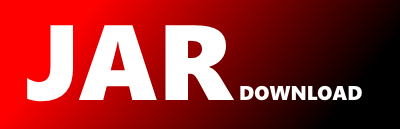
com.ats.tools.report.SuitesReportItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report;
import java.io.File;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import org.testng.TestRunner;
import org.testng.xml.XmlClass;
import org.testng.xml.XmlSuite;
import org.testng.xml.XmlTest;
import com.ats.AtsSingleton;
import com.ats.tools.Utils;
import com.google.common.collect.ImmutableMap;
import com.google.gson.JsonObject;
public class SuitesReportItem {
public static final String CALLSCRIPT_ITERATION = "ats-callscript-iteration";
public static final String CALLSCRIPT_PARAMETER_FILE = "ats-callscript-parameters";
public static final String ITERATION_PROPERTY = "ats-iteration";
public static final String DATA_FILE = "ats-data-file";
public static final String IMAGE_QUALITY = "ats-image-quality";
public static final String DATE_ORDER = "ats-date-order";
private static final String ATS_LOG_LEVEL = "ats.log.level";
private static final String ATS_REPORT_IMAGE_QUALITY = "visual.report";
private static final String ATSV_HTML = "atsv.html";
private static final String ATS_REPORT_DEV_LEVEL = "ats.report.dev.level";
private static final String ATS_REPORT_MGT_LEVEL = "ats.report.mgt.level";
private static final String ATS_REPORT_VALID_LEVEL = "ats.report.validation.level";
private static final String ATS_SUITE_DESCRIPTION = "ats.suite.description";
private static final String ATS_SUITE_DATE_ORDER = "ats.suite.date.order";
public String devReportLvl = "";
public String mgtReportLvl = "";
public String validReportLvl = "";
public List tests;
private String name;
public String getName() {
return name;
}
private String description = "";
public String getDescription() {
return description;
}
private void setDescription(String value) {
if(value == null) {
value = "";
}
description = value;
}
private int visualQuality = 3;
public int getVisualQuality() {
return visualQuality;
}
public String getVisualQualityLabel() {
switch (visualQuality){
case 1:
return "size-optimized";
case 2 :
return "speed-optimized";
case 3:
return "quality";
case 4 :
return "max-quality";
}
return "undefined";
}
public void setVisualQuality(String value) {
visualQuality = Utils.string2Int(value, 3);
}
private boolean atsvHtml = false;
public boolean isAtsvHtml() {
return atsvHtml;
}
public void setAtsvHtml(String value) {
atsvHtml = "true".equalsIgnoreCase(value);
}
private String dateOrder = "";
public String getDateOrder() {
return dateOrder.toUpperCase();
}
private void setDateOrder(String value) {
if(value == null) {
value = "";
}
dateOrder = value;
}
private Map parameters;
public Set> getParametersEntries() {
return parameters.entrySet();
}
private List includedGroups = new ArrayList<>();
public List getIncludedGroups() {
return includedGroups;
}
private List excludedGroups = new ArrayList<>();
public List getExcludedGroups() {
return excludedGroups;
}
private boolean reporting = false;
private transient String ouputDir;
private transient String projectId;
private transient String projectUuid;
private transient boolean subScriptIteration = false;
public boolean isSubScriptIteration() {
return subScriptIteration;
}
private long started;
public SuitesReportItem(String projectUuid, String projectId, TestRunner runner) {
this(runner);
this.projectUuid = projectUuid;
this.projectId = projectId;
this.name = runner.getSuite().getName();
final XmlSuite xmlSuite = runner.getSuite().getXmlSuite();
if (xmlSuite.getGroups() != null) {
this.includedGroups = xmlSuite.getGroups().getRun().getIncludes();
this.excludedGroups = xmlSuite.getGroups().getRun().getExcludes();
}
this.ouputDir = runner.getOutputDirectory();
tests = new ArrayList<>();
if (subScriptIteration) {
for (XmlTest test : xmlSuite.getTests()) {
final List classes = test.getClasses();
if (classes.size() > 0) {
final XmlClass xmlTestClass = classes.get(0);
tests.add(xmlTestClass.getName() + "-" + test.getName());
}
}
} else {
for (XmlTest test : xmlSuite.getTests()) {
final List classes = test.getClasses();
for (XmlClass cla : classes) {
tests.add(cla.getName());
}
}
}
}
public SuitesReportItem(TestRunner runner) {
this.started = System.currentTimeMillis();
String logLevel = "";
final String scriptIteration = runner.getTest().getAllParameters().get(CALLSCRIPT_ITERATION);
if (scriptIteration != null) {
subScriptIteration = true;
logLevel = runner.getSuite().getXmlSuite().getParameter(ATS_LOG_LEVEL);
final Map inheritedParameters = runner.getTest().getAllParameters();
setVisualQuality(inheritedParameters.get(ATS_REPORT_IMAGE_QUALITY));
setAtsvHtml(inheritedParameters.get(ATSV_HTML));
setDateOrder(inheritedParameters.get(ATS_SUITE_DATE_ORDER));
setDescription("Callscript as a suite = " + scriptIteration);
parameters = ImmutableMap.of(DATA_FILE, inheritedParameters.get(CALLSCRIPT_PARAMETER_FILE));
} else {
this.parameters = runner.getTest().getAllParameters();
if (this.parameters != null) {
logLevel = parameters.remove(ATS_LOG_LEVEL);
setDevLevel(parameters.remove(ATS_REPORT_DEV_LEVEL));
setMgtLevel(parameters.remove(ATS_REPORT_MGT_LEVEL));
setValidLevel(parameters.remove(ATS_REPORT_VALID_LEVEL));
setVisualQuality(parameters.remove(ATS_REPORT_IMAGE_QUALITY));
setAtsvHtml(parameters.remove(ATSV_HTML));
setDateOrder(parameters.remove(ATS_SUITE_DATE_ORDER));
setDescription(parameters.remove(ATS_SUITE_DESCRIPTION));
}
}
AtsSingleton.getInstance().setLoglevel(logLevel);
}
public String getProjectId() {
return projectId;
}
public String getProjectUuid() {
return projectUuid;
}
public long getStarted() {
return started;
}
public String getStartLog() {
final JsonObject logs = new JsonObject();
logs.addProperty("name", name);
logs.addProperty("tests", tests.size());
return "suite started -> " + logs.toString();
}
public File getSuitesFile() {
return Paths.get(ouputDir).getParent().resolve(CampaignReportGenerator.ATS_JSON_SUITES).toFile();
}
private void setDevLevel(String value) {
if (value != null) {
devReportLvl = value;
if (!"0".equals(devReportLvl)) {
reporting = true;
}
}
}
private void setMgtLevel(String value) {
if (value != null) {
mgtReportLvl = value;
if (!"0".equals(mgtReportLvl)) {
reporting = true;
}
}
}
private void setValidLevel(String value) {
if (value != null) {
validReportLvl = value;
if (!"0".equals(validReportLvl)) {
reporting = true;
}
}
}
public int getTestsCount() {
return tests.size();
}
public boolean isReporting() {
return reporting;
}
public void setReporting(boolean reporting) {
this.reporting = reporting;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy