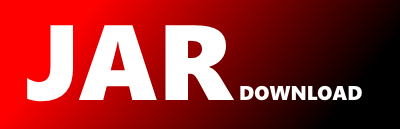
com.ats.tools.report.analytics.ActionsDuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ats-automated-testing Show documentation
Show all versions of ats-automated-testing Show documentation
Code generator library to create and execute GUI automated tests
The newest version!
package com.ats.tools.report.analytics;
import java.awt.BasicStroke;
import java.awt.Color;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.Base64;
import java.util.List;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.ChartUtils;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.axis.NumberAxis;
import org.jfree.chart.axis.NumberTickUnit;
import org.jfree.chart.plot.CategoryPlot;
import org.jfree.chart.plot.PlotOrientation;
import org.jfree.data.category.DefaultCategoryDataset;
public class ActionsDuration {
private static final Color LINE_COLOR = Color.decode("#699400");
private static final Color GRID_COLOR = Color.decode("#5a6886");
private DefaultCategoryDataset dataSet;
private int index = 0;
private int total = 0;
public ActionsDuration() {
dataSet = new DefaultCategoryDataset();
}
public ActionsDuration(List data) {
dataSet = new DefaultCategoryDataset();
for (int l : data) {
addDuration(l);
}
}
public void addDuration(int value) {
total += value;
dataSet.addValue(value, "duration" , String.valueOf(index));
index++;
}
public int getTotal() {
return total;
}
public String getBase64Chart() {
JFreeChart chart = ChartFactory.createLineChart(
null,
null,
null,
dataSet,PlotOrientation.VERTICAL,
true,true,false);
chart.removeLegend();
CategoryPlot plot = (CategoryPlot) chart.getPlot();
plot.setFixedLegendItems(null);
chart.setBackgroundPaint(new Color(0xFF, 0xFF, 0xFF, 0x00));
plot.setBackgroundAlpha(0.0F);
plot.getDomainAxis().setVisible(false);
plot.getDomainAxis().setTickLabelsVisible(false);
plot.getRangeAxis().setVisible(false);
plot.getRangeAxis().setTickLabelsVisible(false);
plot.setOutlinePaint(null);
plot.setFixedLegendItems(null);
float[] dash1 = { 2f, 0f, 3f };
plot.setRangeGridlineStroke(new BasicStroke(0.3F, BasicStroke.CAP_BUTT, BasicStroke.JOIN_ROUND, 1.0f, dash1, 2f));
plot.setRangeGridlinePaint(GRID_COLOR);
plot.getRenderer().setSeriesStroke(0, new BasicStroke(3.0f));
plot.getRenderer().setSeriesPaint(0, LINE_COLOR);
NumberAxis yAxis = (NumberAxis) plot.getRangeAxis();
yAxis.setTickUnit(new NumberTickUnit(500));
final ChartPanel chartPanel = new ChartPanel(chart);
chartPanel.setPreferredSize(new java.awt.Dimension(260, 80));
ByteArrayOutputStream baos = new ByteArrayOutputStream();
try {
ChartUtils.writeChartAsPNG(baos, chart, 260, 80);
} catch (IOException e) {
return null;
}
return Base64.getEncoder().encodeToString(baos.toByteArray());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy