e) {}
}
//-----------------------------------------------------------------------------------------------------
// Build Jasper
//-----------------------------------------------------------------------------------------------------
final Path outputJasperFolder = outputFolderPath.resolve("ats-jasper");
try {
Utils.deleteRecursive(outputJasperFolder.toFile());
}catch(FileNotFoundException e) {}
Files.createDirectory(outputJasperFolder);
copyResource("test.jrxml", outputJasperFolder);
copyResource("suite.jrxml", outputJasperFolder);
copyResource("summary.jrxml", outputJasperFolder);
copyResource("ats-test.jrxml", outputJasperFolder);
copyResource("ats-test-action.jrxml", outputJasperFolder);
try {
JasperCompileManager.compileReportToFile(outputJasperFolder.resolve("test.jrxml").toString(), outputJasperFolder.resolve("test.jasper").toString());
JasperCompileManager.compileReportToFile(outputJasperFolder.resolve("suite.jrxml").toString(), outputJasperFolder.resolve("suite.jasper").toString());
JasperCompileManager.compileReportToFile(outputJasperFolder.resolve("summary.jrxml").toString(), outputJasperFolder.resolve("summary.jasper").toString());
JasperCompileManager.compileReportToFile(outputJasperFolder.resolve("ats-test.jrxml").toString(), outputJasperFolder.resolve("ats-test.jasper").toString());
JasperCompileManager.compileReportToFile(outputJasperFolder.resolve("ats-test-action.jrxml").toString(), outputJasperFolder.resolve("ats-test-action.jasper").toString());
AtsLogger.printLog("JASPER reports templates generated");
}catch(JRException e) {
AtsLogger.printLog("error generating jasper files -> " + e.getMessage());
}
//-----------------------------------------------------------------------------------------------------
// Consolidate data
//-----------------------------------------------------------------------------------------------------
DocumentBuilder builder = DocumentBuilderFactory.newInstance().newDocumentBuilder();
Document writeXmlDocument = builder.newDocument();
final Element report = writeXmlDocument.createElement(XML_SOURCE_ROOT);
report.setAttribute("atsVersion", ATS.getAtsVersion());
report.setAttribute("projectId", suiteReport.projectId);
report.setAttribute("projectUuid", suiteReport.projectUuid);
report.setAttribute("projectName", suiteReport.projectName);
report.setAttribute("projectVersion", suiteReport.projectVersion);
report.setAttribute("projectDescription", suiteReport.projectDescription);
report.setAttribute("path", outputFolderPath.toFile().toURI().toASCIIString());
report.setAttribute("started", String.valueOf(suiteReport.started));
report.setAttribute("startedFormated", OffsetDateTime.ofInstant(Instant.ofEpochMilli(suiteReport.started), ZoneId.systemDefault()).format(DateTimeFormatter.ofPattern("yyyy-MM-dd 'at' HH:mm:ss")));
report.setAttribute("startedFormatedZulu", OffsetDateTime.ofInstant(Instant.ofEpochMilli(suiteReport.started), ZoneOffset.UTC).toString());
writeXmlDocument.appendChild(report);
final Element picsList = writeXmlDocument.createElement("pics");
final String[] defaultImages = new String[]{"logo.png", "true.png", "false.png", "warning.png", "noStop.png", "api.png", "pdf.png",
"external_link_10_white.png","external_link_12_blue.png","external_link_12_blue_visited.png","external_link_12_grey.png","external_link_16_grey.png","external_link_16_white.png","run_16.png"};
for (String img : defaultImages) {
final Element pic = writeXmlDocument.createElement("pic");
pic.setAttribute("name", img.replace(".png", ""));
byte[] imgBytes = null;
if (Files.exists(outputFolderPath.resolve(img))) {
imgBytes = Files.readAllBytes(outputFolderPath.resolve(img));
} else {
imgBytes = ResourceContent.class.getResourceAsStream(CUSTOM_IMAGES_FOLDER + img).readAllBytes();
}
pic.setTextContent("data:image/png;base64," + getBase64DefaultImages(imgBytes));
picsList.appendChild(pic);
}
report.appendChild(picsList);
report.setAttribute("suitesCount", String.valueOf(suiteReport.suites.length));
int totalTestsPassed = 0;
int totalTestsFailed = 0;
int totalSuitesPassed = 0;
int totalActions = 0;
int totalDuration = 0;
final String SATSReport = System.getProperty(ATS_REPORT);
// final String SMGTReport = System.getProperty(MGT_REPORT); // not used
final String SVALIDReport = System.getProperty(VALID_REPORT);
final String SATSReportEnv = System.getenv(ATS_REPORT_ENV);
String devReportLVL = "0";
if (SATSReportEnv != null && !"0".equals(SATSReportEnv) ) {
int tmp = Utils.string2Int(SATSReportEnv,0);
if(( tmp > 0) && tmp < 4){
devReportLVL = SATSReportEnv;
}else{
System.out.println("[WARN] incorrect env variable value [ATS_REPORT] : " + SATSReportEnv);
}
}
if (SATSReport != null && !"0".equals(SATSReport) ) {
int tmp = Utils.string2Int(SATSReport,0);
if(( tmp > 0) && tmp < 4){
devReportLVL = SATSReport;
}else{
System.out.println("[WARN] incorrect parameters value [ats-report] : " + SATSReport);
}
}else{
devReportLVL = Integer.toString(details);
}
report.setAttribute("devReportLevel", devReportLVL);
report.setAttribute("validReportLevel", SVALIDReport);
for (SuitesReportItem info : suiteReport.suites) {
final Path suitePath = outputFolderPath.resolve(info.getName());
boolean suitePassed = true;
final Element suite = writeXmlDocument.createElement("suite");
suite.setAttribute("name", info.getName());
suite.setAttribute("started", String.valueOf(System.currentTimeMillis()));
int devReport = 0;
devReport = Utils.string2Int(SATSReport, details);
int validReport = 0;
if (SVALIDReport != null) {
validReport = trueList.indexOf(SVALIDReport.toLowerCase()) > -1 ? 1 : 0;
}
// ordre de prépondérance :
// - paramètres de Suites
// - variable d'environnement
// - ligne de commande
if (SATSReport != null && !"0".equals(SATSReport)) {
devReport = Utils.string2Int(SATSReport, 0);
if (devReport == 0) {
System.out.println("cmd parameter value error [ats-report] : " + SATSReport);
}
} else if (SATSReportEnv != null && !"0".equals(SATSReportEnv)) {
devReport = Utils.string2Int(SATSReportEnv, 0);
if (devReport == 0) {
System.out.println("env parameter value error [ATS_REPORT] : " + SATSReportEnv);
}
} else if (!"0".equals(info.devReportLvl) ) {
devReport = Utils.string2Int(info.devReportLvl, details);
if (devReport == 0) {
System.out.println("[ERROR] suite parameter value [ats.report.dev.level] : " + info.devReportLvl);
}
}
if (SVALIDReport == null || !"0".equals(SVALIDReport)) {
if (!"".equals(info.validReportLvl) && validReport == 0) {
if ("true".equals(info.validReportLvl)) {
validReport = 1;
}
}
}
String reportName = "suites" + TEMPLATE_EXTENSION;
if (devReport != 0 && (!reports.contains(reportName))) {
reports.add(reportName);
}
reportName = VALID_REPORT + TEMPLATE_EXTENSION;
if (validReport != 0 && (!reports.contains(reportName))) {
reports.add(reportName);
}
suite.setAttribute("description", info.getDescription());
suite.setAttribute("dateOrder", info.getDateOrder());
final Element parameters = writeXmlDocument.createElement("parameters");
suite.appendChild(parameters);
final Properties properties = new Properties();
final Path suiteParamPath = suitePath.resolve(ActionTestScript.SUITE_PARAMETERS);
if (Files.exists(suiteParamPath)) {
properties.load(Files.newBufferedReader(suiteParamPath));
}
final Element imageQuality = writeXmlDocument.createElement("parameter");
imageQuality.setAttribute("name", SuitesReportItem.IMAGE_QUALITY);
imageQuality.setAttribute("value", String.valueOf(info.getVisualQualityLabel()));
parameters.appendChild(imageQuality);
if(info.getDateOrder() != null && info.getDateOrder().length() > 0) {
final Element dateOrder = writeXmlDocument.createElement("parameter");
dateOrder.setAttribute("name", SuitesReportItem.DATE_ORDER);
dateOrder.setAttribute("value", String.valueOf(info.getDateOrder()));
parameters.appendChild(dateOrder);
}
for (Map.Entry entry : info.getParametersEntries()) {
final Element parameter = writeXmlDocument.createElement("parameter");
parameter.setAttribute("name", entry.getKey());
parameter.setAttribute("value", properties.getProperty(entry.getKey(), entry.getValue()));
parameter.setAttribute("defaultValue", entry.getValue());
parameters.appendChild(parameter);
}
final Element groups = writeXmlDocument.createElement("groups");
for (String group : info.getIncludedGroups()) {
final Element groupXml = writeXmlDocument.createElement("included");
groupXml.setTextContent(group);
groups.appendChild(groupXml);
}
for (String group : info.getExcludedGroups()) {
final Element groupXml = writeXmlDocument.createElement("excluded");
groupXml.setTextContent(group);
groups.appendChild(groupXml);
}
suite.appendChild(groups);
final Element tests = writeXmlDocument.createElement("tests");
suite.appendChild(tests);
int testsPassed = 0;
int testsFailed = 0;
int actionsExecuted = 0;
int suiteDuration = 0;
suite.setAttribute("testsCount", String.valueOf(info.getTestsCount()));
suite.setAttribute("started", String.valueOf(info.getStarted()));
suite.setAttribute("startedFormated", OffsetDateTime.ofInstant(Instant.ofEpochMilli(info.getStarted()), ZoneId.systemDefault()).format(DateTimeFormatter.ofPattern("yyyy-MM-dd 'at' HH:mm:ss")));
suite.setAttribute("startedFormatedZulu", OffsetDateTime.ofInstant(Instant.ofEpochMilli(info.getStarted()), ZoneOffset.UTC).toString());
final SimpleHtmlExporterConfiguration configuration = new SimpleHtmlExporterConfiguration();
configuration.setHtmlHeader(HTML_HEADER);
for (String className : info.tests) {
final Path testResultPath = suitePath.resolve(className + "_xml");
final File xmlDataFile = testResultPath.resolve(XmlReport.REPORT_DATA_FILE).toFile();
if (xmlDataFile.exists() && xmlDataFile.isFile()) {
int testDuration = 0;
final Element atsTest = builder.parse(xmlDataFile).getDocumentElement();
final NodeList actionsList = atsTest.getElementsByTagName("action");
int testActionsExecuted = actionsList.getLength();
final NodeList summary = atsTest.getElementsByTagName("summary");
atsTest.setAttribute("devReportLVL", devReportLVL);
if (summary.getLength() > 0) {
if ("1".equals(summary.item(0).getAttributes().getNamedItem("status").getNodeValue())) {
testsPassed++;
totalTestsPassed++;
} else {
testsFailed++;
totalTestsFailed++;
suitePassed = false;
}
testActionsExecuted = Utils.string2Int(summary.item(0).getAttributes().getNamedItem("actions").getNodeValue(), testActionsExecuted);
}
actionsExecuted += testActionsExecuted;
for (int i = 0; i < actionsList.getLength(); i++) {
final Node action = actionsList.item(i);
Element eElement = (Element) action;
Node element = null;
if ((element = eElement.getElementsByTagName("duration").item(0)) != null) {
testDuration += Utils.string2Int(element.getTextContent());
}
element = null;
}
atsTest.setAttribute("duration", String.valueOf(testDuration));
suiteDuration += testDuration;
tests.appendChild(writeXmlDocument.importNode(atsTest, true));
final String atsTestReportPath = testResultPath.resolve(ATS_TEST_REPORT_HTML).toString();
try {
NodeList imgs = atsTest.getElementsByTagName("img");
String agilitestTempResourcesPath;
String xmlDataFileCopyPath;
try {
FileUtils.clearTempDir(AGILITEST_RESOURCES_PREFIX);
agilitestTempResourcesPath = Files.createTempDirectory(AGILITEST_RESOURCES_PREFIX).toFile().getAbsolutePath();
Path copied = Paths.get(agilitestTempResourcesPath + File.separator + xmlDataFile.getName());
Path original = Paths.get(xmlDataFile.getPath());
xmlDataFileCopyPath = Files.copy(original, copied, StandardCopyOption.REPLACE_EXISTING).toString();
} catch (IOException e) {
throw new RuntimeException(e);
}
ImageProcessingUtils.processImages(imgs, agilitestTempResourcesPath);
Transformer transformer = TransformerFactory.newInstance().newTransformer();
Result output = new StreamResult(new File(xmlDataFileCopyPath));
Source input = new DOMSource(atsTest);
transformer.transform(input, output);
AtsLogger.printLog("create ats-test report -> " + atsTestReportPath);
JRSwapFile swapFile = new JRSwapFile(System.getProperty("java.io.tmpdir"), 1024, 1024);
JRSwapFileVirtualizer virtualizer = new JRSwapFileVirtualizer(2, swapFile, true);
final Map testReportParameters = new HashMap<>();
testReportParameters.put("xmlSource", xmlDataFileCopyPath);
testReportParameters.put("reportLevel",devReportLVL);
testReportParameters.put(JRParameter.REPORT_VIRTUALIZER, virtualizer);
final JasperPrint jasperPrint = JasperFillManager.fillReport(outputJasperFolder.resolve("ats-test.jasper").toString(), testReportParameters);
final HtmlExporter exporter = new HtmlExporter();
exporter.getPropertiesUtil().setProperty("net.sf.jasperreports.export.html.embed.image", "true");
final SimpleHtmlExporterOutput outputHtml = new SimpleHtmlExporterOutput(atsTestReportPath);
exporter.setExporterInput(new SimpleExporterInput(jasperPrint));
exporter.setExporterOutput(outputHtml);
exporter.setConfiguration(configuration);
exporter.exportReport();
outputHtml.close();
File agilitestTempResourceFile = Path.of(agilitestTempResourcesPath).toFile();
ImageProcessingUtils.processVideos(atsTestReportPath, agilitestTempResourceFile, agilitestTempResourcesPath);
FileUtils.deleteFolder(agilitestTempResourceFile);
} catch (JRException e) {
e.printStackTrace();
}
}
}
totalActions += actionsExecuted;
totalDuration += suiteDuration;
if (suitePassed) {
totalSuitesPassed++;
}
suite.setAttribute("passed", String.valueOf(suitePassed));
suite.setAttribute("duration", String.valueOf(suiteDuration));
suite.setAttribute("actions", String.valueOf(actionsExecuted));
suite.setAttribute("testsPassed", String.valueOf(testsPassed));
suite.setAttribute("testsFailed", String.valueOf(testsFailed));
final TestsResultBarChart bar = new TestsResultBarChart(testsPassed, testsFailed, info.getTestsCount()-testsPassed-testsFailed);
suite.setAttribute("resultBar", bar.getBase64());
report.appendChild(suite);
}
report.setAttribute("duration", String.valueOf(totalDuration));
report.setAttribute("tests", String.valueOf(totalTestsPassed + totalTestsFailed));
report.setAttribute("testsPassed", String.valueOf(totalTestsPassed));
report.setAttribute("testsFailed", String.valueOf(totalTestsFailed));
report.setAttribute("suitesPassed", String.valueOf(totalSuitesPassed));
report.setAttribute("actions", String.valueOf(totalActions));
final Path atsXmlDataPath = outputFolderPath.resolve(XML_SOURCE_NAME);
Transformer transformer = TransformerFactory.newInstance().newTransformer();
transformer.transform(new DOMSource(writeXmlDocument),
new StreamResult(new OutputStreamWriter(
new FileOutputStream(atsXmlDataPath.toFile()),
StandardCharsets.UTF_8)));
for (String reportFile : reports) {
final File htmlTemplateFile = copyResource(reportFile, outputFolderPath);
MinifyWriter filteredWriter = null;
Transformer htmlTransformer = null;
if ("suites_html.xml".equals(reportFile)) {
filteredWriter = new MinifyWriter(
Files.newBufferedWriter(outputFolderPath.resolve(CampaignReportGenerator.ATS_REPORT_HTML), StandardCharsets.UTF_8));
htmlTransformer = TransformerFactory.newInstance().newTransformer(new StreamSource(htmlTemplateFile));
} else {
filteredWriter = new MinifyWriter(
Files.newBufferedWriter(outputFolderPath.resolve(reportFile.substring(0, reportFile.indexOf("_")) + ".html"), StandardCharsets.UTF_8));
htmlTransformer = TransformerFactory.newInstance().newTransformer(new StreamSource(htmlTemplateFile));
}
htmlTransformer.setOutputProperty(OutputKeys.ENCODING, "UTF-8");
htmlTransformer.transform(
new DOMSource(builder.parse(new InputSource(
new InputStreamReader(Files.newInputStream(atsXmlDataPath), StandardCharsets.UTF_8)))),
new StreamResult(filteredWriter));
filteredWriter.close();
}
// if (jasper != null) {
// final File jasperFolder = new File(jasper);
// if (jasperFolder.exists()) {
//
// final String jasperFolderPath = jasperFolder.getAbsolutePath();
// AtsLogger.printLog("Jasper folder -> " + jasperFolderPath);
//
// copyResource("summary.jrxml", outputFolderPath);
// copyResource("suite.jrxml", outputFolderPath);
// copyResource("test.jrxml", outputFolderPath);
//
// final String outputPath = outputFolderPath.toAbsolutePath().toString();
// final String reportName = "summary";
//
// //-----------------------------------------------------------------------------------------------------
// // Clean files
// //-----------------------------------------------------------------------------------------------------
//
// File[] filesToDelete = outputFolderPath.toFile().listFiles(new FilenameFilter() {
// @Override
// public boolean accept(File dir, String name) {
// return name.endsWith(".jrprint") || name.endsWith(".jasper") || name.equals(reportName + ".pdf");
// }
// });
//
// System.out.println("Delete files.");
// for (File f : filesToDelete) {
// try {
// f.delete();
// System.out.println("File : " + f.getAbsolutePath());
// } catch (Exception e) {
// }
// }
//
// //-----------------------------------------------------------------------------------------------------
// // Build Jasper reports
// //-----------------------------------------------------------------------------------------------------
//
// builder = DocumentBuilderFactory.newInstance().newDocumentBuilder();
// writeXmlDocument = builder.newDocument();
//
// final Element project = writeXmlDocument.createElement("project");
// project.setAttribute("default", "run");
// project.setAttribute("basedir", outputPath);
//
// final Element path = writeXmlDocument.createElement("path");
// path.setAttribute("id", "classpath");
//
// final Element fileset = writeXmlDocument.createElement("fileset");
// fileset.setAttribute("dir", jasperFolderPath);
//
// final Element include = writeXmlDocument.createElement("include");
// include.setAttribute("name", "**/*.jar");
//
// fileset.appendChild(include);
// path.appendChild(fileset);
// project.appendChild(path);
//
// final Element taskdef = writeXmlDocument.createElement("taskdef");
// taskdef.setAttribute("name", "jrc");
// taskdef.setAttribute("classname", "net.sf.jasperreports.ant.JRAntCompileTask");
//
// final Element classpath = writeXmlDocument.createElement("classpath");
// classpath.setAttribute("refid", "classpath");
// taskdef.appendChild(classpath);
// project.appendChild(taskdef);
//
// final Element target = writeXmlDocument.createElement("target");
// target.setAttribute("name", "run");
//
// final Element jrc = writeXmlDocument.createElement("jrc");
// jrc.setAttribute("destdir", outputPath);
// jrc.setAttribute("xmlvalidation", "false");
//
// final Element src = writeXmlDocument.createElement("src");
// final Element fileset7 = writeXmlDocument.createElement("fileset");
// fileset7.setAttribute("dir", outputPath);
//
// final Element include2 = writeXmlDocument.createElement("include");
// include2.setAttribute("name", "**/*.jrxml");
// fileset7.appendChild(include2);
// src.appendChild(fileset7);
//
// jrc.appendChild(src);
//
// final Element classpath2 = writeXmlDocument.createElement("classpath");
// classpath2.setAttribute("refid", "classpath");
// jrc.appendChild(classpath2);
//
// target.appendChild(jrc);
//
// project.appendChild(target);
//
// final File buildFile = outputFolderPath.resolve("build-report.xml").toFile();
//
// transformer = TransformerFactory.newInstance().newTransformer();
// transformer.transform(new DOMSource(project),
// new StreamResult(new OutputStreamWriter(
// new FileOutputStream(buildFile),
// StandardCharsets.UTF_8)));
//
//
// //-----------------------------------------------------------------------------------------------------
// // Launch Ant task to build reports
// //-----------------------------------------------------------------------------------------------------
//
// final Project p = new Project();
//
// p.setUserProperty("ant.file", buildFile.getAbsolutePath());
//
// p.init();
//
// final ProjectHelper helper = ProjectHelper.getProjectHelper();
// p.addReference("ant.projectHelper", helper);
// helper.parse(p, buildFile);
//
// final DefaultLogger consoleLogger = new DefaultLogger();
// consoleLogger.setErrorPrintStream(System.err);
// consoleLogger.setOutputPrintStream(System.out);
// consoleLogger.setEmacsMode(false);
// consoleLogger.setMessageOutputLevel(Project.MSG_INFO);
//
// p.addBuildListener(consoleLogger);
// p.executeTarget(p.getDefaultTarget());
//
// //-----------------------------------------------------------------------------------------------------
// // Generate pdf report
// //-----------------------------------------------------------------------------------------------------
//
// AtsLogger.printLog("Generate reports.");
//
// try {
// generatePdf(reportName, outputPath, jasperFolder);
// } catch (ClassNotFoundException | IllegalAccessException | IllegalArgumentException |
// InvocationTargetException | NoSuchMethodException | SecurityException e) {
// e.printStackTrace();
// } catch (JRException e) {
// // TODO Auto-generated catch block
// e.printStackTrace();
// }
// }
// }else {
try {
final String reportFullName = outputPath.resolve("summary.pdf").toString();
final Map parameters = new HashMap<>();
parameters.put("workingDir", outputJasperFolder.toString());
parameters.put("xmlSource", outputPath.resolve(XML_SOURCE_NAME).toString());
parameters.put("xmlSourceRoot", XML_SOURCE_ROOT);
String fileName = JasperFillManager.fillReportToFile(outputJasperFolder.resolve("summary.jasper").toString(), parameters);
JasperExportManager.exportReportToPdfFile(fileName, reportFullName);
AtsLogger.printLog("PDF file -> " + reportFullName + " ... OK");
AtsLogger.printLog("report generated in " + (System.nanoTime() - reportGenerationStartTime)/1000000000 + " second(s)");
timer.cancel();
long maxMemoryUsed = memoryMonitor.stopMonitoring();
AtsLogger.printLog("maximum memory used during report generation -> " + maxMemoryUsed + " MB");
} catch (JRException e) {
e.printStackTrace();
}
//}
}
private static File copyResource(String resName, Path dest) throws IOException {
Path filePath = dest.resolve(resName);
if (!Files.exists(filePath)) {
InputStream is = ResourceContent.class.getResourceAsStream(CUSTOM_TEMPLATES_FOLDER + resName);
InputStream in = new BufferedInputStream(is);
File targetFile = dest.resolve(resName).toFile();
OutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile));
byte[] buffer = new byte[1024];
int lengthRead;
while ((lengthRead = in.read(buffer)) > 0) {
out.write(buffer, 0, lengthRead);
out.flush();
}
in.close();
is.close();
out.close();
}
return filePath.toFile();
}
private static String getBase64DefaultImages(byte[] b) throws IOException {
return Base64.getEncoder().encodeToString(b);
}
/*public static void saveB64Pic(Path imagePath) throws FileNotFoundException, IOException {
byte[] encoded = null;
if (Files.exists(imagePath)) {
try {
final File f = new File(imagePath.toString());
final FileInputStream fileInputStreamReader = new FileInputStream(f);
final byte[] fileContent = new byte[(int) f.length()];
fileInputStreamReader.read(fileContent);
encoded = Base64.getEncoder().encode(fileContent);
fileInputStreamReader.close();
} catch (IOException e) {
e.printStackTrace();
}
} else {
return;
}
File outputFile = new File(imagePath.toString().substring(0, imagePath.toString().lastIndexOf(".")) + ".b64");
try (FileOutputStream outputStream = new FileOutputStream(outputFile)) {
outputStream.write("data:image/png;base64,".getBytes());
outputStream.write(encoded);
outputStream.write(" ".getBytes());
}
}*/
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy