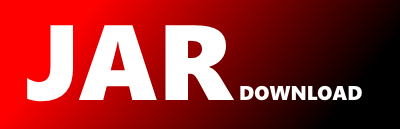
com.ats.tools.report.actions.HtmlReportActionChannel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.actions;
import com.ats.tools.report.models.Action;
import com.ats.tools.report.models.OperatorsWithIcons;
import com.ats.tools.report.models.TemplateConstants;
import org.apache.commons.lang3.StringUtils;
import java.util.Map;
public class HtmlReportActionChannel {
private static final String CHANNEL_NAME_HEADER = TemplateConstants.HEADER_ONE;
private static final String CHANNEL_NAME = TemplateConstants.VALUE_ONE;
private static final String APPLICATION_HEADER = TemplateConstants.HEADER_TWO;
private static final String APPLICATION = TemplateConstants.VALUE_TWO;
private static final String APPLICATION_VERSION_HEADER = TemplateConstants.HEADER_THREE;
private static final String APPLICATION_VERSION = TemplateConstants.VALUE_THREE;
private static final String APPLICATION_ICON_TEMPLATE = "
";
private static final String SWITCH_CHANNEL_HTML_TEMPLATE = "${oldChannel}${operator}${newChannel}";
public static enum ActionChannelOperation {
CHANNEL_START, CHANNEL_CLOSE, CHANNEL_CHANGE
}
private static final String APP_ICON = "${appIcon}";
private static final String APP_NAME = "${appName}";
private String template;
private String result;
public HtmlReportActionChannel(String template, Action action, ActionChannelOperation actionChannelOperation, Map appIcons) {
this.template = template;
if (actionChannelOperation.equals(ActionChannelOperation.CHANNEL_CHANGE)) {
this.result = template.replace(CHANNEL_NAME_HEADER, "Switch channel");
if (action.getChannel() != null) {
this.result = result.replace(CHANNEL_NAME, buildSwitchChannelString(action));
} else {
this.result = result.replace(CHANNEL_NAME, "");
}
} else {
if (action.getAppDataJson() != null && action.getChannel() != null) {
String applicationName = action.getAppDataJson().getApp();
String appIcon = appIcons.get(action.getAppDataJson().getApp());
if (StringUtils.isNoneBlank(appIcon)) {
applicationName = APPLICATION_ICON_TEMPLATE.replace(APP_ICON, appIcon) + action.getAppDataJson().getApp();
}
this.result = template.replace(CHANNEL_NAME_HEADER, "Channel name");
this.result = result.replace(CHANNEL_NAME, action.getAppDataJson().getName());
this.result = result.replace(APPLICATION_HEADER, "Application");
this.result = result.replace(APPLICATION, applicationName);
if (actionChannelOperation.equals(ActionChannelOperation.CHANNEL_START)) {
this.result = result.replace(APPLICATION_VERSION_HEADER, "Version");
if (action.getAppDataJson().getAppVersion() != null) {
this.result = result.replace(APPLICATION_VERSION, action.getAppDataJson().getAppVersion());
}
}
} else {
this.result = template.replace(CHANNEL_NAME_HEADER, "Channel name");
this.result = result.replace(CHANNEL_NAME, "");
this.result = result.replace(APPLICATION_HEADER, "Application");
this.result = result.replace(APPLICATION, "");
if (actionChannelOperation.equals(ActionChannelOperation.CHANNEL_START)) {
this.result = result.replace(APPLICATION_VERSION_HEADER, "Version");
this.result = result.replace(APPLICATION_VERSION, "");
}
}
}
}
private CharSequence buildSwitchChannelString(Action action) {
return SWITCH_CHANNEL_HTML_TEMPLATE.replace("${oldChannel}", action.getChannel().getName())
.replace("${newChannel}", action.getValue())
.replace("${operator}", OperatorsWithIcons.getIconForOperator("ARROW"));
}
public void setTemplate(String template) {
this.template = template;
}
public String getResult() {
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy