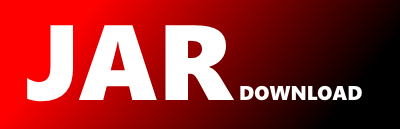
com.ats.tools.report.actions.HtmlReportAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.actions;
import static com.ats.tools.report.utils.HtmlActionUtils.getActionUrl;
import java.util.Map;
import com.ats.tools.ResourceContent;
import com.ats.tools.Utils;
import com.ats.tools.logger.levels.AtsLogger;
import com.ats.tools.report.actions.HtmlReportActionAssert.ActionAssertOperation;
import com.ats.tools.report.actions.HtmlReportActionChannel.ActionChannelOperation;
import com.ats.tools.report.models.Action;
import com.ats.tools.report.models.Image;
import com.ats.tools.report.models.TestInfo;
import com.ats.tools.report.utils.HtmlReportActionLabel;
public class HtmlReportAction {
public static final String STATUS_LINE_STYLE = "${statusLineStyle}";
public static final String ACTION_NAME_STYLE = "${actionNameStyle}";
public static final String ACTION_ID = "${actionId}";
public static final String RESULT_ICON = "${result-icon}";
public static final String ACTION_NAME = "${actionName}";
public static final String SCRIPT_NAME = "${scriptName}";
public static final String LINE = "${line}";
public static final String EXECUTION_TIME_MIL = "${executionTimeMil}";
public static final String EXECUTION_TIME_MIN = "${executionTimeMin}";
public static final String EXECUTION_TIME_SEC = "${executionTimeSec}";
private static final String ID_HIDDEN_PLACEHOLDER_MIN = "${idHiddenPlaceHolderMin}";
private static final String ID_HIDDEN_PLACEHOLDER_SEC = "${idHiddenPlaceHolderSec}";
private static final String ID_HIDDEN_PLACEHOLDER_MIL = "${idHiddenPlaceHolderMil}";
private static final String ACTION_LABEL_CLASS = "${actionLabelClass}";
private static final String ACTION_LABEL = "${actionLabel}";
public static final String MEDIA_DATA = "${mediaData}";
public static final String ELEMENT = "${element}";
public static final String ACTION_BODY = "${actionBody}";
public static final String DURATION_IDENTIFICATION_CLASS = "${durationIdentificationClass}";
public static final String MEDIA_DATA_HTML_TEMPLATE = "<${mediaTag} class='data-image' ${controls} src='data:${contentType}/${mediaType};base64,${mediaSrc}' alt='Image'>${mediaTag}>";
public static final String TEST_SCRIPT_HTML_TEMPLATE = "${scriptName} ( line ${line} )";
private String actionTemplate = ResourceContent.getHtmlReportActionHtmlTemplate();
private final String oneElementDataTemplate = ResourceContent.getHtmlReportOneElementDataActionTemplate();
private final String twoElementDataTemplate = ResourceContent.getHtmlReportTwoElementsDataActionTemplate();
private final String threeElementDataTemplate = ResourceContent.getHtmlReportThreeElementsDataActionTemplate();
private final String fiveElementDataTemplate = ResourceContent.getHtmlReportApiCallActionTemplate();
private final String actionAssertPropertyTemplate = ResourceContent.getHtmlReportAssertPropertyActionTemplate();
private final String actionAssertOccurrenceTemplate = ResourceContent.getHtmlReportAssertOccurrenceActionTemplate();
private final String actionAssertValueTemplate = ResourceContent.getHtmlReportAssertValueActionTemplate();
public HtmlReportAction() {}
public String processAction(Action action, Map appIcons, TestInfo testInfo, int reportLevel) {
actionTemplate = actionTemplate.replace(SCRIPT_NAME, buildScriptName(action, testInfo));
actionTemplate = actionTemplate.replace(LINE, String.valueOf(action.getLine()));
HtmlReportActionLabel htmlReportActionLabel = new HtmlReportActionLabel(action.getType());
actionTemplate = actionTemplate.replace(ACTION_LABEL_CLASS, htmlReportActionLabel.getCssClass());
actionTemplate = actionTemplate.replace(ACTION_LABEL, htmlReportActionLabel.getLabel());
if (action.getDuration() < 1000) {
this.actionTemplate = actionTemplate.replace(EXECUTION_TIME_MIL, action.getDuration() % 1000 + " ms");
this.actionTemplate = actionTemplate.replace(ID_HIDDEN_PLACEHOLDER_MIN, "hidden");
this.actionTemplate = actionTemplate.replace(ID_HIDDEN_PLACEHOLDER_SEC, "hidden");
} else {
this.actionTemplate = actionTemplate.replace(ID_HIDDEN_PLACEHOLDER_MIL, "hidden");
this.actionTemplate = actionTemplate.replace(EXECUTION_TIME_SEC, Utils.getSecondsForTimeline((long) action.getDuration()) + " sec");
this.actionTemplate = actionTemplate.replace(EXECUTION_TIME_MIN, action.getDuration() / 60000 + " min");
}
actionTemplate = actionTemplate.replace(DURATION_IDENTIFICATION_CLASS, action.getScript() + "-" + action.getLine() + "-" + action.getDuration());
if (reportLevel == 3) {
String mediaData = buildMediaData(action.getImage());
actionTemplate = actionTemplate.replace(MEDIA_DATA, mediaData);
} else {
actionTemplate = actionTemplate.replace("${imageVisibilityPlaceHolder}", "style='display: none;'");
}
if (action.getType().equals("ActionComment")) {
actionTemplate = actionTemplate.replace(ACTION_NAME, action.getType());
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-non-functional");
actionTemplate = actionTemplate.replace(ACTION_NAME_STYLE, "action-name-non-functional");
actionTemplate = actionTemplate.replace(RESULT_ICON, "result-non-icon");
actionTemplate = actionTemplate.replace("element", "result-non-icon");
} else {
actionTemplate = actionTemplate.replace(ACTION_NAME, action.getType());
if (action.isPassed()) {
if (action.getType().equals("ActionCallscript")) {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-action-call-script");
} else {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-pass");
}
actionTemplate = actionTemplate.replace(ACTION_NAME_STYLE, "action-name-pass");
actionTemplate = actionTemplate.replace(RESULT_ICON, "result-pass-icon");
} else if (!action.isPassed() && !action.isStop()) {
if (action.getType().equals("ActionCallscript")) {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-action-call-script error " + testInfo.getTestName());
} else {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-fail-pass error " + testInfo.getTestName());
}
actionTemplate = actionTemplate.replace(ACTION_NAME_STYLE, "action-name-fail-pass");
actionTemplate = actionTemplate.replace(RESULT_ICON, "result-fail-pass-icon");
} else if (!action.isPassed() && action.isStop()) {
if (action.getType().equals("ActionCallscript")) {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-action-call-script error " + testInfo.getTestName());
} else {
actionTemplate = actionTemplate.replace(STATUS_LINE_STYLE, "status-line-failed error " + testInfo.getTestName());
}
actionTemplate = actionTemplate.replace(ACTION_NAME_STYLE, "action-name-failed");
actionTemplate = actionTemplate.replace(RESULT_ICON, "result-failed-icon");
}
actionTemplate = actionTemplate.replace(ACTION_ID, action.getScript());
}
switch (action.getType()) {
case "ActionChannelStart": {
if (action.getAppDataJson() == null || action.getAppDataJson().getAppVersion() == null) {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionChannel(twoElementDataTemplate, action, ActionChannelOperation.CHANNEL_START, appIcons).getResult());
} else {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionChannel(threeElementDataTemplate, action, ActionChannelOperation.CHANNEL_START, appIcons).getResult());
}
break;
}
case "ActionChannelClose": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionChannel(twoElementDataTemplate, action, ActionChannelOperation.CHANNEL_CLOSE, appIcons).getResult());
break;
}
case "ActionChannelSwitch": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionChannel(oneElementDataTemplate, action, ActionChannelOperation.CHANNEL_CHANGE, appIcons).getResult());
break;
}
case "ActionGotoUrl": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionGoToUrl(oneElementDataTemplate, action).getResult());
break;
}
case "ActionMouseDragDrop":
case "ActionMouseSwipe":
case "ActionMouse":
case "ActionMouseKey": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionMouse(twoElementDataTemplate, action, false).getResult());
break;
}
case "ActionMouseScroll": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionMouse(twoElementDataTemplate, action, true).getResult());
break;
}
case "ActionWindowState": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionWindowState(oneElementDataTemplate, action, false).getResult());
break;
}
case "ActionWindowResize": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionWindowState(twoElementDataTemplate, action, true).getResult());
break;
}
case "ActionText": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionTextAndSelect(twoElementDataTemplate, action, false).getResult());
break;
}
case "ActionSelect": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionTextAndSelect(twoElementDataTemplate, action, true).getResult());
break;
}
case "ActionProperty": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionProperty(twoElementDataTemplate, action).getResult());
break;
}
case "ActionScripting": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionScripting(twoElementDataTemplate, action).getResult());
break;
}
case "ActionWindowSwitch": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionWindowSwitch(oneElementDataTemplate, action).getResult());
break;
}
case "ActionAssertValue": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionAssert(actionAssertValueTemplate, action, ActionAssertOperation.ASSER_VALUE).getResult());
break;
}
case "ActionAssertProperty": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionAssert(actionAssertPropertyTemplate, action, ActionAssertOperation.ASSERT_PROPERTY).getResult());
break;
}
case "ActionAssertCount": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionAssert(actionAssertOccurrenceTemplate, action, ActionAssertOperation.ASSERT_COUNT).getResult());
break;
}
case "ActionComment": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionComment(oneElementDataTemplate, action).getResult());
break;
}
case "ActionApi": {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionApi(fiveElementDataTemplate, action).getResult());
break;
}
case "ActionCallscript": {
if (action.getAppDataJson() != null && !action.getAppDataJson().getData().isEmpty() || action.getActionElement() != null) {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionCallScript(twoElementDataTemplate, action, true, testInfo.getProject()).getResult());
} else {
actionTemplate = actionTemplate.replace(ACTION_BODY, new HtmlReportActionCallScript(oneElementDataTemplate, action, false, testInfo.getProject()).getResult());
}
break;
}
case "StartScriptAction": {
actionTemplate = "";
break;
}
default: {
AtsLogger.printLog("default action");
}
}
if (!action.getType().equals("ActionComment")) {
if (reportLevel == 2) {
actionTemplate = actionTemplate.replace("data-body-multiple-cell-cell-with-right-header", "data-body-multiple-cell-cell-with-right-header data-body-image-less-cell");
} else if (reportLevel == 1) {
actionTemplate = actionTemplate.replace("class='data'", "style='display: none;'");
actionTemplate = actionTemplate.replace("class='inner-container'", "class='inner-container' style='min-height: unset;'");
actionTemplate = actionTemplate.replace("class='header'", "class='header' style='padding-bottom: unset;'");
}
}
return actionTemplate;
}
private String buildMediaData(Image image) {
if (image.getType().equals("video")) {
this.actionTemplate = actionTemplate.replace("${mediaIcon}", "data-video-icon");
this.actionTemplate = actionTemplate.replace("${mediaHostIcon}", "data-video-host-icon");
return MEDIA_DATA_HTML_TEMPLATE.replace("${mediaTag}", "video")
.replace("${contentType}", "video")
.replace("${mediaType}", "mp4")
.replace("${mediaSrc}", image.getSrc())
.replace("${controls}", "controls='controls'");
} else {
this.actionTemplate = actionTemplate.replace("${mediaHostIcon}", "data-image-host-icon");
this.actionTemplate = actionTemplate.replace("${mediaIcon}", "data-image-icon");
return MEDIA_DATA_HTML_TEMPLATE.replace("${mediaTag}", "img").replace("${contentType}", "image").replace("${mediaType}", image.getType()).replace("${mediaSrc}", image.getSrc());
}
}
private CharSequence buildScriptName(Action action, TestInfo testInfo) {
return TEST_SCRIPT_HTML_TEMPLATE.replace("${scriptNameUri}", getActionUrl(action, testInfo)).replace("${scriptName}", action.getScript() + " ").replace("${scriptNameTitle}", action.getScript());
}
public void setActionTemplate(String actionTemplate) {
this.actionTemplate = actionTemplate;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy