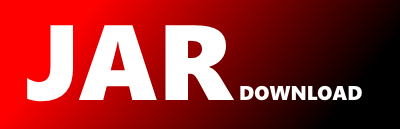
com.ats.tools.report.actions.HtmlReportActionCallScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.actions;
import com.ats.tools.report.models.Action;
import com.ats.tools.report.models.Project;
import com.ats.tools.report.models.TemplateConstants;
import java.util.*;
import java.util.stream.Collectors;
public class HtmlReportActionCallScript {
private static final String ACTION_CALL_SCRIPT_HEADER = TemplateConstants.HEADER_ONE;
private static final String ACTION_CALL_SCRIPT = TemplateConstants.VALUE_ONE;
private static final String ACTION_CALL_SCRIPT_PARAMETERS_HEADER = TemplateConstants.HEADER_TWO;
private static final String ACTION_CALL_SCRIPT_PARAMETERS_VALUE = TemplateConstants.VALUE_TWO;
public static final String ATS_CALL_SCRIPT_HTML_TEMPLATE = "${scriptName}";
private String template;
private String result;
public HtmlReportActionCallScript(String template, Action action, boolean hasParameters, Project project) {
this.template = template;
this.result = template.replace(ACTION_CALL_SCRIPT_HEADER, "SubScript called");
this.result = result.replace(ACTION_CALL_SCRIPT, buildScriptUrl(action, project));
if (hasParameters) {
if (action.getActionElement() == null) {
this.result = result.replace(ACTION_CALL_SCRIPT_PARAMETERS_HEADER, "Parameters");
HashMap assetsMap = new HashMap<>();
Set> entries = action.getAppDataJson().getData().entrySet().stream().filter(stringStringEntry -> !stringStringEntry.getValue().equals("Ø")).collect(Collectors.toSet());
entries.stream().peek(stringStringEntry -> {
if (stringStringEntry.getKey().equals("asset")) {
String asset = stringStringEntry.getValue().replace("assets:///", "");
String assetWithUri = ATS_CALL_SCRIPT_HTML_TEMPLATE.replace("${scriptNameUri}", project.getProjectId() + "/asset/" + asset).replace("${scriptName}", asset);
stringStringEntry.setValue(assetWithUri);
}
}).forEach(stringStringEntry -> assetsMap.put(stringStringEntry.getKey(), stringStringEntry.getValue()));
if (assetsMap.containsKey("asset")) {
this.result = result.replace(ACTION_CALL_SCRIPT_PARAMETERS_VALUE, String.join("", assetsMap.values()));
} else {
this.result = result.replace(ACTION_CALL_SCRIPT_PARAMETERS_VALUE, HtmlReportActionKeyValueTemplate.buildEntireKeyValueString(assetsMap.entrySet().stream()
.sorted(Comparator.comparing(o -> Integer.valueOf(o.getKey())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue, (oldValue, newValue) -> oldValue, LinkedHashMap::new)), true));
}
} else {
this.result = result.replace(ACTION_CALL_SCRIPT_PARAMETERS_HEADER, "Parameters");
this.result = this.result.replace(ACTION_CALL_SCRIPT_PARAMETERS_VALUE, action.getActionElement().getCriterias());
}
}
}
private CharSequence buildScriptUrl(Action action, Project project) {
return ATS_CALL_SCRIPT_HTML_TEMPLATE.replace("${scriptNameUri}", project.getProjectId() + "/script/" + action.getValue()).replace("${scriptName}", action.getValue());
}
public void setTemplate(String template) {
this.template = template;
}
public String getResult() {
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy